Python Check If A String Is A Number
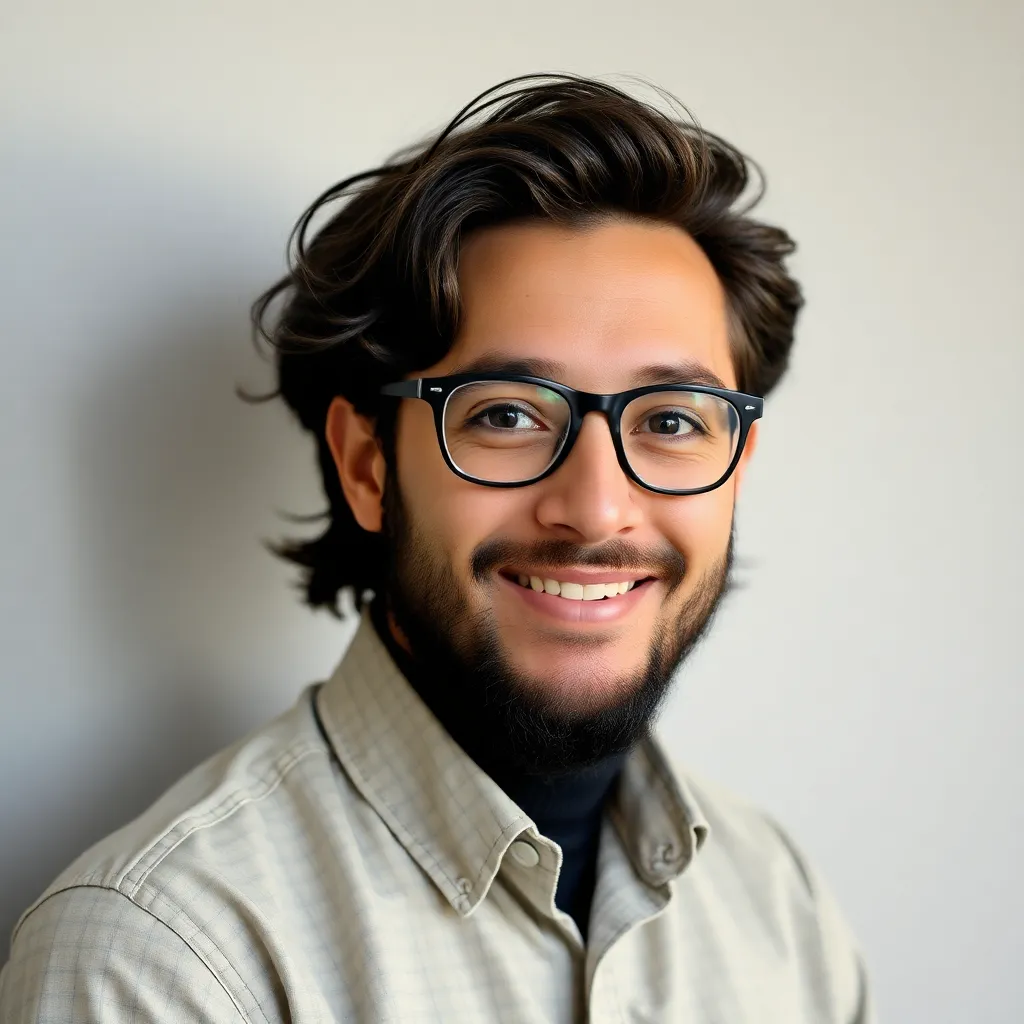
News Leon
Mar 19, 2025 · 5 min read
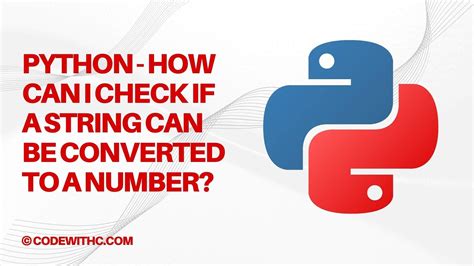
Table of Contents
Python: Checking if a String is a Number: A Comprehensive Guide
Checking if a string represents a number is a common task in Python programming. Whether you're processing user input, reading data from files, or working with external APIs, robustly validating numerical data is crucial to avoid errors and ensure your program's reliability. This comprehensive guide explores various methods to determine if a string can be safely converted to a numeric type in Python, covering both simple and complex scenarios. We'll delve into the intricacies of each approach, highlighting their strengths and weaknesses, and providing practical examples to illustrate their usage.
Understanding the Problem
Before diving into the solutions, let's clarify the challenge. We're not just looking for strings containing digits; we need to account for various number formats, including integers, floating-point numbers, and potentially numbers with different separators or prefixes. A simple string like "123"
is clearly a number, but what about "123.45"
, "+123"
, "-12.3e-2"
, or even "1,234.56"
(with a thousands separator)? Each of these represents a number, but requires a different approach for validation.
Method 1: Using try-except
with Type Conversion
This is arguably the most straightforward and Pythonic method. It leverages Python's exception handling mechanism to attempt the conversion and gracefully handle potential errors.
def is_number(s):
"""
Checks if a string can be converted to a number (int or float).
Args:
s: The input string.
Returns:
True if the string can be converted, False otherwise.
"""
try:
float(s) # Try converting to float; this handles ints and floats
return True
except ValueError:
return False
# Examples
print(is_number("123")) # Output: True
print(is_number("123.45")) # Output: True
print(is_number("-123")) # Output: True
print(is_number("1.23e-2")) # Output: True
print(is_number("abc")) # Output: False
print(is_number("1,234")) # Output: False (thousands separator not handled)
This method is concise, readable, and handles both integer and floating-point numbers. However, it doesn't inherently manage strings with thousands separators or other non-standard formats.
Enhancing the try-except
Method for More Robustness
We can enhance this method to handle more complex scenarios by preprocessing the string:
import re
def is_number_enhanced(s):
"""
Improved version handling thousands separators and signs.
"""
s = s.replace(",", "") #remove thousands separator
try:
float(s)
return True
except ValueError:
return False
#Example
print(is_number_enhanced("1,234.56")) # Output: True
This improved version removes commas before attempting conversion, making it more flexible. However, it still might fail with more exotic number formats.
Method 2: Using Regular Expressions
For more complex validation, regular expressions provide a powerful tool. You can define a pattern that matches the expected format of your numerical strings.
import re
def is_number_regex(s):
"""
Checks if a string matches a number pattern using regular expressions. This example covers a wide range of number formats but can be adjusted based on specific needs.
"""
pattern = r"^[-+]?\d*\.?\d+(?:[eE][-+]?\d+)?$" # Matches integers, floats, and scientific notation
if re.match(pattern, s):
return True
else:
return False
# Examples
print(is_number_regex("123")) # Output: True
print(is_number_regex("123.45")) # Output: True
print(is_number_regex("-123")) # Output: True
print(is_number_regex("1.23e-2")) # Output: True
print(is_number_regex("abc")) # Output: False
print(is_number_regex("1,234")) # Output: False
print(is_number_regex("+123.45")) #Output: True
This approach allows for precise control over the accepted number formats. However, crafting a comprehensive regular expression can be complex and error-prone, particularly if you need to support a wide variety of formats.
Method 3: Leveraging isdigit()
(for Integer Strings Only)
The isdigit()
method is a simple way to check if a string consists only of digits. However, it's limited to positive integers and doesn't handle decimals, negative signs, or scientific notation.
def is_digit_string(s):
"""
Checks if a string contains only digits (positive integers).
"""
return s.isdigit()
#Examples
print(is_digit_string("123")) #Output: True
print(is_digit_string("123.45")) #Output: False
print(is_digit_string("-123")) #Output: False
This method is useful only for the most basic case of positive integer strings.
Method 4: Custom Functions for Specific Formats
For highly specialized needs, consider creating custom functions tailored to your specific number formats. This provides maximum control but requires more coding effort.
def is_integer_with_thousands_separator(s):
"""Checks for integers with thousands separators (e.g., 1,234,567)."""
s = s.replace(",", "")
try:
int(s)
return True
except ValueError:
return False
#Example
print(is_integer_with_thousands_separator("1,234,567")) #Output: True
print(is_integer_with_thousands_separator("1,234.567")) #Output: False
def is_currency(s):
"""Checks for currency format (e.g., $123.45, €123,45)."""
#A more complex regex would be needed here to account for various currency symbols and formats
pass #Implementation would require a robust regex pattern.
This approach allows for fine-grained control but demands more development time and testing.
Choosing the Right Method
The best method depends on your specific requirements:
-
Simple integer and float validation: The
try-except
method (Method 1) offers a good balance of simplicity, readability, and effectiveness for most common scenarios. The enhanced version addresses many common variations. -
Complex number formats and stringent validation: Regular expressions (Method 2) provide the most flexibility and precision but require more expertise to use correctly.
-
Positive integer strings only:
isdigit()
(Method 3) is the simplest approach for this limited case. -
Highly specialized number formats: Custom functions (Method 4) offer maximum control but demand more development effort.
Error Handling and User Experience
Regardless of the chosen method, robust error handling is essential. Always include appropriate try-except
blocks to gracefully handle cases where the input string is not a valid number. Provide clear and informative error messages to the user if necessary.
Beyond Basic Number Checking
The techniques discussed here form the foundation for more advanced numerical data validation. You might need to incorporate additional checks for:
- Range validation: Ensure the number falls within a specific range.
- Data type validation: Verify that the number is of the correct type (integer, float, etc.).
- Precision validation: Check the number of decimal places.
- Format validation: Enforce specific formatting rules (e.g., currency format).
By combining these basic methods with additional checks and careful error handling, you can create robust and reliable number validation routines in your Python programs. Remember to always prioritize clear code, informative error messages, and user-friendly interfaces. Choosing the optimal method depends heavily on the context of your application and the level of string validation complexity required. Prioritize clarity and maintainability in your code while balancing the need for accuracy and robustness in your number validation process.
Latest Posts
Latest Posts
-
What Are The Differences Between Political Parties And Interest Groups
Mar 19, 2025
-
Only Moveable Bone In The Skull
Mar 19, 2025
-
In Which Stage Of Meiosis Is The Chromosome Number Halved
Mar 19, 2025
-
The Electric Potential Difference Between The Ground And A Cloud
Mar 19, 2025
-
What Percent Is 3 Of 5
Mar 19, 2025
Related Post
Thank you for visiting our website which covers about Python Check If A String Is A Number . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.