What Does It Mean To Initialize A Variable
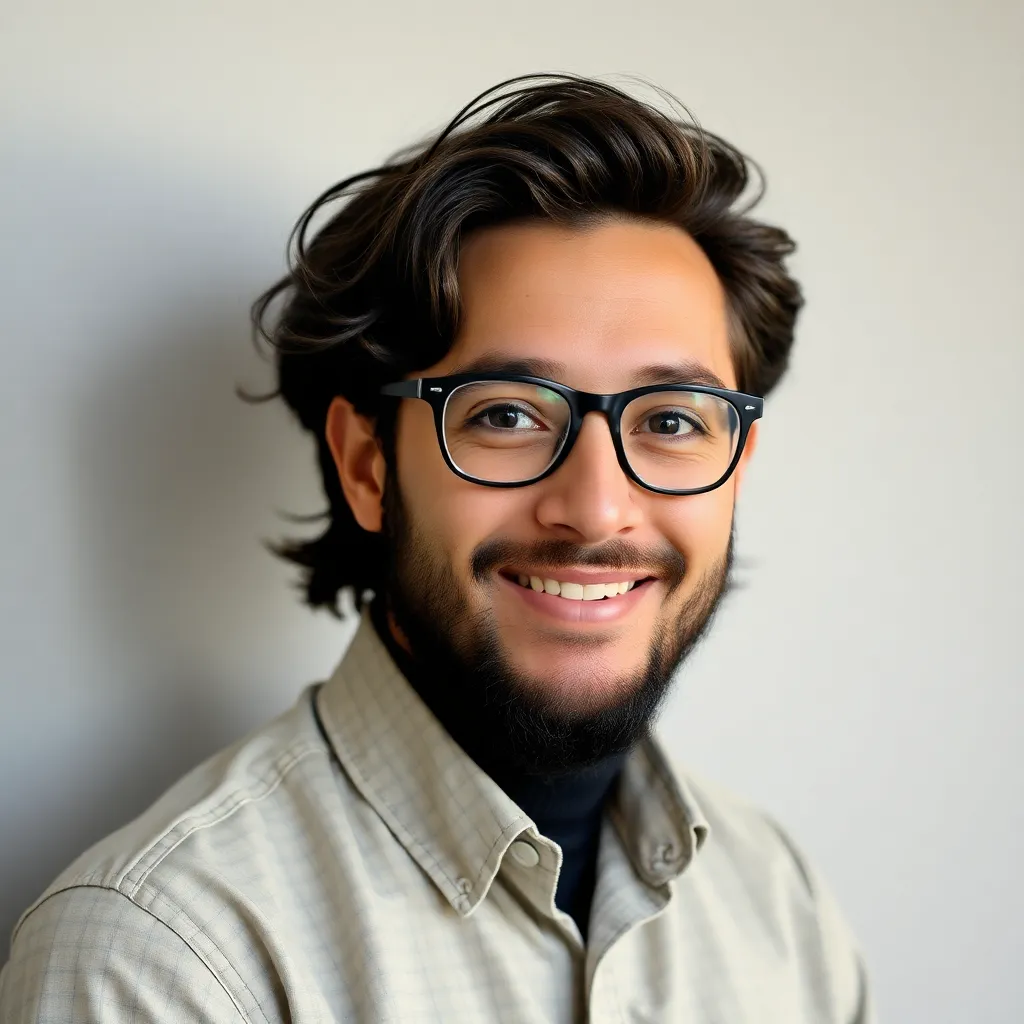
News Leon
Mar 15, 2025 · 5 min read
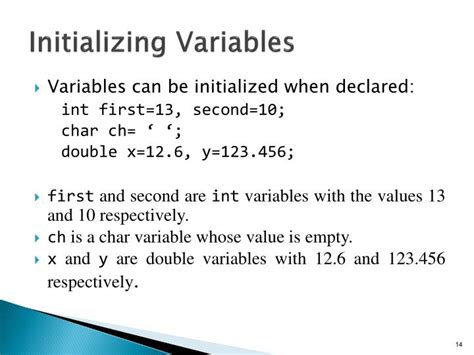
Table of Contents
What Does It Mean to Initialize a Variable? A Deep Dive into Programming Fundamentals
Initializing a variable might seem like a minor detail in programming, but it's a foundational concept with significant implications for code correctness, efficiency, and security. Understanding variable initialization is crucial for writing robust and reliable software. This comprehensive guide will explore the meaning of variable initialization, its importance, different approaches across various programming languages, and common pitfalls to avoid.
Understanding Variable Initialization: The Basics
In programming, a variable is a named storage location that holds a value. Think of it like a container that can store different types of data, such as numbers, text, or more complex structures. Initialization is the process of assigning an initial value to a variable when it's declared. This initial value sets the variable's starting state before it's used in any operations.
Why Initialize?
Why bother initializing variables? Several crucial reasons highlight its importance:
-
Preventing Undefined Behavior: Uninitialized variables contain unpredictable values (garbage data) left over from previous memory usage. Using these undefined values can lead to unexpected results, program crashes, or security vulnerabilities. Initialization guarantees a known starting point, eliminating this uncertainty.
-
Ensuring Correct Program Logic: Proper initialization ensures your code functions as intended. If a variable's value is crucial for a calculation or decision, an uninitialized variable can lead to incorrect outputs or erroneous program flow.
-
Improving Code Readability and Maintainability: Explicitly initializing variables makes your code clearer and easier to understand. Readers can immediately see the variable's intended starting state, reducing ambiguity and improving maintainability.
-
Avoiding Errors: Many programming languages and compilers have mechanisms to detect uninitialized variable usage. Initialization helps prevent warnings or errors during compilation or runtime, streamlining the development process.
-
Security: Uninitialized variables can create security vulnerabilities. An attacker might exploit the unpredictable values in uninitialized memory to gain unauthorized access or control of the system.
Initialization Techniques Across Programming Languages
The specific syntax and rules for initializing variables vary slightly depending on the programming language. However, the underlying principle remains consistent: assign a starting value when you declare the variable.
C++ Initialization
C++ offers several ways to initialize variables:
- Direct Initialization: The simplest method is to assign a value directly during declaration:
int age = 30;
double price = 99.99;
std::string name = "John Doe";
- Uniform Initialization: Introduced in C++11, uniform initialization improves consistency and avoids potential ambiguities:
int age {30};
double price {99.99};
std::string name {"John Doe"};
- Initialization Lists (for classes): When creating objects of a class, you use initialization lists within the constructor:
class Person {
public:
int age;
std::string name;
Person(int a, std::string n) : age(a), name(n) {}
};
- Default Initialization: If no value is provided, the variable's default value depends on its type (e.g., 0 for integers, 0.0 for doubles, an empty string for strings).
Java Initialization
Java emphasizes type safety and requires explicit initialization for variables within methods. Instance variables (those within a class but outside of methods) are automatically initialized to default values if not explicitly initialized.
int age = 30;
double price = 99.99;
String name = "John Doe";
// Instance variable with default initialization (0 for int)
int count;
public void myMethod() {
int x; // Needs to be initialized before use within the method
x = 10;
System.out.println(x);
}
Python Initialization
Python is dynamically typed, meaning you don't explicitly declare variable types. Initialization happens simply by assigning a value:
age = 30
price = 99.99
name = "John Doe"
Python also allows for simultaneous initialization of multiple variables:
a, b, c = 10, 20, 30
JavaScript Initialization
Similar to Python, JavaScript is dynamically typed. Initialization is done through assignment:
let age = 30;
let price = 99.99;
let name = "John Doe";
// Using const for constants (cannot be reassigned)
const PI = 3.14159;
JavaScript's let
keyword indicates a variable that can be reassigned, while const
defines a constant whose value cannot be changed after initialization.
Advanced Initialization Techniques
Beyond basic assignment, more sophisticated initialization techniques exist, particularly in object-oriented programming:
-
Constructor Initialization: In object-oriented languages, constructors are special methods that initialize an object's attributes when a new object is created. This is crucial for setting up objects in a consistent and valid state.
-
Static Initialization: Some languages allow for static initialization, where variables are initialized once when the program or class is loaded, rather than each time an object is created. This can be useful for constants or shared resources.
-
Initialization Blocks: Some languages provide initialization blocks (like in Java) to perform complex initialization logic before the constructor is called. This is useful for tasks that require multiple steps or depend on external factors.
Common Pitfalls and Best Practices
While initialization is straightforward, several common mistakes can lead to problems:
-
Uninitialized Variables: The most critical error is failing to initialize a variable before using it. This leads to undefined behavior. Always explicitly initialize variables, even if they have default values.
-
Incorrect Initialization: Assigning an inappropriate value (e.g., a wrong data type) during initialization can cause errors or unexpected results. Carefully review your variable types and assigned values.
-
Initialization Order: In complex programs with multiple variables and classes, understand the order of initialization to avoid dependencies on uninitialized variables.
-
Over-Initialization: Don't unnecessarily initialize a variable more than once. This can lead to redundant code and potential confusion.
Best Practices:
- Always Initialize: Make it a habit to initialize all variables before using them.
- Use Meaningful Names: Choose descriptive names for your variables to clarify their purpose and initial values.
- Use Appropriate Data Types: Ensure the variable's data type accurately reflects the kind of values it will hold.
- Document Initialization: Clearly document the purpose of each variable and its initial value, especially in more complex code.
- Employ Static Analysis Tools: Tools can automatically detect potential uninitialized variable errors during compilation or static analysis.
Conclusion: The Importance of Initialization in Robust Code
Variable initialization is not a mere formality; it's a fundamental programming practice that significantly impacts the reliability, security, and maintainability of your code. By understanding the various techniques and pitfalls involved, you can write more robust, efficient, and secure applications. Consistent and correct variable initialization is a cornerstone of good software engineering practices, fostering code clarity, preventing errors, and ultimately improving the overall quality of your programs. Always prioritize initialization, and remember, it is a small step that prevents many large problems down the line.
Latest Posts
Latest Posts
-
Is Boiling Water A Physical Change
Mar 17, 2025
-
Is A Webcam An Input Or Output Device
Mar 17, 2025
-
Word For A Person Who Uses Big Words
Mar 17, 2025
-
What Is 375 As A Percentage
Mar 17, 2025
-
Which Is The Correct Order Of The Scientific Method
Mar 17, 2025
Related Post
Thank you for visiting our website which covers about What Does It Mean To Initialize A Variable . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.