How To Use End In Python
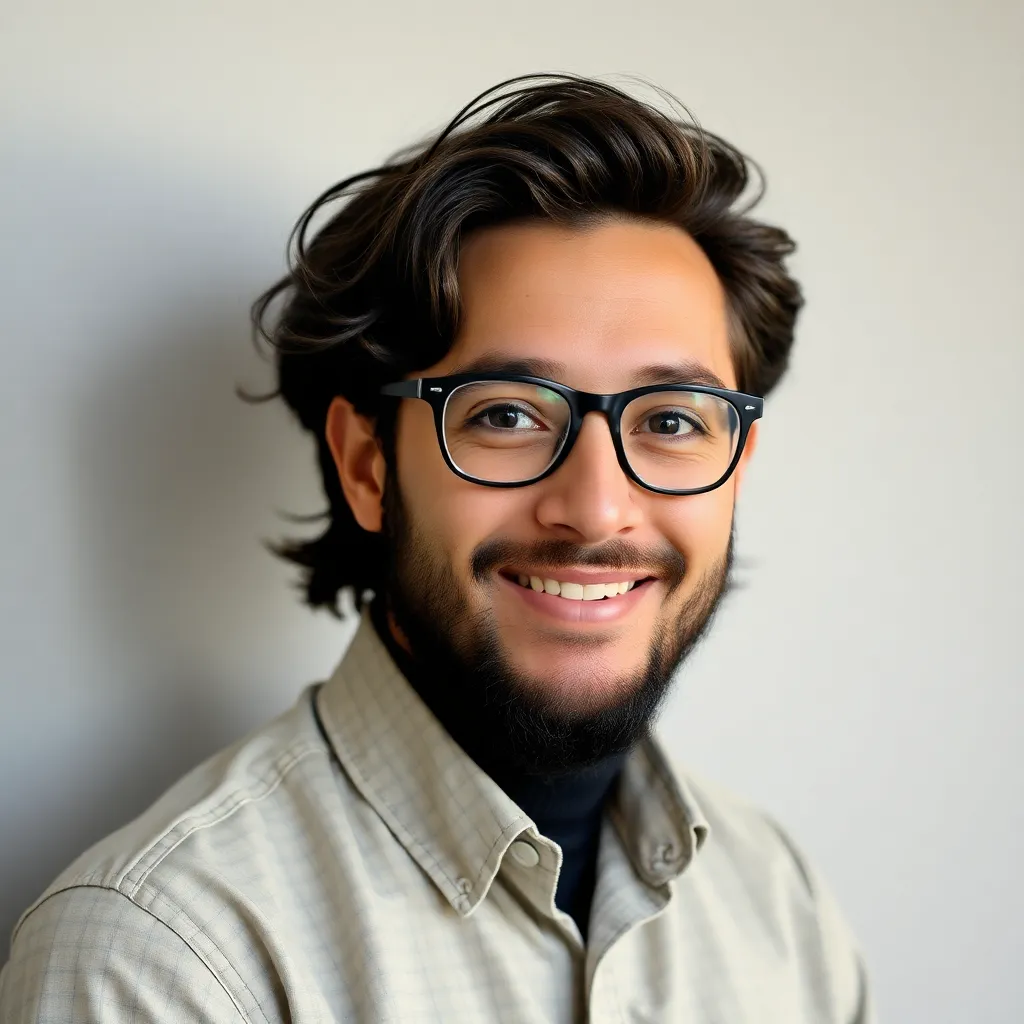
News Leon
Mar 20, 2025 · 5 min read
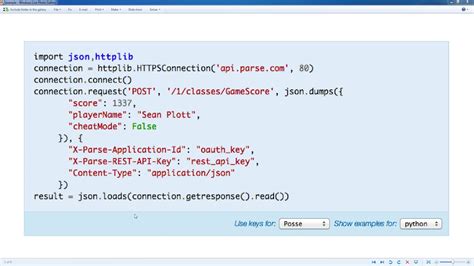
Table of Contents
Mastering the Art of 'end' in Python: A Comprehensive Guide
Python's print()
function offers a surprising amount of flexibility, far beyond simply displaying output to the console. One often-overlooked yet incredibly powerful feature is the end
parameter, which allows you to fine-tune the behavior of the print()
function in ways that dramatically improve code readability and functionality. This comprehensive guide will delve deep into the capabilities of end
in Python, covering its basics, advanced applications, and common use cases.
Understanding the Default Behavior of print()
Before exploring the nuances of the end
parameter, let's establish a baseline understanding of how print()
behaves by default. When you call print()
with a single argument (e.g., a string), Python automatically adds a newline character (\n
) at the end of the output. This means each print()
statement pushes the subsequent output to a new line.
print("Hello")
print("World")
This will produce:
Hello
World
Introducing the end
Parameter: Controlling the Terminator
The magic of end
lies in its ability to override this default newline behavior. The end
parameter allows you to specify a different character (or string) to be printed at the end of the output, thereby enabling greater control over how your output is formatted.
print("Hello", end=" ") # Note the space after "Hello"
print("World")
This code snippet will now produce:
Hello World
The end=" "
argument tells print()
to append a space instead of a newline, resulting in "Hello" and "World" appearing on the same line.
Advanced Applications of the end
Parameter
The applications of the end
parameter extend far beyond simple line concatenation. Let's explore some advanced use cases that demonstrate its power and versatility:
1. Creating Customized Output Formatting:
end
can be used to create custom output formats beyond simple space separation. For instance, you can create formatted tables or lists with specific delimiters:
name = "Alice"
age = 30
city = "New York"
print(f"{name}|{age}|{city}", end="\n") #Using a pipe as separator
print(f"Bob|25|London", end="\n")
This produces a table-like output:
Alice|30|New York
Bob|25|London
This technique is particularly useful when generating data for other applications or exporting data in specific formats like CSV.
2. Building Progress Indicators:
In scenarios involving lengthy operations, providing real-time feedback to the user is crucial. The end
parameter is incredibly helpful for building simple progress indicators:
import time
for i in range(5):
print(f"Processing... {i+1}/5", end="\r") # \r overwrites the previous line
time.sleep(1)
print("Process complete!") #New line after the loop finishes
The \r
(carriage return) character moves the cursor to the beginning of the line without advancing to the next line. Each iteration overwrites the previous progress message, creating a dynamic progress indicator.
3. Concatenating Strings Without Newlines:
Building complex strings efficiently is a common task. Using end
eliminates the need for manual string concatenation or the use of join()
, simplifying the code:
parts = ["This", "is", "a", "sentence."]
for part in parts:
print(part, end=" ")
print() # Add a newline at the end for clarity
This will print:
This is a sentence.
4. Creating Custom Separators in Loops:
When iterating through lists or other iterable objects and printing the elements, using end
allows for easy customization of the separator between items.
numbers = [1, 2, 3, 4, 5]
for i, num in enumerate(numbers):
print(num, end=", " if i < len(numbers) - 1 else "\n")
This will print:
1, 2, 3, 4, 5
The conditional assignment of end
ensures a comma is added between each number except the last, which is followed by a newline.
5. Interactive Prompts and User Input:
In interactive programs, you often want prompts to stay on the same line as user input. end
facilitates this cleanly:
name = input("Enter your name: ", end="")
print(f"\nHello, {name}!")
This keeps the prompt and input on the same line, improving the user experience.
Beyond Characters: Using Strings with end
The end
parameter isn't restricted to single characters; you can use strings of any length:
print("This is line 1", end="---End of Line---\n")
print("This is line 2")
Output:
This is line 1---End of Line---
This is line 2
This demonstrates the flexibility of using longer strings to customize the output termination.
end
in Conjunction with Other print()
Features
The power of end
is further amplified when combined with other features of the print()
function, such as sep
(separator between multiple arguments) and file redirection.
print("apple", "banana", "cherry", sep=", ", end=".\n")
This combines sep
for internal element separation and end
for the final output formatting.
Error Handling and Best Practices
While using end
offers significant flexibility, it's important to handle potential errors and follow best practices:
-
Avoid Overuse: While powerful, excessive use of
end
can make code less readable. Use it judiciously where it provides a clear benefit. -
Clarity: Ensure your use of
end
is clear and understandable within the context of your code. Comments can be helpful to clarify the intent. -
Consistency: Maintain consistency in your use of
end
throughout your project for better readability and maintainability. -
Testing: Always test your code to ensure that the use of
end
produces the desired output.
Conclusion: Mastering end
for Enhanced Python Output
The end
parameter in Python's print()
function is a deceptively powerful tool for manipulating output formatting and controlling console interactions. By understanding its capabilities and employing best practices, you can write more efficient, readable, and user-friendly Python code. From creating custom progress indicators to crafting elegant output formats, mastering end
significantly enhances your Python programming prowess. Remember to experiment, explore, and incorporate end
into your workflow where appropriate to elevate the quality of your projects.
Latest Posts
Latest Posts
-
Which Of The Following Is Equal To 5 1 3
Mar 21, 2025
-
Is A Country A Proper Noun
Mar 21, 2025
-
For The Circuit Shown In The Figure
Mar 21, 2025
-
How To Calculate Average Velocity On A Velocity Time Graph
Mar 21, 2025
-
Which Of The Following Is Not Found In Prokaryotic Cells
Mar 21, 2025
Related Post
Thank you for visiting our website which covers about How To Use End In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.