What Does Isalpha Do In Python
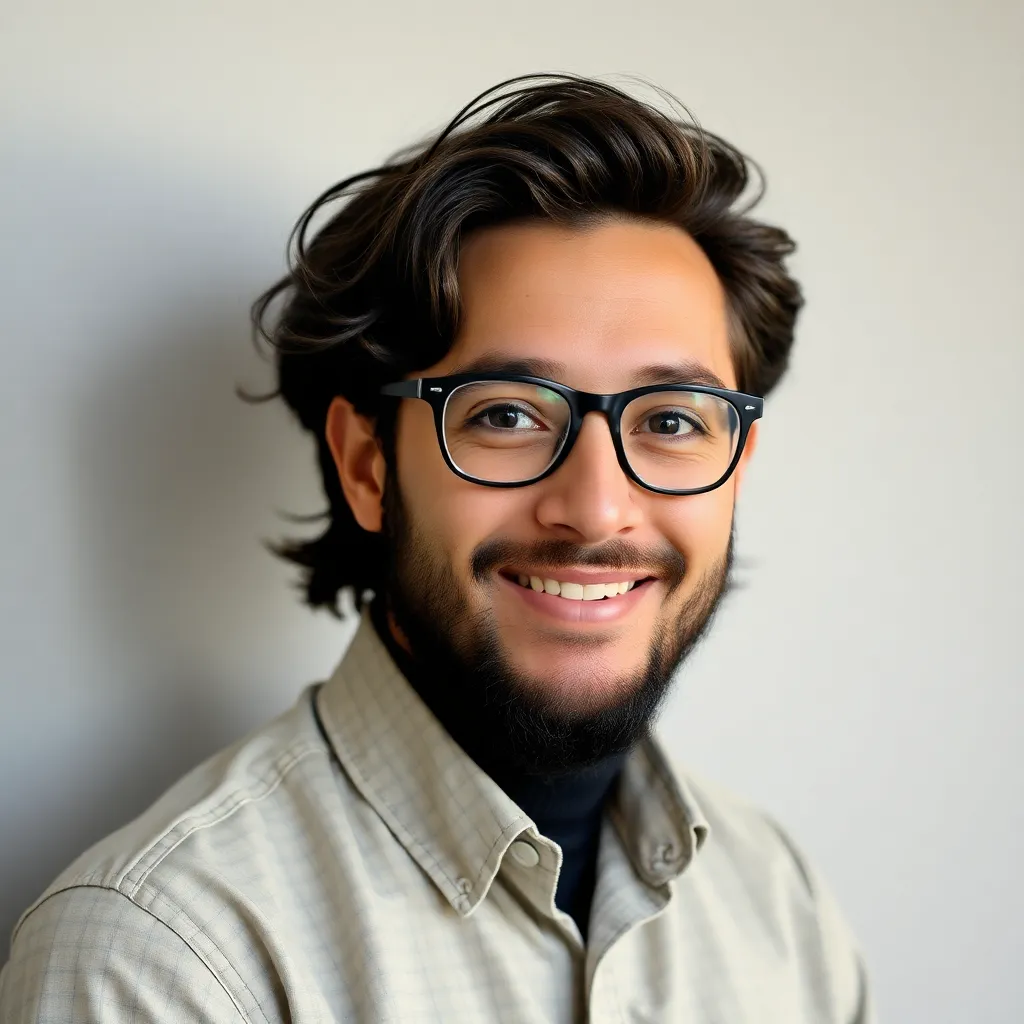
News Leon
Mar 29, 2025 · 6 min read
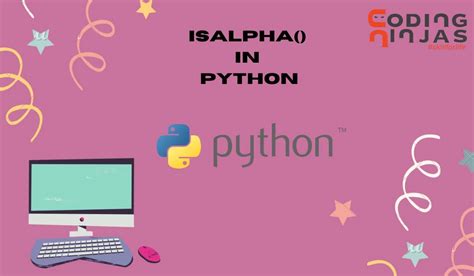
Table of Contents
What Does isalpha()
Do in Python? A Deep Dive into String Character Checks
Python's built-in string methods are powerful tools for manipulating and analyzing text data. Among these, isalpha()
plays a crucial role in determining the character composition of strings. This comprehensive guide will explore the functionality of isalpha()
, its applications, variations, potential pitfalls, and best practices for its effective usage within your Python programs.
Understanding isalpha()
's Core Function
The isalpha()
method is a string function specifically designed to check if all characters within a given string are alphabetic. In essence, it verifies whether the string consists exclusively of letters from the alphabet (a-z, A-Z). It returns True
if the condition is met; otherwise, it returns False
.
Key Characteristics:
- Case-Insensitive:
isalpha()
considers both uppercase and lowercase letters as alphabetic characters. "Hello" and "hello" will both returnTrue
. - Space Sensitivity: Spaces, numbers, punctuation marks, and any non-alphabetic characters will cause
isalpha()
to returnFalse
. - Unicode Support: Modern Python versions offer excellent Unicode support.
isalpha()
correctly handles characters from various alphabets, including accented characters and those outside the basic Latin alphabet. - Empty Strings: An empty string (
""
) will returnFalse
because it doesn't contain any alphabetic characters.
Practical Applications of isalpha()
isalpha()
finds application across diverse programming tasks related to text processing, data validation, and user input handling. Here are some prime examples:
1. Data Validation and Input Sanitization
One of the most common uses is validating user input. Imagine a registration form requiring a username consisting solely of alphabetic characters. isalpha()
provides a straightforward mechanism for enforcing this rule:
username = input("Enter your username (alphabetic characters only): ")
if username.isalpha():
print("Valid username.")
else:
print("Invalid username. Please use only alphabetic characters.")
This prevents the submission of usernames with numbers, symbols, or spaces, enhancing data integrity and security.
2. Text Preprocessing and Cleaning
In natural language processing (NLP) and data analysis, text often requires preprocessing to remove non-alphabetic elements. isalpha()
can be integrated into data cleaning pipelines to filter out unwanted characters:
text = "This is a sample string with 123 numbers and some symbols like !@#$."
cleaned_text = "".join([char for char in text if char.isalpha() or char.isspace()])
print(cleaned_text) # Output: This is a sample string with numbers and some symbols like
This code snippet iterates through each character, retaining only alphabetic characters and spaces, resulting in cleaner text for subsequent analysis.
3. Word Segmentation and Tokenization
During text analysis tasks, breaking down text into individual words (tokenization) is essential. isalpha()
can assist in accurately identifying word boundaries:
text = "This-is,a;sample.text"
words = []
current_word = ""
for char in text:
if char.isalpha():
current_word += char
else:
if current_word:
words.append(current_word)
current_word = ""
if current_word:
words.append(current_word)
print(words) # Output: ['This', 'is', 'a', 'sample', 'text']
By identifying alphabetic sequences, this method effectively segments the input string into a list of words.
4. Password Validation
While not exclusively relying on isalpha()
, it's part of a more comprehensive password validation process. Forcing users to include alphabetic characters alongside numbers and symbols enhances password strength:
password = input("Enter a password (at least one letter and one number): ")
if not password.isalpha() and any(char.isdigit() for char in password):
print("Strong password!")
else:
print("Weak password. Please include at least one letter and one number.")
5. Analyzing Character Frequency
isalpha()
can be leveraged to determine the proportion of alphabetic characters in a given text. This information could be valuable in various applications, such as analyzing the readability of text or identifying potential data errors:
text = "This is a sample string!"
alpha_count = sum(1 for char in text if char.isalpha())
total_chars = len(text)
alpha_percentage = (alpha_count / total_chars) * 100
print(f"Alphabetic character percentage: {alpha_percentage:.2f}%")
Beyond isalpha()
: Exploring Related Methods
Python provides a suite of related string methods for character classification, each serving a specific purpose. These methods offer a comprehensive toolkit for text analysis:
isalnum()
: Checks if all characters are alphanumeric (letters or numbers).isdigit()
: Checks if all characters are digits (0-9).islower()
: Checks if all characters are lowercase letters.isupper()
: Checks if all characters are uppercase letters.istitle()
: Checks if the string is titlecased (each word starts with an uppercase letter).isspace()
: Checks if all characters are whitespace characters (spaces, tabs, newlines).
These methods, when combined, provide fine-grained control over character classification within your Python programs. For instance, you could create a more robust password validator that incorporates multiple character types.
Potential Pitfalls and Best Practices
While isalpha()
is a straightforward function, understanding its limitations and following best practices is crucial for error-free and efficient code:
- Locale Considerations: While
isalpha()
handles a broad range of Unicode characters, subtle variations in character classification can occur depending on the locale settings. For applications requiring high precision in character classification across different languages, consider using locale-aware libraries. - Performance Optimization: For very large strings, repeatedly calling
isalpha()
on each character might impact performance. Consider using more optimized approaches, such as list comprehensions or generator expressions. - Clarity and Readability: Always prioritize clear and concise code. Using descriptive variable names and adding comments makes your code easier to understand and maintain. Avoid overly complex nested conditions using these string methods.
- Error Handling: Always anticipate potential errors, such as unexpected input formats or non-string data. Include appropriate error handling mechanisms (e.g.,
try-except
blocks) to gracefully manage such situations.
Advanced Applications and Integration
The power of isalpha()
truly shines when combined with other Python features and libraries:
- Regular Expressions: Integrating
isalpha()
with regular expressions allows for sophisticated pattern matching. For instance, you could use regular expressions to extract words from text containing mixed alphabetic and non-alphabetic characters while leveragingisalpha()
for validation. - Pandas DataFrames: In data analysis with Pandas,
isalpha()
can be applied to entire columns of a DataFrame, efficiently checking the character composition of textual data. - Natural Language Processing (NLP):
isalpha()
plays a pivotal role in NLP tasks, from tokenization and stemming to part-of-speech tagging and sentiment analysis.
Conclusion: Mastering isalpha()
for Robust Text Processing
Python's isalpha()
method is a fundamental tool for anyone working with text data. By understanding its functionality, applications, and limitations, you can significantly enhance the robustness and efficiency of your Python programs. Its seamless integration with other Python features and libraries further expands its utility, making it an indispensable asset for developers engaged in diverse text processing tasks. Remember to combine isalpha()
with other character classification methods and error handling techniques to create robust and reliable applications. The careful application of these methods will ensure your text processing routines are not only accurate but also optimized for efficiency and clarity.
Latest Posts
Latest Posts
-
What Is The Reciprocal Of 1 6
Mar 31, 2025
-
What Mineral Is The Hardest Known Substance In Nature
Mar 31, 2025
-
Which Organelle Is Enclosed By A Double Membrane
Mar 31, 2025
-
Compare And Contrast An Ecosystem And A Habitat
Mar 31, 2025
-
Network Layer Firewall Works As A
Mar 31, 2025
Related Post
Thank you for visiting our website which covers about What Does Isalpha Do In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.