What Does Rstrip Do In Python
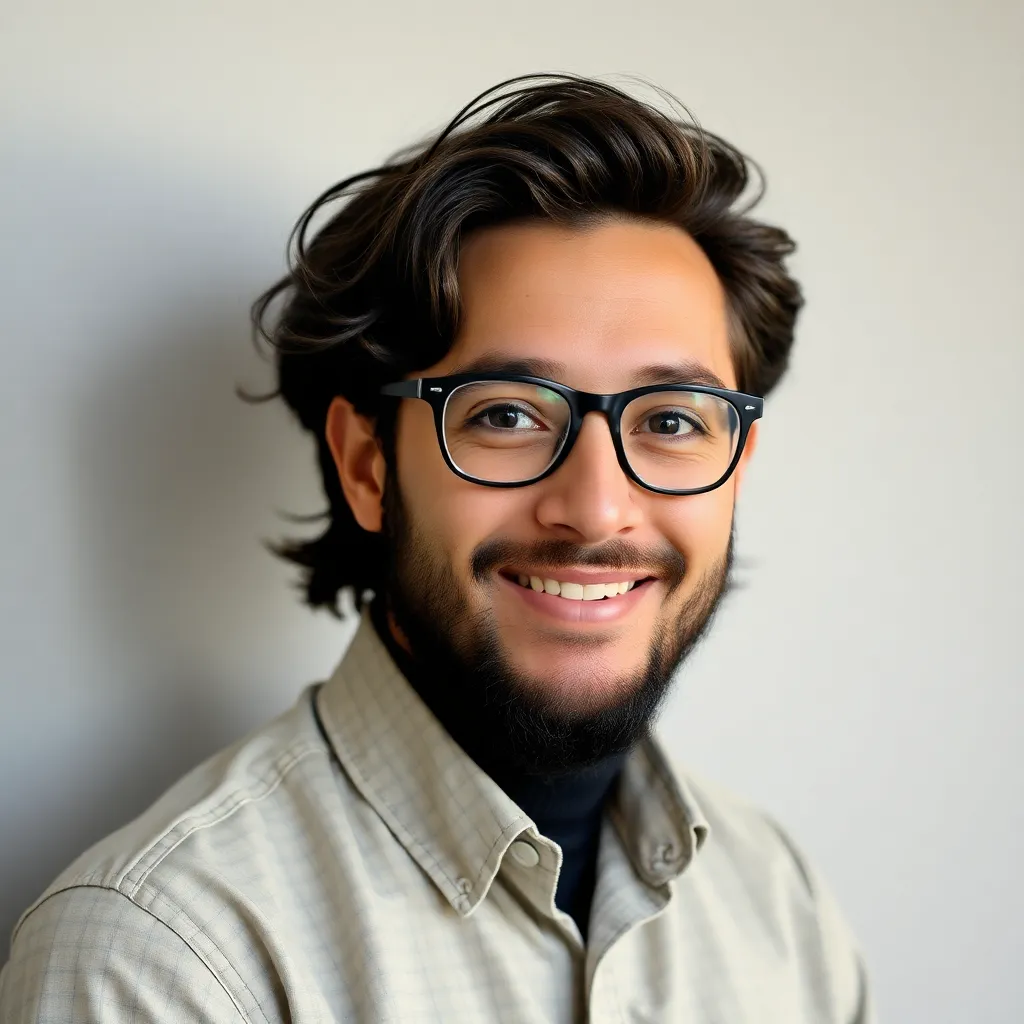
News Leon
Mar 25, 2025 · 5 min read
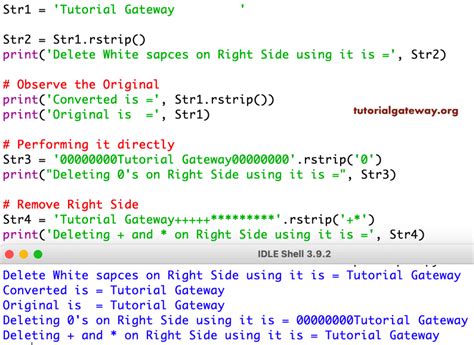
Table of Contents
What Does rstrip() Do in Python? A Deep Dive into String Manipulation
Python's built-in string methods offer a powerful toolkit for text manipulation. Among these, rstrip()
stands out as a crucial function for cleaning and preparing strings for various applications. This comprehensive guide explores the functionality of rstrip()
in detail, examining its core behavior, variations, common use cases, and potential pitfalls. We'll delve beyond the basics, providing practical examples and advanced techniques to solidify your understanding.
Understanding the Core Functionality of rstrip()
At its heart, rstrip()
is a string method designed to remove trailing characters from a string. "Trailing" refers to characters at the end of the string. Crucially, rstrip()
removes characters only from the right side (hence the "r" in rstrip()
). This distinguishes it from lstrip()
(which removes leading characters) and strip()
(which removes both leading and trailing characters).
The Basic Syntax:
The basic syntax is straightforward:
string.rstrip([chars])
string
: The string you want to modify.[chars]
: (Optional) A set of characters to remove. If omitted, it removes whitespace characters (spaces, tabs, newlines, etc.) by default.
Example 1: Removing Whitespace
my_string = "Hello World! "
cleaned_string = my_string.rstrip()
print(f"Original string: '{my_string}'")
print(f"Cleaned string: '{cleaned_string}'")
This will output:
Original string: 'Hello World! '
Cleaned string: 'Hello World!'
Notice how the trailing spaces are removed.
Example 2: Removing Specific Characters
my_string = "Python programming!!!***"
cleaned_string = my_string.rstrip("!*")
print(f"Original string: '{my_string}'")
print(f"Cleaned string: '{cleaned_string}'")
This outputs:
Original string: 'Python programming!!!***'
Cleaned string: 'Python programming!!!'
Here, we specified "!" and "*" as characters to remove from the right side. Only those characters are removed; other trailing characters remain.
Beyond the Basics: Advanced rstrip()
Techniques
While the basic usage is clear, the true power of rstrip()
lies in its flexibility and application in more complex scenarios.
1. Removing Multiple Character Sets
rstrip()
can efficiently handle removing multiple types of trailing characters simultaneously.
my_string = "Data Science \t\n!!!"
cleaned_string = my_string.rstrip(" !\t\n") #Removes spaces, exclamation marks, tabs, and newlines
print(f"Original string: '{my_string}'")
print(f"Cleaned string: '{cleaned_string}'")
This example demonstrates the removal of spaces, exclamation marks, tabs (\t
), and newlines (\n
) from the right end.
2. In-place Modification (Using in-place
methods)
While rstrip()
itself doesn't modify the original string in-place (it returns a new string), this behavior can be mimicked for efficiency in scenarios with large strings, using assignment:
my_string = " Extra spaces at the end. "
my_string = my_string.rstrip() # Assign the result back to the original variable
print(f"Modified string: '{my_string}'")
This method avoids creating unnecessary copies of the string, potentially improving performance.
3. Handling Unicode Characters
rstrip()
gracefully handles Unicode characters, allowing for the removal of trailing characters from diverse languages and scripts.
my_string = "你好世界! " # "Hello World!" in Chinese with trailing spaces and a fullwidth space
cleaned_string = my_string.rstrip()
print(f"Original string: '{my_string}'")
print(f"Cleaned string: '{cleaned_string}'")
The trailing whitespace, including the full-width space, will be correctly removed.
4. Integration with Other String Methods
rstrip()
works seamlessly with other Python string methods. This allows for chained operations to perform complex text cleaning efficiently.
my_string = " Unwanted characters at both ends !!!"
cleaned_string = my_string.strip().rstrip("!") #strip removes leading and trailing whitespace, then rstrip removes trailing !
print(f"Cleaned string: '{cleaned_string}'")
This efficiently cleans the string of both leading and trailing characters of different kinds.
Common Use Cases of rstrip()
The applications of rstrip()
are extensive and span various programming domains:
1. Data Cleaning and Preprocessing
In data science and machine learning, rstrip()
is essential for cleaning datasets. Raw text data often contains extra whitespace or unwanted characters at the end of strings, which can lead to errors or inconsistencies in analysis. rstrip()
helps to standardize and prepare data for processing.
2. File Processing
When working with text files, rstrip()
is crucial for removing trailing whitespace or newline characters from lines read from a file. This ensures that each line is handled consistently.
3. Web Development
In web development, rstrip()
can be used to sanitize user inputs, removing trailing characters that could pose security risks or lead to unexpected behavior.
4. Network Programming
In network communication, rstrip()
helps to clean up received data, removing any trailing control characters or whitespace that might be added during transmission.
Potential Pitfalls and Considerations
While rstrip()
is a powerful tool, it's essential to be aware of potential pitfalls:
1. Unexpected Character Removal
Carefully specify the characters to remove using [chars]
. Incorrectly specifying characters can lead to unintended removals.
2. Performance Considerations for Extremely Large Strings
For extremely large strings, the creation of a new string with rstrip()
might be computationally expensive. Consider alternative approaches or in-place modification techniques in such cases.
Conclusion: Mastering rstrip()
for Efficient String Manipulation
Python's rstrip()
method is a versatile and indispensable tool for manipulating strings. Understanding its core functionality, variations, and common use cases empowers you to efficiently clean, preprocess, and prepare text data for various applications. By carefully considering potential pitfalls and leveraging its integration with other string methods, you can unlock the full potential of rstrip()
in your Python programming endeavors. This detailed guide provides a robust foundation for confident and effective utilization of rstrip()
across your projects, enhancing code clarity and robustness in string manipulation. Remember to always test your code thoroughly to ensure the desired outcome is achieved and unexpected behavior is mitigated. Mastering this seemingly simple function unlocks significant potential in your Python projects.
Latest Posts
Latest Posts
-
Which Of The Following Represent Statistical Information
Mar 26, 2025
-
Which Of The Following Is A Steroid Hormone
Mar 26, 2025
-
Osmosis Refers To The Movement Of
Mar 26, 2025
-
Digit 9 Is Always At Hundreds Place
Mar 26, 2025
-
The Rime Of Ancient Mariner Summary
Mar 26, 2025
Related Post
Thank you for visiting our website which covers about What Does Rstrip Do In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.