Check If Character Is Letter Python
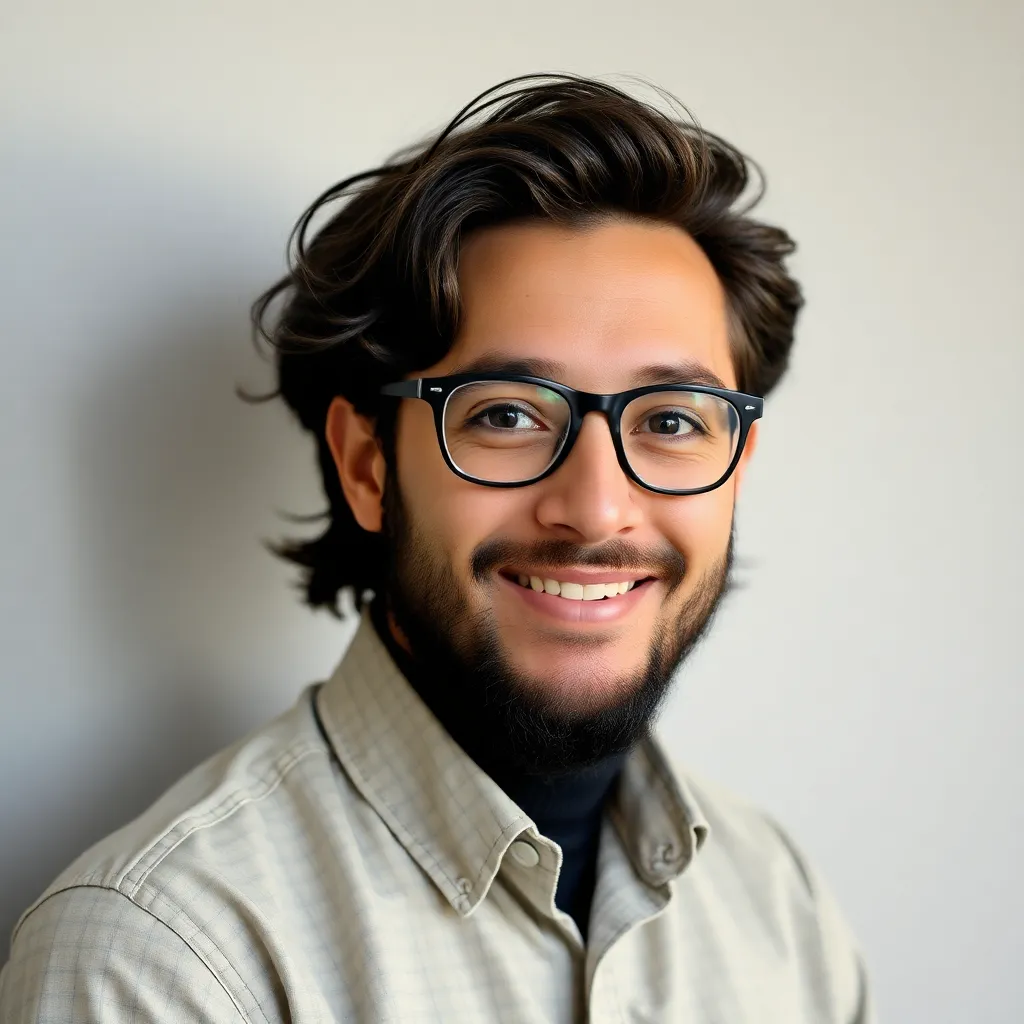
News Leon
Mar 24, 2025 · 7 min read
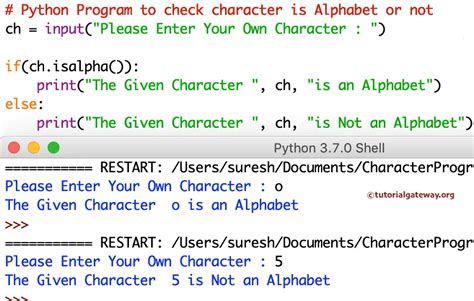
Table of Contents
Checking if a Character is a Letter in Python: A Comprehensive Guide
Determining whether a given character is a letter is a fundamental task in many programming scenarios, particularly those involving text processing, data validation, and string manipulation. Python, with its rich library of built-in functions and modules, offers several elegant ways to achieve this. This comprehensive guide explores various methods, compares their efficiency, and provides practical examples to help you confidently check if a character is a letter in your Python programs.
Understanding Character Classification in Python
Before diving into the methods, let's establish a clear understanding of what constitutes a "letter" in the context of Python. Generally, a letter refers to an alphabetic character, encompassing both uppercase (A-Z) and lowercase (a-z) letters from the English alphabet. However, depending on your needs, you might need to consider characters from other alphabets or even diacritical marks (accents, umlauts). The methods discussed here primarily focus on the standard English alphabet, but we'll also touch upon handling extended character sets.
Method 1: Using the isalpha()
Method
The most straightforward and Pythonic way to check if a character is a letter is to use the built-in isalpha()
string method. This method returns True
if all characters in a string are alphabetic characters and False
otherwise. Crucially, it's case-sensitive. Let's illustrate this with examples:
char1 = 'A'
char2 = 'a'
char3 = '1'
char4 = '
Latest Posts
Latest Posts
-
Most Chemical Digestion Takes Place In The
Mar 28, 2025
-
What Is The Conjugate Base Of Hso4
Mar 28, 2025
-
What Is The Function Of Areolar Tissue
Mar 28, 2025
-
What Is 6 25 As A Fraction
Mar 28, 2025
-
Which Of The Following Compounds Is Ionic
Mar 28, 2025
Related Post
Thank you for visiting our website which covers about Check If Character Is Letter Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.