How To Use Insert In Python
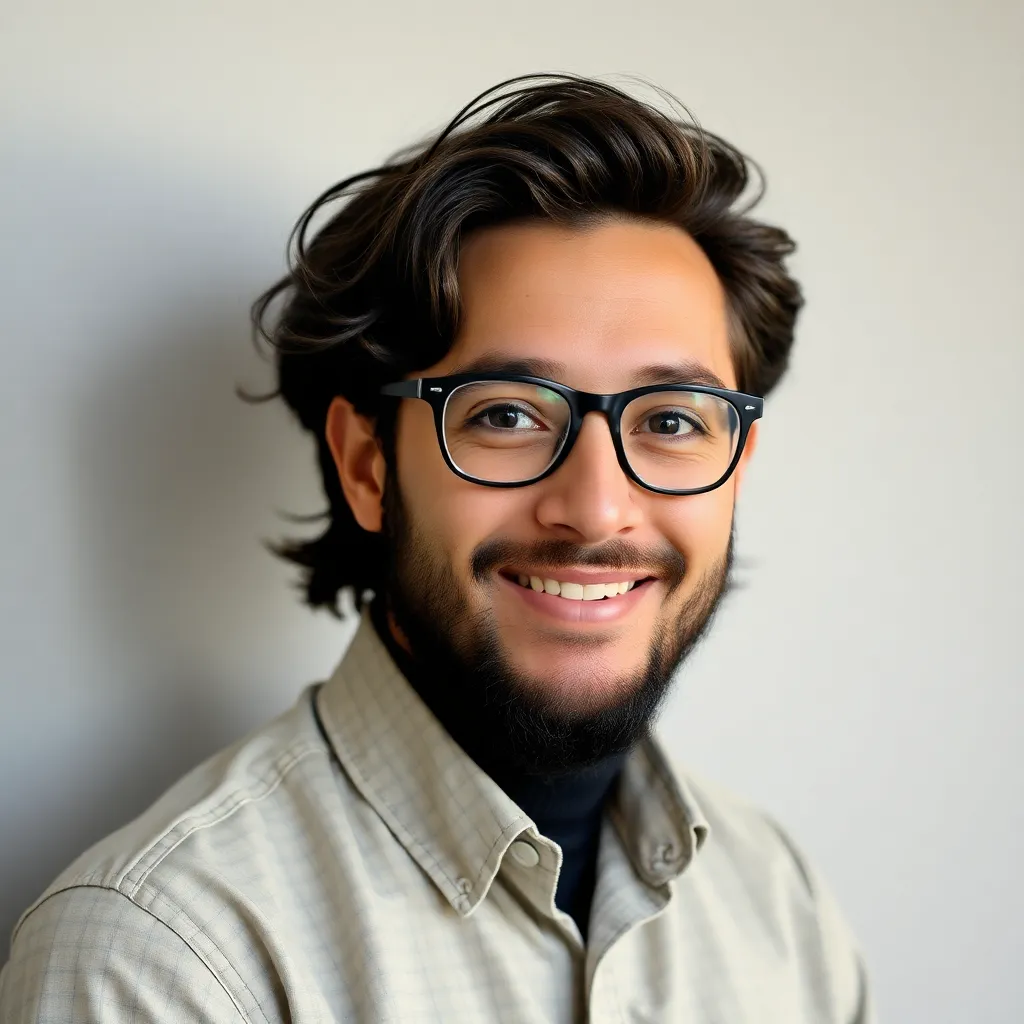
News Leon
Mar 31, 2025 · 5 min read
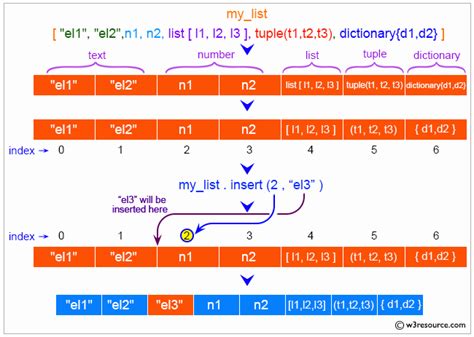
Table of Contents
Mastering the Art of insert()
in Python: A Comprehensive Guide
Python's built-in insert()
method provides a powerful way to manipulate lists and other sequence-like objects. Understanding its nuances is crucial for efficient and elegant Python programming. This comprehensive guide will delve into the intricacies of insert()
, exploring its usage, showcasing practical examples, and addressing common pitfalls. We'll cover everything from basic insertion to more advanced techniques, making you a confident insert()
master.
Understanding the insert()
Method
The insert()
method is a powerful tool for modifying lists in Python. Unlike the append()
method, which adds an element to the end of a list, insert()
allows you to add an element at a specific index within the list. This precise control is essential for various data manipulation tasks.
The syntax is straightforward:
list_name.insert(index, element)
list_name
: The list you want to modify.index
: The numerical index where you want to insert theelement
. Remember that Python uses zero-based indexing, meaning the first element is at index 0, the second at index 1, and so on.element
: The item you wish to insert into the list. This can be any data type—numbers, strings, other lists, objects, etc.
Important Note: If the index
you provide is greater than or equal to the length of the list, the element
is appended to the end of the list. If the index
is negative, it's counted from the end of the list (similar to negative indexing in list slicing).
Basic insert()
Examples
Let's start with some fundamental examples to solidify your understanding:
my_list = [10, 20, 30, 40]
# Insert 15 at index 2
my_list.insert(2, 15)
print(my_list) # Output: [10, 20, 15, 30, 40]
# Insert "hello" at the beginning (index 0)
my_list.insert(0, "hello")
print(my_list) # Output: ['hello', 10, 20, 15, 30, 40]
# Insert 50 at the end (index equal to list length)
my_list.insert(len(my_list), 50)
print(my_list) # Output: ['hello', 10, 20, 15, 30, 40, 50]
# Insert using a negative index
my_list.insert(-1, "world")
print(my_list) # Output: ['hello', 10, 20, 15, 30, 40, 'world', 50]
These examples demonstrate the flexibility of insert()
. You can add elements anywhere within the list, including at the beginning, end, or at a specific position in between.
Advanced insert()
Techniques
Now let's explore more advanced scenarios where insert()
shines:
Inserting Multiple Elements
While insert()
adds only one element at a time, we can cleverly use it in conjunction with list slicing and other techniques to insert multiple elements. Consider this example:
my_list = [1, 2, 3, 4, 5]
new_elements = [6, 7, 8]
# Insert multiple elements at index 2
my_list[2:2] = new_elements #Slicing inserts the list at the specified index
print(my_list) # Output: [1, 2, 6, 7, 8, 3, 4, 5]
my_list = [1,2,3,4,5]
my_list.insert(2,[6,7,8]) #This will insert a list inside the list
print(my_list) #Output: [1, 2, [6, 7, 8], 3, 4, 5]
This technique efficiently handles the insertion of multiple elements at once without needing multiple insert()
calls.
Inserting based on conditions
Often, you'll want to insert elements conditionally. This is where the power of Python's control flow statements comes into play:
my_list = [1, 3, 5, 7, 9]
for i, num in enumerate(my_list):
if num % 2 != 0: #Check for odd numbers
my_list.insert(i, num * 2) #Insert the double of the odd number before it
print(my_list) #Output: [2, 1, 6, 3, 10, 5, 14, 7, 18, 9]
This code iterates through the list, checks for odd numbers, and conditionally inserts their doubles before the original odd number. Notice how this can modify the indices as you go. It's crucial to consider index adjustments when modifying a list while iterating.
insert()
vs. append()
vs. extend()
It's essential to understand the differences between insert()
, append()
, and extend()
to choose the most appropriate method for your task:
Method | Description | Example |
---|---|---|
append() |
Adds an element to the end of the list. | my_list.append(10) |
insert() |
Inserts an element at a specific index. | my_list.insert(2, 10) |
extend() |
Extends the list by appending elements from an iterable (e.g., another list). | my_list.extend([10, 20]) |
Choosing the right method depends on your needs. If you need to add an element to the end, append()
is efficient. For precise placement, use insert()
. If you need to add multiple elements from another iterable, extend()
is the best choice.
Error Handling and Best Practices
Index Errors
The most common error encountered with insert()
is IndexError
. This occurs when you try to insert an element at an index that's out of bounds for the list. Always double-check your indices to avoid this.
my_list = [1, 2, 3]
try:
my_list.insert(10, 5) # IndexError: list assignment index out of range
except IndexError as e:
print(f"An error occurred: {e}")
Efficiency Considerations
While insert()
is flexible, inserting elements at the beginning of a large list can be inefficient because it requires shifting all subsequent elements. For performance-critical applications, consider using alternative data structures like collections.deque
which are optimized for insertions at both ends.
Readability and Maintainability
Write clear and concise code using insert()
. Use meaningful variable names, add comments where necessary, and keep your code well-structured.
Real-World Applications of insert()
The insert()
method is incredibly versatile and finds application in numerous scenarios:
- Data Processing: Inserting data into specific locations within a list based on criteria or ordering.
- Queue Management:
insert()
can be used to simulate queue operations with proper index management. - Game Development: Inserting new objects or players into game worlds at specific coordinates.
- Text Processing: Inserting characters or words into strings (converted to lists).
- Algorithm Implementation: Many algorithms utilize
insert()
for tasks such as sorting and searching.
Conclusion
Python's insert()
method provides a powerful mechanism for manipulating lists and other sequences. By understanding its syntax, capabilities, and potential pitfalls, you can leverage its full potential in your Python projects. From basic insertions to advanced techniques like conditional insertion and efficient handling of multiple elements, insert()
is a valuable tool in a Python programmer's arsenal. Remember to choose the most efficient and appropriate method for each scenario, always prioritizing code readability and maintainability. Mastering insert()
will significantly enhance your Python programming skills and allow you to create more robust and efficient applications.
Latest Posts
Latest Posts
-
All Of The Following Characteristics Are Associated With Epithelium Except
Apr 02, 2025
-
This Organelle Pumps Out Excess Water
Apr 02, 2025
-
If The Demand For A Good Is Elastic Then
Apr 02, 2025
-
Solid Liquid Or Gas That A Wave Travels Through
Apr 02, 2025
-
What Is The Antiderivative Of E 2x
Apr 02, 2025
Related Post
Thank you for visiting our website which covers about How To Use Insert In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.