Increment And Decrement Operators In C++
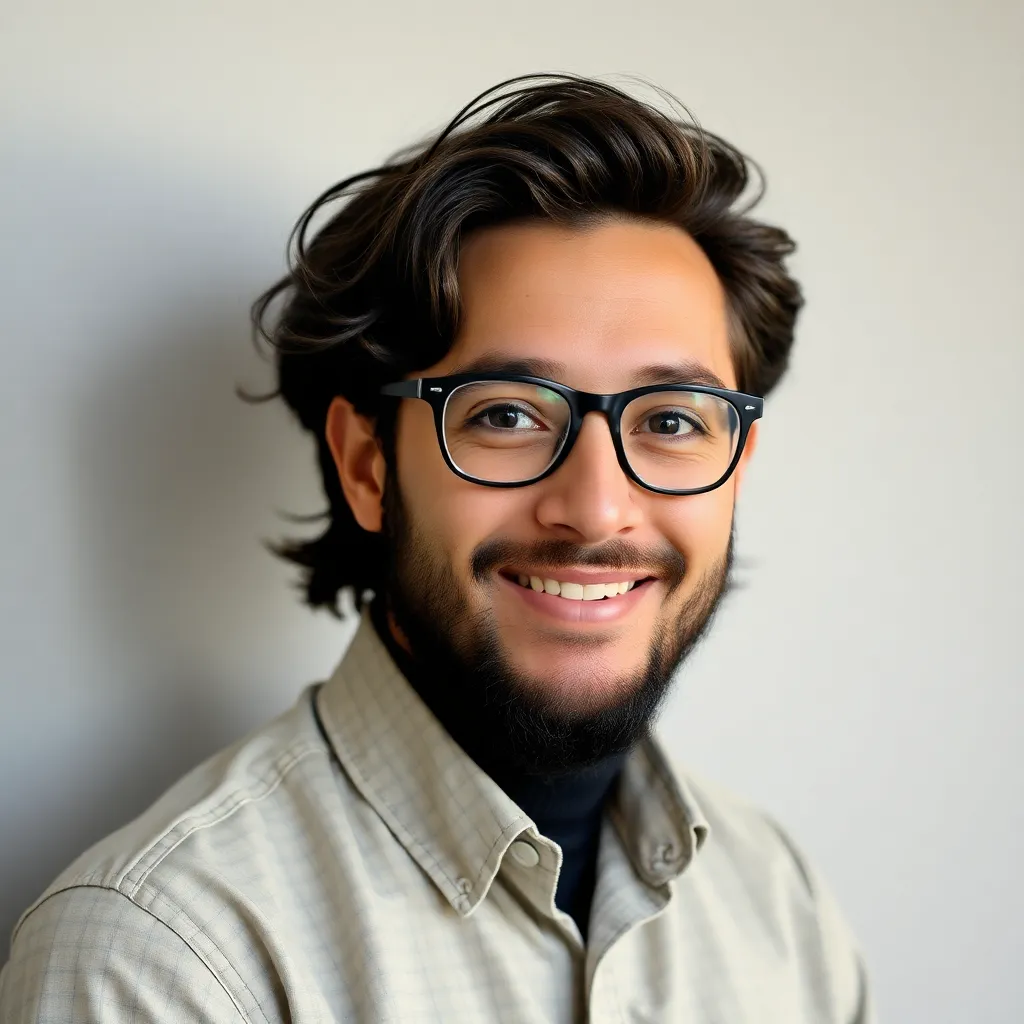
News Leon
Mar 15, 2025 · 5 min read
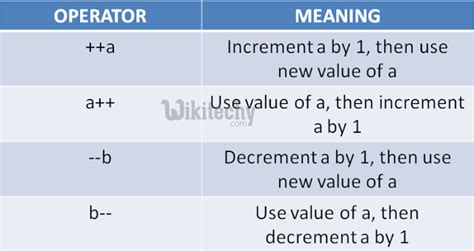
Table of Contents
Increment and Decrement Operators in C++: A Deep Dive
Increment and decrement operators are fundamental components of C++ programming, offering concise ways to modify numerical values. Understanding their nuances, especially the subtle differences between prefix and postfix variations, is crucial for writing efficient and error-free code. This comprehensive guide will explore these operators in detail, covering their syntax, functionality, behavior in various contexts, and best practices for their usage.
Understanding the Basics: ++ and --
The increment operator (++
) increases the value of a variable by 1, while the decrement operator (--
) decreases the value by 1. Both operators work on integer types (like int
, short
, long
, etc.) and floating-point types (like float
, double
). However, their behavior is most often discussed and relevant with integer types. Their power lies not just in their simplicity, but in their flexibility, thanks to their prefix and postfix forms.
Prefix vs. Postfix: A Key Distinction
The crucial difference between increment and decrement operators lies in their placement relative to the operand (the variable being modified):
-
Prefix: The operator is placed before the operand (
++x
or--x
). In this form, the increment or decrement operation happens before the value is used in the expression. -
Postfix: The operator is placed after the operand (
x++
orx--
). In this form, the increment or decrement operation happens after the value is used in the expression.
Let's illustrate with an example:
#include
int main() {
int x = 5;
int y;
y = ++x; // Prefix increment
std::cout << "x (prefix): " << x << ", y: " << y << std::endl; // Output: x (prefix): 6, y: 6
x = 5; // Reset x
y = x++; // Postfix increment
std::cout << "x (postfix): " << x << ", y: " << y << std::endl; // Output: x (postfix): 6, y: 5
return 0;
}
In the prefix case, x
is incremented before its value is assigned to y
. In the postfix case, the original value of x
(5) is assigned to y
, and then x
is incremented.
This seemingly small difference can significantly impact the results, especially within complex expressions. Understanding this difference is paramount to avoiding subtle bugs.
Increment and Decrement in Expressions
The placement of the increment/decrement operator significantly alters the outcome when used within larger expressions. Consider this:
#include
int main() {
int a = 10;
int b = 20;
int c;
c = a++ + b; //Postfix increment of a
std::cout << "a: " << a << ", b: " << b << ", c: " << c << std::endl; // Output: a: 11, b: 20, c: 30
a = 10; //Reset a
b = 20; //Reset b
c = ++a + b; //Prefix increment of a
std::cout << "a: " << a << ", b: " << b << ", c: " << c << std::endl; // Output: a: 11, b: 20, c: 31
return 0;
}
Notice how the order of operations determines the final value of c
. In the first instance, a
is added to b
before it is incremented. In the second, a
is incremented before the addition takes place. This highlights the importance of careful consideration when embedding these operators within more complex calculations.
Increment/Decrement and Loops
Increment and decrement operators are extensively used in loops, providing an elegant way to control iteration. The for
loop is a prime example:
#include
int main() {
for (int i = 0; i < 10; ++i) {
std::cout << i << " ";
}
std::cout << std::endl; // Output: 0 1 2 3 4 5 6 7 8 9
return 0;
}
Here, the ++i
(prefix increment) efficiently increases the loop counter i
after each iteration. While the postfix version (i++
) would also function correctly in this example, prefix increment is generally preferred for its slight performance advantage in this specific context. The compiler can often optimize this difference away, however, so it's not always a significant concern.
Avoiding Common Pitfalls
While powerful, increment and decrement operators can lead to errors if not handled carefully:
-
Unintended Side Effects: Using them within complex expressions without fully understanding the order of operations can lead to unexpected results. Always break down complex expressions to improve readability and reduce the chance of mistakes.
-
Overuse: While concise, overuse can reduce code readability. If the increment/decrement operation isn't immediately obvious, it might be better to use a more explicit assignment statement (
x = x + 1;
orx = x - 1;
). -
Mixing Prefix and Postfix: Avoid needlessly mixing prefix and postfix forms within the same expression, as this significantly increases the cognitive load required to understand the code. Consistency greatly improves maintainability.
-
Modifying the same variable multiple times within an expression: This can lead to unpredictable outcomes. Ensure that each increment/decrement operation clearly affects a specific point in the expression.
Best Practices
To avoid issues and ensure clean, maintainable code, follow these best practices:
-
Prioritize Readability: Choose the operator placement (prefix or postfix) that enhances readability, even if it means slightly sacrificing potential performance optimization.
-
Use Consistently: Maintain consistency in your use of prefix or postfix within a given code segment.
-
Simplify Expressions: If an expression is becoming too complex, break it down into smaller, more manageable parts.
-
Comment Clearly: If your use of increment/decrement operators is not self-evident, add a comment explaining the intention.
Advanced Scenarios and Considerations
While the basics are straightforward, certain scenarios require extra attention:
-
Pointers and Increment/Decrement: When applied to pointers, these operators advance the pointer to the next memory location. Understanding pointer arithmetic is essential here. Incrementing a pointer moves it forward by the size of the data type it points to.
-
Overloaded Operators: In advanced C++, you can overload the increment and decrement operators to define custom behavior for user-defined types (classes and structs).
-
Compiler Optimizations: Modern compilers often optimize increment/decrement operations, sometimes making the difference between prefix and postfix negligible. However, relying on compiler optimizations isn't a substitute for understanding the fundamental behavior.
Conclusion
Increment and decrement operators are fundamental tools in C++. Mastering their nuances, particularly the distinction between prefix and postfix forms, is key to writing efficient, reliable, and maintainable code. By adhering to best practices and carefully considering their use within complex expressions, you can harness their power effectively and avoid potential pitfalls. Always prioritize readability and avoid overly clever or obscure usage that hinders code understanding and maintainability. Understanding the underlying mechanics allows you to choose the most appropriate approach in each scenario, maximizing the effectiveness of these essential operators.
Latest Posts
Latest Posts
-
Concave Mirror And Convex Mirror Difference
Mar 15, 2025
-
Which Is Not A Cranial Bone Of The Skull
Mar 15, 2025
-
Mountain Range That Separates Europe And Asia
Mar 15, 2025
-
16 Out Of 40 As A Percentage
Mar 15, 2025
-
Which Of The Following Is A True Solution
Mar 15, 2025
Related Post
Thank you for visiting our website which covers about Increment And Decrement Operators In C++ . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.