What Does Chr Do In Python
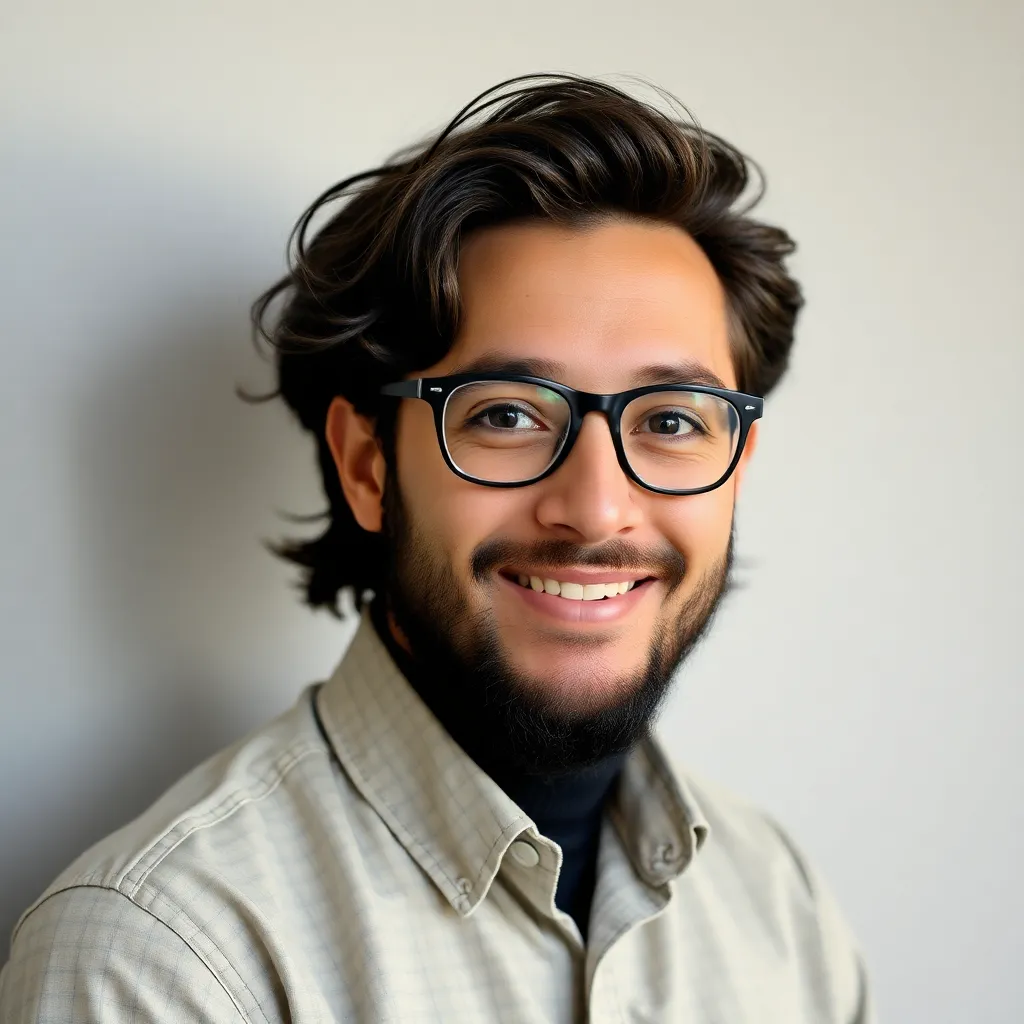
News Leon
Mar 28, 2025 · 5 min read
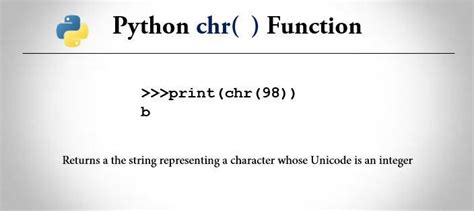
Table of Contents
What Does chr()
Do in Python? A Deep Dive into Character Representation
Python's built-in function chr()
is a fundamental tool for working with characters and their corresponding numerical representations. Understanding its functionality is crucial for programmers dealing with text processing, encoding, cryptography, and more. This comprehensive guide delves into the intricacies of chr()
, exploring its usage, practical applications, and potential pitfalls.
Understanding the ASCII and Unicode Systems
Before diving into chr()
, it's essential to grasp the underlying principles of character encoding. Computers fundamentally store information as numbers. To represent characters (letters, numbers, symbols), encoding systems map numerical values to characters.
ASCII: The Legacy Encoding
ASCII (American Standard Code for Information Interchange) is a historical encoding standard that uses 7 bits to represent 128 characters. This includes uppercase and lowercase English letters, numbers, punctuation marks, and control characters. While simple, ASCII's limitations are apparent – it doesn't support characters from other languages.
Unicode: A Universal Solution
Unicode addresses ASCII's shortcomings by providing a universal character set encompassing characters from virtually every language. It employs multiple encoding forms, the most common being UTF-8 and UTF-16. UTF-8 is a variable-length encoding, meaning characters can be represented using 1 to 4 bytes, making it efficient for storing text containing a mix of characters from different languages. UTF-16 uses 2 or 4 bytes per character.
The Role of chr()
in Character Representation
The chr()
function in Python acts as a bridge between the numerical representation of a character and its actual character form. It takes an integer (an ASCII or Unicode code point) as input and returns the corresponding character.
Syntax:
chr(i)
where i
is an integer representing the Unicode code point.
Example:
# Get the character corresponding to Unicode code point 65
character = chr(65)
print(character) # Output: A
# Get the character corresponding to Unicode code point 97
character = chr(97)
print(character) # Output: a
# Get a special character
special_char = chr(945) # Alpha
print(special_char)
#Example of a character outside the basic multilingual plane (BMP)
supplementary_char = chr(0x1F600) #Grinning Face
print(supplementary_char)
These examples demonstrate how chr()
converts integer code points to their character equivalents. Note that the input integer must be within the valid range of Unicode code points (0 to 1,114,111). Attempting to use a value outside this range will raise a ValueError
.
chr()
and its Relationship with ord()
Python's ord()
function performs the inverse operation of chr()
. It takes a character as input and returns its corresponding Unicode code point (an integer).
Example:
# Get the Unicode code point of the character 'A'
code_point = ord('A')
print(code_point) # Output: 65
# Get the Unicode code point of a special character
code_point_alpha = ord('α')
print(code_point_alpha) # Output: 945
#Demonstrating the relationship
char = 'A'
code = ord(char)
char_back = chr(code)
print(f"Original character: {char}, Codepoint: {code}, Character after conversion: {char_back}")
The combination of chr()
and ord()
allows for seamless conversion between characters and their numerical representations, opening up possibilities for various programming tasks.
Practical Applications of chr()
chr()
finds its application in numerous areas of programming:
1. Generating Character Sequences
chr()
simplifies the generation of character sequences. For instance, creating a string containing all uppercase letters:
uppercase_letters = "".join([chr(i) for i in range(65, 91)])
print(uppercase_letters) # Output: ABCDEFGHIJKLMNOPQRSTUVWXYZ
2. Handling Special Characters
Many programming tasks involve dealing with special characters such as accented letters, symbols, or control characters. chr()
facilitates their manipulation:
# Print a copyright symbol
copyright_symbol = chr(169)
print(copyright_symbol) # Output: ©
3. Encoding and Decoding
In scenarios requiring encoding and decoding of data, chr()
plays a crucial role. It helps translate numerical representations into readable characters.
4. Working with Files and Databases
When interacting with files or databases that store data in encoded formats, chr()
is instrumental in converting numerical representations back into characters for proper interpretation.
5. Cryptography
Basic cryptographic techniques might utilize chr()
to transform numerical keys into characters or vice-versa. This forms part of encryption/decryption processes.
Error Handling and Best Practices
Handling ValueError
As mentioned earlier, providing an integer outside the valid Unicode range (0 to 1,114,111) to chr()
results in a ValueError
. Robust code should incorporate error handling to gracefully manage such situations:
try:
invalid_char = chr(1200000) #Outside Valid Range
except ValueError:
print("Invalid Unicode code point.")
Choosing the Correct Encoding
When working with text files or data from external sources, it is crucial to specify the correct encoding (e.g., UTF-8, UTF-16, Latin-1). Incorrect encoding can lead to character representation errors.
Performance Considerations
For generating extensive character sequences, consider using optimized methods instead of repeatedly calling chr()
in a loop. String manipulation techniques can be significantly more efficient for large-scale operations.
Advanced Applications and Further Exploration
The applications of chr()
extend far beyond basic character conversion. Its role in advanced programming scenarios includes:
- Internationalization and Localization:
chr()
is fundamental for handling multilingual text and adapting applications for different locales. - Regular Expressions: When working with regular expressions that involve specific character ranges,
chr()
simplifies specifying character sets. - Custom Encoding Schemes: For specialized encoding or decoding needs,
chr()
can be combined with other techniques to create custom solutions.
Conclusion
chr()
is a seemingly simple yet powerful Python function with wide-ranging applications in various programming domains. Understanding its mechanics, limitations, and integration with ord()
empowers programmers to handle character representation effectively, building robust and versatile applications. By following best practices and incorporating appropriate error handling, developers can leverage chr()
to its full potential, leading to efficient and reliable code. The comprehensive understanding of character encoding, coupled with the skillful use of chr()
, is crucial for building applications capable of handling text data from diverse sources and languages effectively. Remember to always consider the context of your application and choose the appropriate encoding to ensure the accurate representation and manipulation of characters.
Latest Posts
Latest Posts
-
Classify The Following As A Homogeneous Or A Heterogeneous Mixture
Mar 31, 2025
-
What Physical Quantity Does The Slope Represent
Mar 31, 2025
-
An Object Becomes Positively Charged By Gaining Protons
Mar 31, 2025
-
Right Hand Rule For Angular Velocity
Mar 31, 2025
-
Read The Extract And Answer The Following Questions
Mar 31, 2025
Related Post
Thank you for visiting our website which covers about What Does Chr Do In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.