What Does Count Do In Python
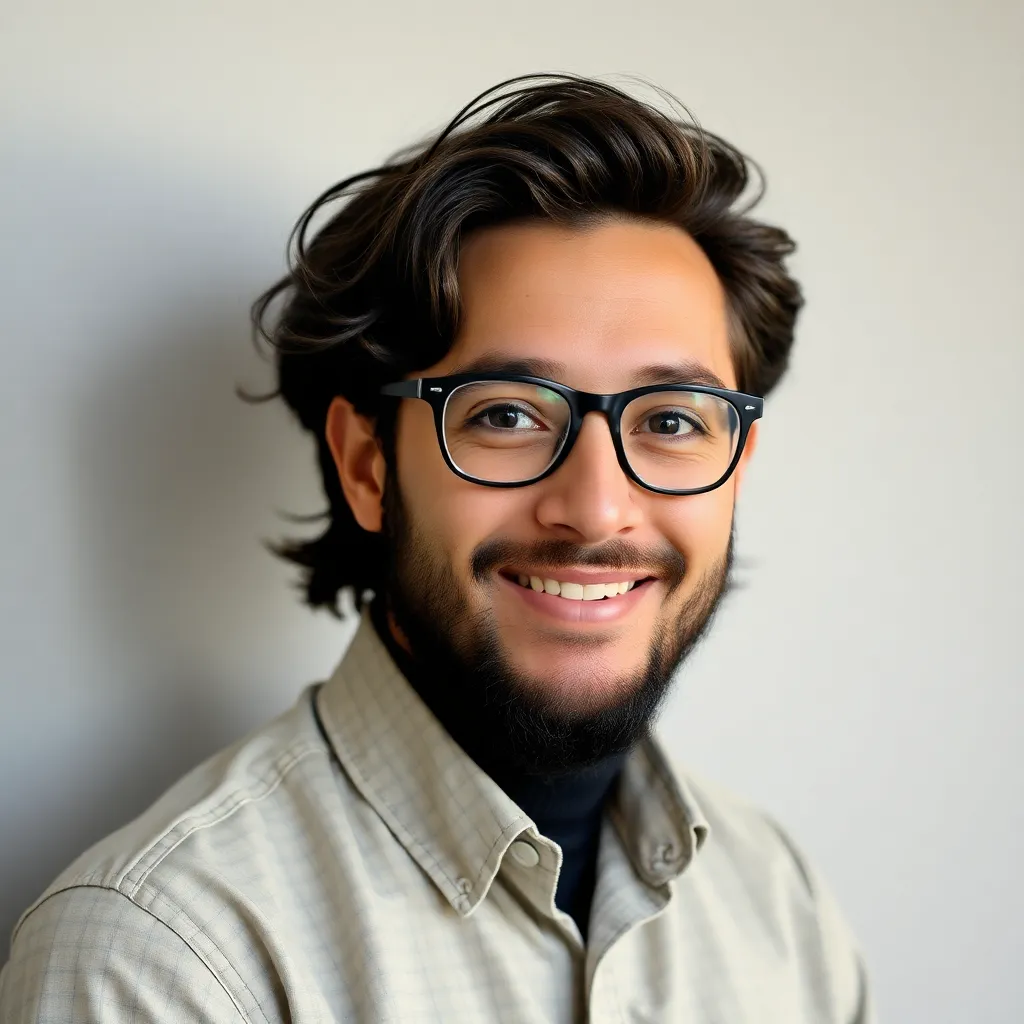
News Leon
Mar 30, 2025 · 6 min read
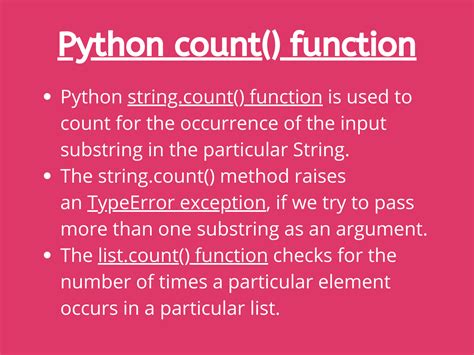
Table of Contents
What Does count()
Do in Python? A Deep Dive into String and List Counting
Python's built-in count()
method is a powerful tool for efficiently determining the frequency of specific items within sequences like strings and lists. Understanding its functionality is crucial for any Python programmer, from beginners tackling basic string manipulation to experienced developers working on complex data analysis projects. This comprehensive guide will explore the count()
method in detail, covering its usage, variations, and practical applications.
Understanding the count()
Method
The count()
method is a string and list method that returns the number of times a specified value appears in a string or a list. It's a straightforward yet versatile function with significant applications in various programming scenarios.
Syntax
The basic syntax of the count()
method is consistent for both strings and lists:
string.count(value, start, end)
list.count(value)
value
: This is the item you want to count. It can be a character (for strings), a number, or any object (for lists).start
(optional, strings only): This specifies the starting index for the search. If omitted, the search begins at index 0.end
(optional, strings only): This specifies the ending index for the search (exclusive). If omitted, the search continues to the end of the string.
Key Differences Between String and List count()
While the core functionality is the same, there's a crucial difference in the optional arguments: strings allow for specifying start and end indices to limit the search area, whereas lists do not offer this functionality. This limitation stems from the fundamental differences in how strings and lists are structured and accessed in Python. Strings are immutable sequences of characters, while lists are mutable sequences of potentially any data type.
Counting Characters in Strings
Let's explore how count()
works with strings. We'll use several examples to illustrate different scenarios.
my_string = "This is a test string. This string is a test."
# Count the occurrences of "is"
count_is = my_string.count("is")
print(f"The substring 'is' appears {count_is} times.") # Output: 2
# Count occurrences of 't'
count_t = my_string.count('t')
print(f"The character 't' appears {count_t} times.") # Output: 5
# Count occurrences of 'T' (case-sensitive)
count_T = my_string.count('T')
print(f"The character 'T' appears {count_T} times.") # Output: 2
# Using start and end indices
count_is_partial = my_string.count("is", 10, 30)
print(f"The substring 'is' appears {count_is_partial} times between indices 10 and 30.") #Output: 1
#Counting Empty Strings
empty_string_count = "hello".count("")
print(f"The number of empty strings in 'hello' is: {empty_string_count}") #Output: 6 (Because an empty string can be found before each character, between each pair of characters and at the end)
These examples demonstrate the case-sensitivity of count()
and the utility of the start
and end
parameters for targeted counting within a specific portion of the string. Remember that count()
is case-sensitive; "Is" and "is" are considered different.
Counting Elements in Lists
The count()
method applied to lists functions similarly, but without the start
and end
parameters.
my_list = [1, 2, 2, 3, 4, 2, 5, 6, 2]
# Count the occurrences of 2
count_2 = my_list.count(2)
print(f"The number 2 appears {count_2} times.") # Output: 4
# Count occurrences of a specific object
my_list_objects = [{"a": 1}, {"a": 1}, {"b": 2}]
count_object = my_list_objects.count({"a": 1})
print(f"The object {{'a': 1}} appears {count_object} times.") # Output: 2
#Counting None values
none_list = [1, None, 2, None, 3, None]
none_count = none_list.count(None)
print(f"The number of None values is: {none_count}") # Output: 3
This shows how count()
efficiently handles various data types within lists. The examples clearly highlight that the count method directly compares objects for equality, not just their values (in the case of dictionaries). Therefore, creating new dictionaries, even with the same key-value pairs, will not be counted as the same object.
Practical Applications of count()
The count()
method finds applications in numerous programming scenarios:
1. Data Analysis and Frequency Distribution:
Determining the frequency of words in a text, analyzing the distribution of numerical values in a dataset, or identifying the most frequent items in a list are common use cases.
text = "This is a sample text. This text is used to demonstrate the count method."
word_counts = {}
words = text.lower().split()
for word in words:
word_counts[word] = word_counts.get(word, 0) + 1
print(word_counts)
This code snippet demonstrates a basic word frequency analysis using count()
implicitly within a loop.
2. String Manipulation and Pattern Matching:
Identifying the number of occurrences of specific substrings within a larger string is vital in tasks like text processing, searching, and data validation.
email = "[email protected];[email protected];[email protected]"
count_emails = email.count('@') # Crude email count for demonstration purposes.
print(f"Approximate number of email addresses: {count_emails}") # Output: 3
3. Log File Analysis:
Analyzing log files to count the occurrences of error messages, specific events, or user actions often utilizes the count()
method for efficient data summarization.
4. Security and Anomaly Detection:
Monitoring system logs for suspicious patterns or unusual frequencies of specific events can involve using count()
to flag potential security threats.
5. Game Development:
Counting occurrences of specific items or events within a game can be useful for tracking player progress, balancing gameplay, or implementing scoring systems.
Advanced Usage and Considerations
While straightforward in its basic application, count()
can be integrated into more sophisticated programming tasks.
Combining with Other Methods:
count()
can be effectively combined with other string or list methods to perform more complex operations. For instance, you can use count()
in conjunction with slicing, splitting, or other methods to achieve more specific counting operations.
Error Handling:
While count()
itself doesn't typically raise exceptions, errors might arise from the data being processed. Proper error handling (e.g., using try-except
blocks) is essential when dealing with potentially problematic input data (like malformed strings or lists).
Performance Considerations:
For extremely large datasets, the performance of count()
might become a concern. In such cases, alternative approaches like using specialized libraries or optimized algorithms might be necessary to maintain efficiency.
Conclusion
Python's count()
method is an indispensable tool for any programmer working with strings and lists. Its simplicity belies its versatility and importance in various programming tasks. By understanding its nuances, including the differences between its application to strings and lists, and its practical applications across various domains, you can significantly enhance the efficiency and effectiveness of your Python code. From basic string manipulation to complex data analysis, mastering the count()
method empowers you to write more efficient, robust, and insightful Python programs. Remember to choose the approach best suited to your data size and computational requirements for optimal performance.
Latest Posts
Latest Posts
-
How Many Parents Are Involved In Asexual Reproduction
Apr 01, 2025
-
What Is The Order Of The Breakdown Products Of Hemoglobin
Apr 01, 2025
-
What Muscle Subdivides The Ventral Body Cavity
Apr 01, 2025
-
How Do You Separate Iron Filings And Sand
Apr 01, 2025
-
How Many Electrons Does F Orbital Hold
Apr 01, 2025
Related Post
Thank you for visiting our website which covers about What Does Count Do In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.