A Circular Linked List Can Be Used To Implement
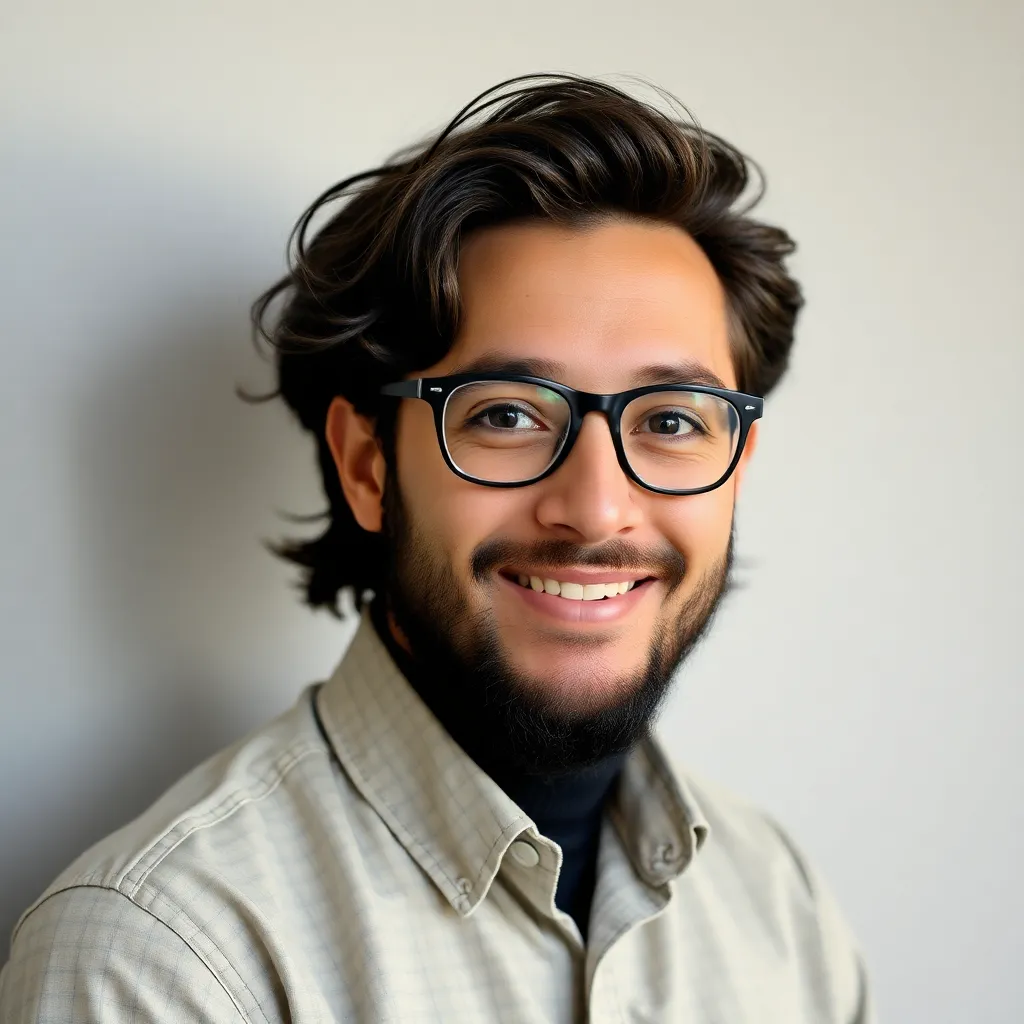
News Leon
Mar 23, 2025 · 6 min read
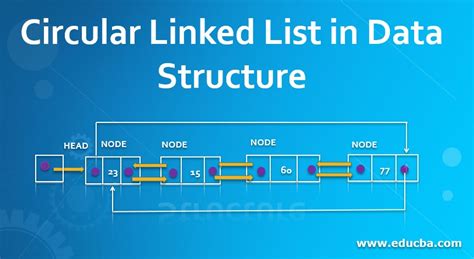
Table of Contents
A Circular Linked List Can Be Used To Implement: Exploring Diverse Applications
Circular linked lists, a fascinating data structure where the last node points back to the head, offer unique advantages over traditional linked lists. Their cyclical nature allows for elegant solutions to various programming problems. This article delves into the diverse applications of circular linked lists, exploring their strengths and demonstrating their practical utility in various scenarios.
Understanding Circular Linked Lists: A Foundation
Before diving into the applications, let's solidify our understanding of circular linked lists. Unlike singly linked lists, where the last node's next
pointer is NULL
, a circular linked list's last node's next
pointer points back to the head node, creating a closed loop. This seemingly simple modification opens up a world of possibilities.
Key Advantages: Why Choose a Circular Linked List?
- Efficient Traversal: Circular lists simplify traversal. You can start at any point and traverse the entire list without needing to check for
NULL
pointers. This is especially beneficial in applications requiring continuous looping. - Implementation of Queues and Deques: Circular linked lists provide an efficient way to implement queues and double-ended queues (deques) with O(1) time complexity for enqueue and dequeue operations.
- Round-Robin Scheduling: The cyclical nature lends itself naturally to round-robin scheduling algorithms, ensuring fairness in resource allocation.
- Memory Management: In some systems, using a circular linked list can improve memory allocation and deallocation efficiency.
Key Disadvantages: Potential Drawbacks
- Potential for Infinite Loops: Incorrect handling of pointers can easily lead to infinite loops during traversal. Careful coding is crucial.
- Slightly Increased Complexity: Implementing a circular linked list might involve slightly more complex code compared to a singly linked list.
Diverse Applications of Circular Linked Lists
Now, let's explore the various applications where circular linked lists shine:
1. Implementing Circular Buffers (Ring Buffers)
Circular buffers, also known as ring buffers, are exceptionally well-suited for managing data streams where the oldest data is overwritten by the newest data. This is crucial in real-time systems with limited memory. A circular linked list's cyclical nature perfectly mirrors the circular buffer's behavior.
How it works: Data is added to the tail of the list, and when the buffer is full, the head pointer moves forward, effectively overwriting the oldest element. This constant overwriting ensures efficient use of memory and avoids data loss.
Example Scenarios:
- Network Packet Handling: Storing incoming network packets temporarily before processing.
- Audio/Video Streaming: Buffering audio and video data for smooth playback.
- Real-time Data Acquisition: Temporarily storing sensor readings before analysis.
2. Efficient Implementation of Queues (FIFO)
A queue follows the First-In, First-Out (FIFO) principle. Circular linked lists provide an elegant way to implement queues with O(1) time complexity for both enqueue (adding to the rear) and dequeue (removing from the front) operations.
How it works: The head
pointer indicates the front of the queue, and the tail
pointer indicates the rear. Enqueueing involves adding a new node at the tail
, and dequeuing involves removing the node at the head
and updating the head
pointer accordingly. The circular nature ensures that once the tail
reaches the end, it wraps back to the beginning, effectively handling the buffer's cyclical nature.
Example Scenarios:
- Task Scheduling: Managing tasks waiting for processing.
- Printer Queues: Managing print jobs awaiting execution.
- Event Handling: Queuing events for processing in order of arrival.
3. Implementing Double-Ended Queues (Deques)
Double-ended queues (deques) allow insertion and deletion from both ends. Circular linked lists enable efficient implementation of deques, maintaining O(1) time complexity for operations at both ends.
How it works: Similar to queue implementation, but with operations at both the head
and tail
. Insertion and deletion at either end can be performed efficiently due to the circular nature.
Example Scenarios:
- Undo/Redo Functionality: Implementing undo and redo functionality in applications.
- Browser History: Managing browser history efficiently.
- Expression Evaluation: Processing expressions using a stack-like structure.
4. Round-Robin Scheduling in Operating Systems
Round-robin scheduling is a CPU scheduling algorithm where each process gets a fixed time slice to execute. Once the time slice is over, the process is preempted, and the next process in the queue gets its turn. Circular linked lists are ideally suited for this scenario.
How it works: Processes are stored in a circular linked list. The scheduler iterates through the list, giving each process a time slice. Once a process completes or its time slice is over, the scheduler moves to the next process in the list. This ensures fair allocation of CPU time.
Example Scenarios:
- Time-sharing Operating Systems: Managing multiple processes concurrently.
- Real-time Systems: Scheduling tasks in a fair and timely manner.
5. Memory Management in Specific Applications
While not as common as other applications, circular linked lists can improve memory management in specific situations. They can be employed in custom memory allocators or in systems where continuous allocation and deallocation of memory blocks are frequent. The circular structure enables efficient reuse of freed memory blocks.
How it works: Free memory blocks are linked together in a circular linked list. When memory is allocated, a block is removed from the list. When memory is freed, the block is added back to the list. This approach reduces fragmentation and improves overall memory management efficiency.
6. Implementing a Simple Game Loop
In game development, a circular linked list can elegantly manage the game loop’s tasks or entities. The cyclical nature perfectly matches the repetitive nature of game updates.
How it works: Each entity or game task is a node in the list. The game loop iterates through the list, processing each node's update function. The circularity ensures that the loop continues seamlessly.
7. Implementing a Circular Queue for Data Transmission
In data communication, a circular queue can be used to buffer data being sent or received. This structure prevents data loss when the rate of data generation and processing differs.
How it Works: The transmitting and receiving sides use circular queues to handle data flow. The queues provide a buffer between the producer (sender) and the consumer (receiver) ensuring efficient transfer.
Choosing the Right Data Structure: When to Use Circular Linked Lists
While circular linked lists offer many advantages, they aren’t always the best choice. Consider these points:
- Complexity: Implementing and managing circular linked lists is slightly more complex than singly linked lists. Choose them only when the benefits outweigh the increased complexity.
- Error Prone: Incorrect handling of pointers can easily lead to infinite loops. Thorough testing is vital.
- Alternative Structures: Consider alternative data structures like arrays or doubly linked lists if the application doesn’t specifically benefit from the circular nature.
Conclusion: Harnessing the Power of Circular Linked Lists
Circular linked lists, though often overlooked, offer a powerful and elegant solution to a variety of programming problems. Their cyclical nature allows for efficient implementation of queues, deques, circular buffers, and various other applications. Understanding their strengths and weaknesses is crucial for choosing the right data structure for your specific needs. By carefully considering the advantages and disadvantages, developers can leverage the unique capabilities of circular linked lists to create efficient and robust software solutions. This comprehensive exploration highlights their versatility and potential for creating innovative and effective systems. Remember careful planning and meticulous implementation are key to utilizing their power effectively.
Latest Posts
Latest Posts
-
What Are The Units Of Entropy
Mar 24, 2025
-
The Genetic Material Of Hiv Consists Of
Mar 24, 2025
-
In The Figure Two Particles Each With Mass M
Mar 24, 2025
-
Which Compound Contains A Triple Bond
Mar 24, 2025
-
Name The Blood Vessel That Delivers Blood To Each Glomerulus
Mar 24, 2025
Related Post
Thank you for visiting our website which covers about A Circular Linked List Can Be Used To Implement . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.