Python Check If Character Is Alphanumeric
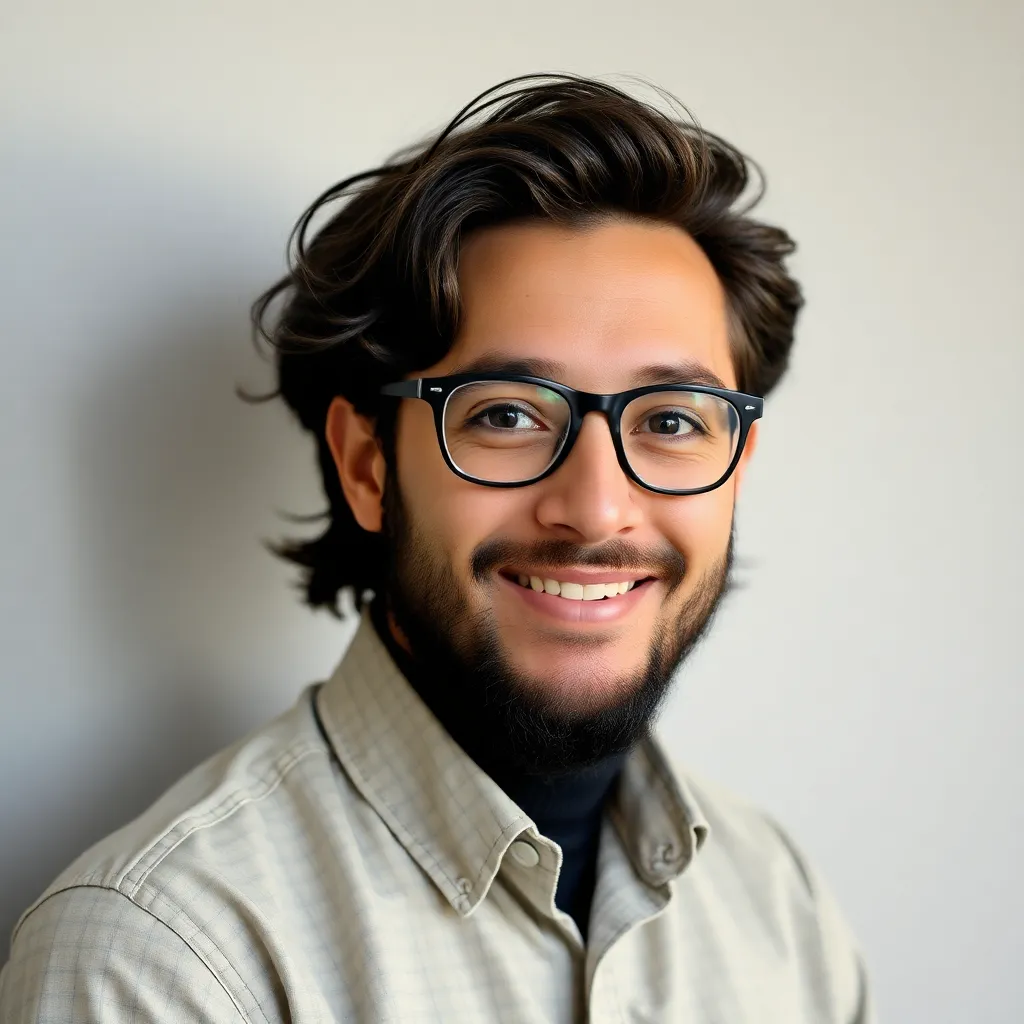
News Leon
Mar 16, 2025 · 5 min read
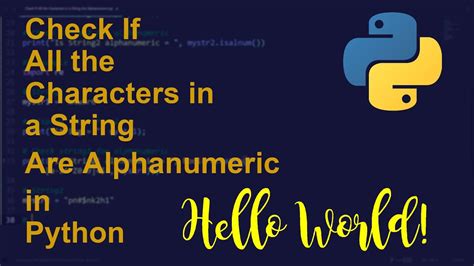
Table of Contents
Python: Checking if a Character is Alphanumeric: A Comprehensive Guide
Python offers several elegant ways to determine if a given character is alphanumeric. This seemingly simple task is crucial in various applications, from data validation and cleaning to password security and natural language processing. This comprehensive guide will delve into the different methods, explore their nuances, and provide practical examples to solidify your understanding. We’ll also touch upon the broader context of character classification in Python and discuss potential pitfalls to avoid.
Understanding Alphanumeric Characters
Before diving into the code, let's define what constitutes an alphanumeric character. An alphanumeric character is simply a character that is either a letter (a-z, A-Z) or a number (0-9). This is a fundamental concept in computer science and forms the basis for many string manipulation tasks.
Method 1: Using the isalnum()
Method
The most straightforward and Pythonic way to check if a character is alphanumeric is by using the built-in isalnum()
string method. This method returns True
if all characters in a string are alphanumeric (letters or numbers), and False
otherwise. It elegantly handles both uppercase and lowercase letters.
character = 'a'
print(f"'{character}' is alphanumeric: {character.isalnum()}") # Output: True
character = 'A'
print(f"'{character}' is alphanumeric: {character.isalnum()}") # Output: True
character = '5'
print(f"'{character}' is alphanumeric: {character.isalnum()}") # Output: True
character = '#'
print(f"'{character}' is alphanumeric: {character.isalnum()}") # Output: False
character = ' '
print(f"'{character}' is alphanumeric: {character.isalnum()}") # Output: False
character = '1a'
print(f"'{character}' is alphanumeric: {character.isalnum()}") # Output: True
character = 'a1!'
print(f"'{character}' is alphanumeric: {character.isalnum()}") # Output: False
Important Note: The isalnum()
method operates on strings. If you're dealing with a single character, ensure it's enclosed in single quotes (' ') to treat it as a string. Attempting to use it on a single character without quotes might lead to a TypeError
.
Method 2: Using ASCII Values (for Advanced Users)
For a deeper understanding, we can explore using ASCII values. Each character has an associated ASCII (American Standard Code for Information Interchange) value. Letters and numbers have specific ranges within the ASCII table. We can leverage this knowledge to check if a character's ASCII value falls within the appropriate range.
def is_alphanumeric_ascii(char):
"""Checks if a character is alphanumeric using ASCII values."""
ascii_val = ord(char)
return (ascii_val >= 48 and ascii_val <= 57) or \
(ascii_val >= 65 and ascii_val <= 90) or \
(ascii_val >= 97 and ascii_val <= 122)
character = 'a'
print(f"'{character}' is alphanumeric: {is_alphanumeric_ascii(character)}") # Output: True
character = 'Z'
print(f"'{character}' is alphanumeric: {is_alphanumeric_ascii(character)}") # Output: True
character = '9'
print(f"'{character}' is alphanumeric: {is_alphanumeric_ascii(character)}") # Output: True
character = '
Latest Posts
Latest Posts
-
How Many Feet Is 1 2 Miles
Mar 18, 2025
-
How Many Valence Electrons Does Mn Have
Mar 18, 2025
-
Lines Of Symmetry On A Trapezoid
Mar 18, 2025
-
Two Same Words With Different Meanings
Mar 18, 2025
-
Select The Correct Statement About Equilibrium
Mar 18, 2025
Related Post
Thank you for visiting our website which covers about Python Check If Character Is Alphanumeric . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.