Python Round Number To 2 Decimals
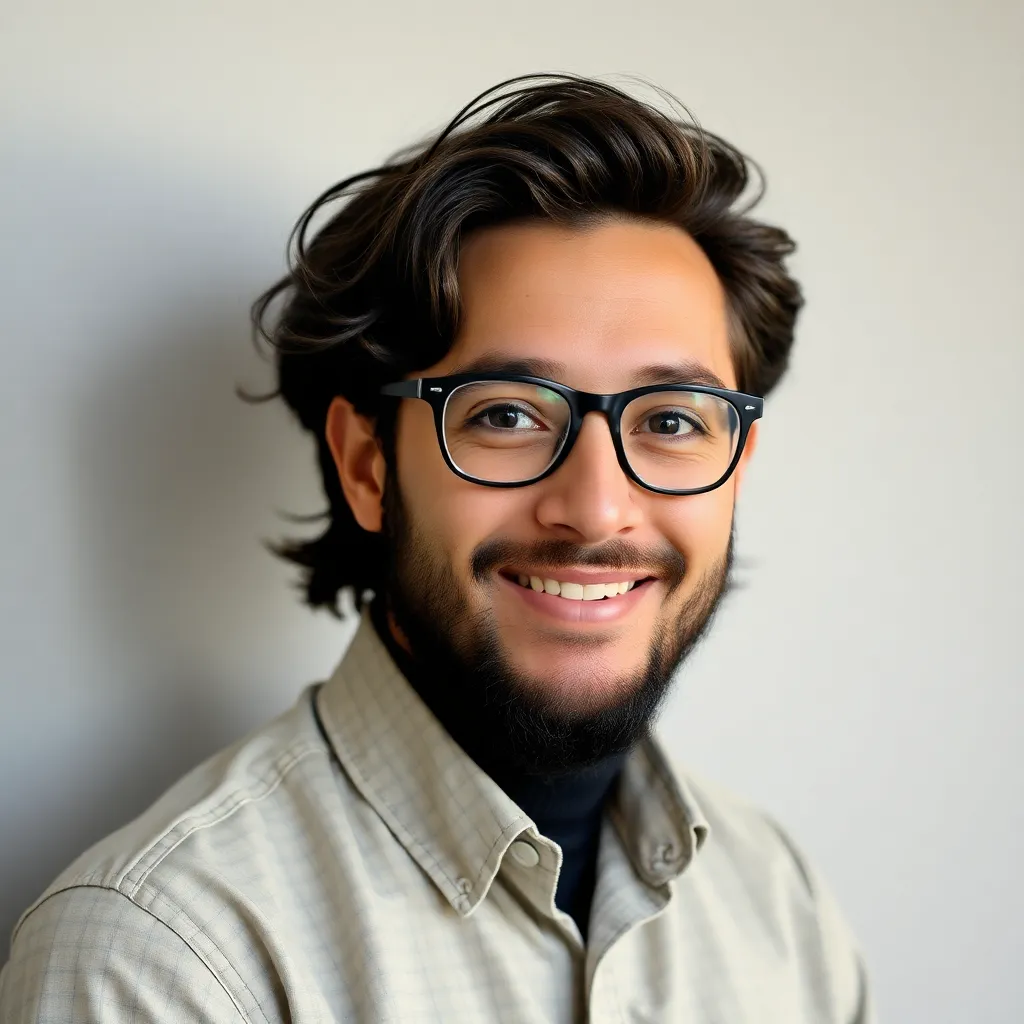
News Leon
Apr 04, 2025 · 5 min read
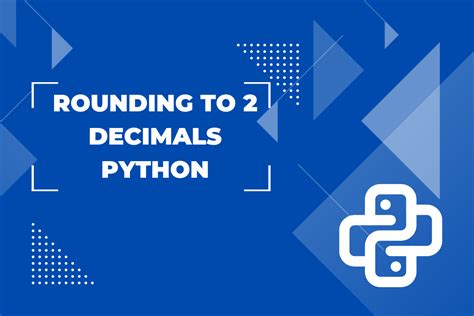
Table of Contents
Python Round Number to 2 Decimals: A Comprehensive Guide
Rounding numbers to two decimal places is a common task in various programming applications, especially when dealing with financial calculations, scientific data, or displaying results to users. Python offers several ways to achieve this, each with its own nuances and advantages. This comprehensive guide will explore different methods, their practical implications, and best practices for ensuring accurate and efficient rounding in your Python projects.
Understanding the Basics of Rounding
Before diving into Python's functionalities, let's clarify what rounding means. Rounding is the process of approximating a number to a certain level of precision. When rounding to two decimal places, we aim to find the closest number with only two digits after the decimal point. The fundamental rule is:
- If the third decimal place is 5 or greater, round up the second decimal place.
- If the third decimal place is less than 5, keep the second decimal place as it is.
For instance:
- 3.14159 becomes 3.14
- 2.71828 becomes 2.72
- 1.995 becomes 2.00
Method 1: Using the round()
function
Python's built-in round()
function is the most straightforward and commonly used method for rounding numbers. It takes two arguments:
- The number to be rounded.
- The number of decimal places (optional, defaults to 0).
number = 3.14159
rounded_number = round(number, 2)
print(rounded_number) # Output: 3.14
number2 = 2.71828
rounded_number2 = round(number2, 2)
print(rounded_number2) # Output: 2.72
number3 = 1.995
rounded_number3 = round(number3,2)
print(rounded_number3) # Output: 2.0
number4 = -1.995
rounded_number4 = round(number4,2)
print(rounded_number4) # Output: -2.0
Important Note on round()
's Behavior with 0.5: The round()
function follows the standard "half to even" rule (also known as banker's rounding). This means that when the number to be rounded ends in exactly 0.5, it will round to the nearest even number.
print(round(2.5)) # Output: 2
print(round(3.5)) # Output: 4
print(round(4.5)) # Output: 4
print(round(-2.5)) # Output: -2
print(round(-3.5)) # Output: -4
print(round(1.50)) # Output: 2
print(round(2.50)) # Output: 2
print(round(0.50)) # Output: 0
This behavior is designed to minimize bias over many rounding operations. While it might seem counterintuitive at first, it ensures fairness in the long run.
Method 2: Using String Formatting
String formatting provides another elegant way to round numbers to a specific number of decimal places. The f-string
method is particularly concise and readable:
number = 3.14159
rounded_number = f"{number:.2f}"
print(rounded_number) # Output: 3.14
number2 = 2.71828
rounded_number2 = f"{number2:.2f}"
print(rounded_number2) # Output: 2.72
number3 = 1.995
rounded_number3 = f"{number3:.2f}"
print(rounded_number3) # Output: 2.00
number4 = -1.995
rounded_number4 = f"{number4:.2f}"
print(rounded_number4) # Output: -2.00
Note that the result of f"{number:.2f}"
is a string, not a floating-point number. If you need to perform further calculations, you'll need to convert it back to a float using float()
.
number = 3.14159
rounded_string = f"{number:.2f}"
rounded_number = float(rounded_string)
print(rounded_number, type(rounded_number)) # Output: 3.14
Method 3: Using the decimal
Module
For applications requiring extremely high precision or where the behavior of round()
might be undesirable, the decimal
module offers a more robust solution. The decimal
module provides a Decimal
class that allows for precise control over rounding and decimal representation.
from decimal import Decimal, ROUND_HALF_UP, ROUND_HALF_EVEN
number = Decimal("3.14159")
rounded_number = number.quantize(Decimal("0.00"), ROUND_HALF_UP)
print(rounded_number) # Output: 3.14
number2 = Decimal("3.14159")
rounded_number2 = number2.quantize(Decimal("0.00"), ROUND_HALF_EVEN)
print(rounded_number2) # Output: 3.14
number3 = Decimal("2.71828")
rounded_number3 = number3.quantize(Decimal("0.00"), ROUND_HALF_UP)
print(rounded_number3) # Output: 2.72
number4 = Decimal("1.995")
rounded_number4 = number4.quantize(Decimal("0.00"), ROUND_HALF_UP)
print(rounded_number4) # Output: 2.00
number5 = Decimal("-1.995")
rounded_number5 = number5.quantize(Decimal("0.00"), ROUND_HALF_UP)
print(rounded_number5) # Output: -2.00
The quantize()
method specifies the precision and rounding mode. ROUND_HALF_UP
rounds 0.5 upwards, while ROUND_HALF_EVEN
implements banker's rounding. Using the decimal
module is particularly advantageous when working with financial data where even minor rounding errors can have significant consequences.
Choosing the Right Method
The best method for rounding to two decimal places depends on your specific needs:
round()
: Ideal for most general-purpose rounding tasks where simplicity and readability are paramount. It's efficient and usually sufficient for common applications.- String Formatting: Useful when you need to directly format a number for display or output without further numerical manipulation. The f-string approach is clean and efficient.
decimal
module: The preferred choice when high precision and fine-grained control over rounding behavior are crucial, particularly in financial or scientific computations where accuracy is paramount.
Handling potential errors and exceptions
While rounding is generally straightforward, it's good practice to consider error handling to prevent unexpected behavior in your code. For instance, if your input isn't a number, you could handle it gracefully with a try-except
block:
def round_to_two_decimals(value):
try:
return round(float(value), 2)
except ValueError:
return "Invalid input: Not a number"
print(round_to_two_decimals(3.14159)) # Output: 3.14
print(round_to_two_decimals("abc")) # Output: Invalid input: Not a number
This robust approach prevents crashes and provides informative feedback to the user. Similarly, you can add input validation to ensure the input meets specific requirements, such as being within a certain range.
Advanced Rounding Scenarios
Sometimes, you might need more control over the rounding process beyond simply specifying the number of decimal places. Here are a few scenarios and how to handle them:
Rounding to significant figures: If you need to round to a specific number of significant figures (rather than decimal places), you'll need a slightly different approach. You might calculate the order of magnitude of the number and adjust the rounding accordingly.
Rounding to the nearest integer: This simplifies to using round(number)
without specifying the number of decimal places.
Custom rounding rules: If you need a rounding rule that deviates from standard practices (e.g., always rounding up or down), you'll need to implement your own custom logic, potentially using conditional statements.
Conclusion
Rounding numbers to two decimal places in Python is a flexible task with several efficient methods. The choice of method depends on the context and your needs, ranging from the simple round()
function for everyday use to the more precise decimal
module for critical applications. Remember to consider error handling and input validation to ensure robustness and prevent unexpected behavior in your code. By mastering these techniques, you'll be well-equipped to handle numerical computations accurately and efficiently in your Python projects. Remember always to prioritize code clarity and maintainability, choosing the method that best suits your specific situation and coding style.
Latest Posts
Latest Posts
-
Costs Which Do Not Change With The Level Of Output
Apr 05, 2025
-
Like The Symmetry Of A Starfish
Apr 05, 2025
-
In All Cases Normative Economics Deals With
Apr 05, 2025
-
Which Statement Is True Of Eukaryotes But Not Of Prokaryotes
Apr 05, 2025
-
The Main Function Of The Entrepreneur Is To
Apr 05, 2025
Related Post
Thank you for visiting our website which covers about Python Round Number To 2 Decimals . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.