How To Get Absolute Value In Python
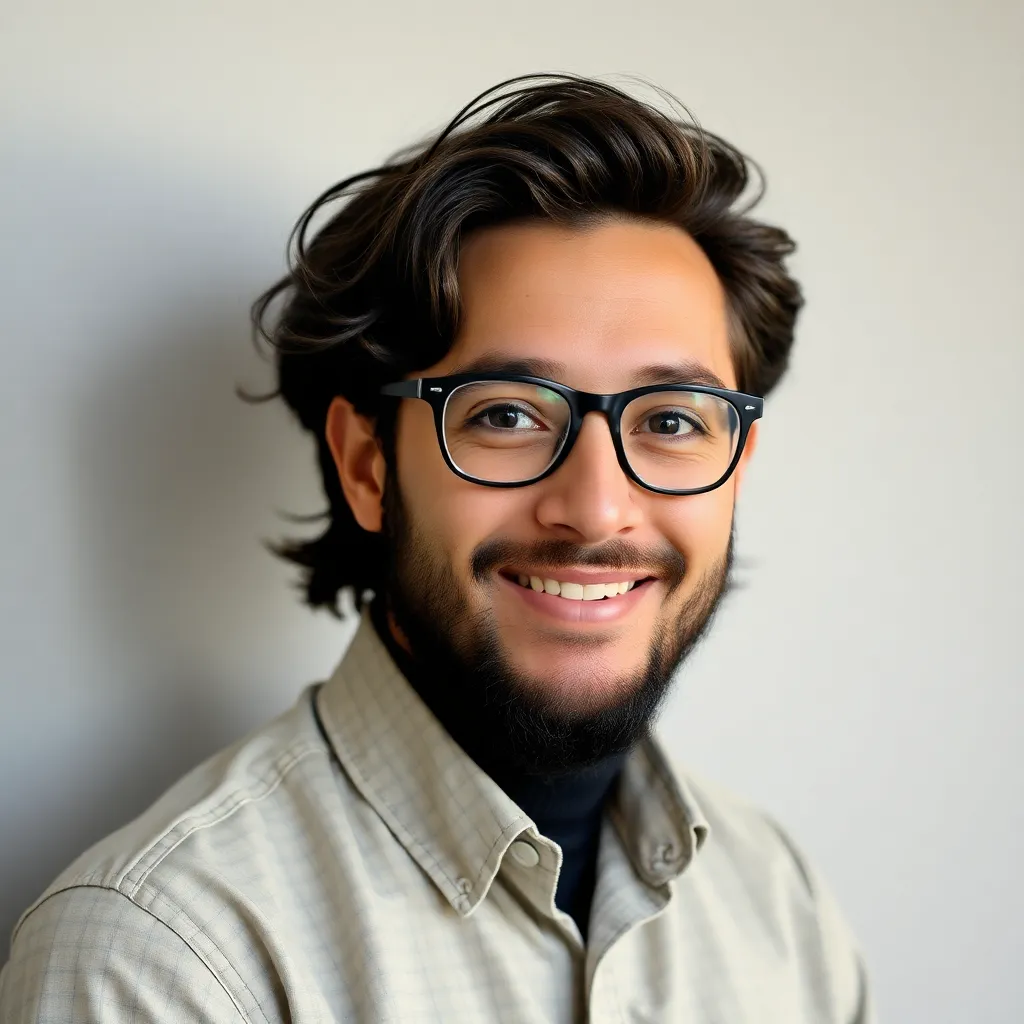
News Leon
Mar 15, 2025 · 5 min read
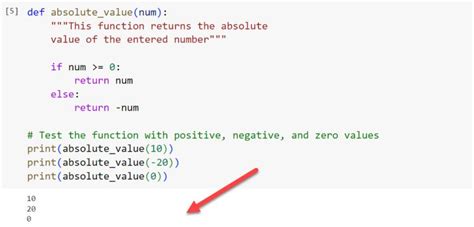
Table of Contents
How to Get the Absolute Value in Python: A Comprehensive Guide
Python, known for its readability and versatility, provides several efficient ways to obtain the absolute value of a number. Understanding how to do this is crucial for various programming tasks, from mathematical calculations to data manipulation and algorithm development. This comprehensive guide explores the different methods, highlighting their nuances and best use cases. We'll cover everything from basic approaches to more advanced scenarios, ensuring you're equipped to handle any absolute value challenge in your Python code.
Understanding Absolute Value
Before diving into the Python implementations, let's clarify what absolute value means. The absolute value of a number is its distance from zero on the number line. Therefore, it's always a non-negative number. For example:
- The absolute value of 5 is 5 (|5| = 5).
- The absolute value of -5 is also 5 (|-5| = 5).
- The absolute value of 0 is 0 (|0| = 0).
This concept is fundamental in mathematics and finds widespread application in programming.
Method 1: Using the abs()
Function
The most straightforward and recommended way to get the absolute value in Python is by using the built-in abs()
function. This function works flawlessly with integers, floating-point numbers, and even complex numbers.
# Examples using abs()
positive_number = 5
negative_number = -10
zero = 0
floating_point = -3.14
print(abs(positive_number)) # Output: 5
print(abs(negative_number)) # Output: 10
print(abs(zero)) # Output: 0
print(abs(floating_point)) # Output: 3.14
# Example with a complex number
complex_number = 3 + 4j
print(abs(complex_number)) # Output: 5.0 (magnitude of the complex number)
The abs()
function is concise, efficient, and readily available, making it the preferred method for most situations. Its broad compatibility with different number types adds to its versatility.
Method 2: Using Conditional Statements (if-else)
While less efficient than abs()
, using conditional statements provides a deeper understanding of the underlying logic. This approach is particularly useful for educational purposes or when dealing with custom data types where a dedicated abs()
equivalent isn't readily available.
def my_abs(x):
"""Calculates the absolute value using conditional statements."""
if x < 0:
return -x
else:
return x
print(my_abs(5)) # Output: 5
print(my_abs(-5)) # Output: 5
print(my_abs(0)) # Output: 0
print(my_abs(-3.14)) # Output: 3.14
This method explicitly checks if the number is negative; if it is, it returns the negation (making it positive). Otherwise, it returns the original number.
Method 3: Using the math.fabs()
Function (for floats only)
The math
module, part of Python's standard library, provides the math.fabs()
function specifically designed for floating-point numbers. While functionally similar to abs()
, math.fabs()
is slightly more specialized.
import math
float_num = -7.5
print(math.fabs(float_num)) # Output: 7.5
# Attempting to use math.fabs with an integer or complex number will result in a TypeError.
#print(math.fabs(-7)) #this will throw an error
It’s important to note that math.fabs()
only works with floating-point numbers; using it with integers or complex numbers will result in a TypeError
. Therefore, abs()
remains the more versatile option.
Handling Complex Numbers
Complex numbers, which have a real and an imaginary part (e.g., 3 + 4j), require a slightly different approach to defining absolute value. The abs()
function elegantly handles this by returning the magnitude (or modulus) of the complex number. This magnitude is calculated using the Pythagorean theorem: √(real² + imaginary²).
complex_num = 3 + 4j
magnitude = abs(complex_num)
print(magnitude) # Output: 5.0
The abs()
function automatically handles the calculation, making it a seamless way to obtain the magnitude of a complex number. No specialized functions are needed.
Error Handling and Robustness
While the abs()
function is generally robust, it's good practice to consider potential error scenarios when integrating it into larger applications. Although it handles various number types gracefully, it will raise a TypeError
if you attempt to pass in an unsupported data type (e.g., a string).
try:
result = abs("hello") # This will cause a TypeError
except TypeError:
print("Error: Input must be a number.")
This example showcases how error handling (using try-except
blocks) can enhance the robustness of your code by gracefully managing potential TypeError
exceptions.
Advanced Use Cases: Absolute Value in NumPy
NumPy, a powerful library for numerical computing in Python, provides vectorized operations for efficient array processing. This means you can calculate the absolute value of entire arrays at once without needing to loop through individual elements.
import numpy as np
array = np.array([-1, 2, -3, 4, -5])
absolute_array = np.abs(array)
print(absolute_array) # Output: [1 2 3 4 5]
NumPy's np.abs()
function offers significant performance improvements when working with large datasets compared to iterating through them and applying the standard abs()
function individually.
Absolute Value in Data Cleaning and Analysis
Absolute values are frequently used in data cleaning and analysis tasks. For example:
- Calculating errors or deviations: Determining the absolute difference between predicted and actual values helps quantify the error in a model's predictions.
- Handling negative values: In scenarios where negative values don't have a meaningful interpretation (like distances or magnitudes), converting them to their absolute values is essential.
- Data normalization: Absolute values can be employed in normalization techniques to scale data to a specific range, making it easier to compare and analyze.
Optimization and Performance
For most standard scenarios, the built-in abs()
function offers optimal performance. Its implementation is highly optimized within the Python interpreter. However, if you're working with extremely large datasets, NumPy's np.abs()
provides a considerable performance advantage due to its vectorized nature.
Conclusion: Choosing the Right Method
The best method for obtaining the absolute value in Python depends on your specific context:
- For most cases: The built-in
abs()
function is the simplest, most versatile, and efficient choice. - For educational purposes or understanding the underlying logic: The
if-else
approach is helpful. - For floating-point numbers only:
math.fabs()
can be used, butabs()
is generally preferred for its broader compatibility. - For large numerical arrays: NumPy's
np.abs()
is significantly faster and more efficient.
By understanding these various methods and their nuances, you can confidently incorporate absolute value calculations into your Python programs, ensuring both correctness and efficiency. Remember to handle potential errors gracefully using try-except
blocks, especially when working with user-supplied input or external data sources. Mastering absolute value calculations is a crucial step in becoming a proficient Python programmer.
Latest Posts
Latest Posts
-
Which Is Not A Cranial Bone Of The Skull
Mar 15, 2025
-
Mountain Range That Separates Europe And Asia
Mar 15, 2025
-
16 Out Of 40 As A Percentage
Mar 15, 2025
-
Which Of The Following Is A True Solution
Mar 15, 2025
-
How Many Vertices Does A Rectangular Pyramid Have
Mar 15, 2025
Related Post
Thank you for visiting our website which covers about How To Get Absolute Value In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.