How To Use Summation In Python
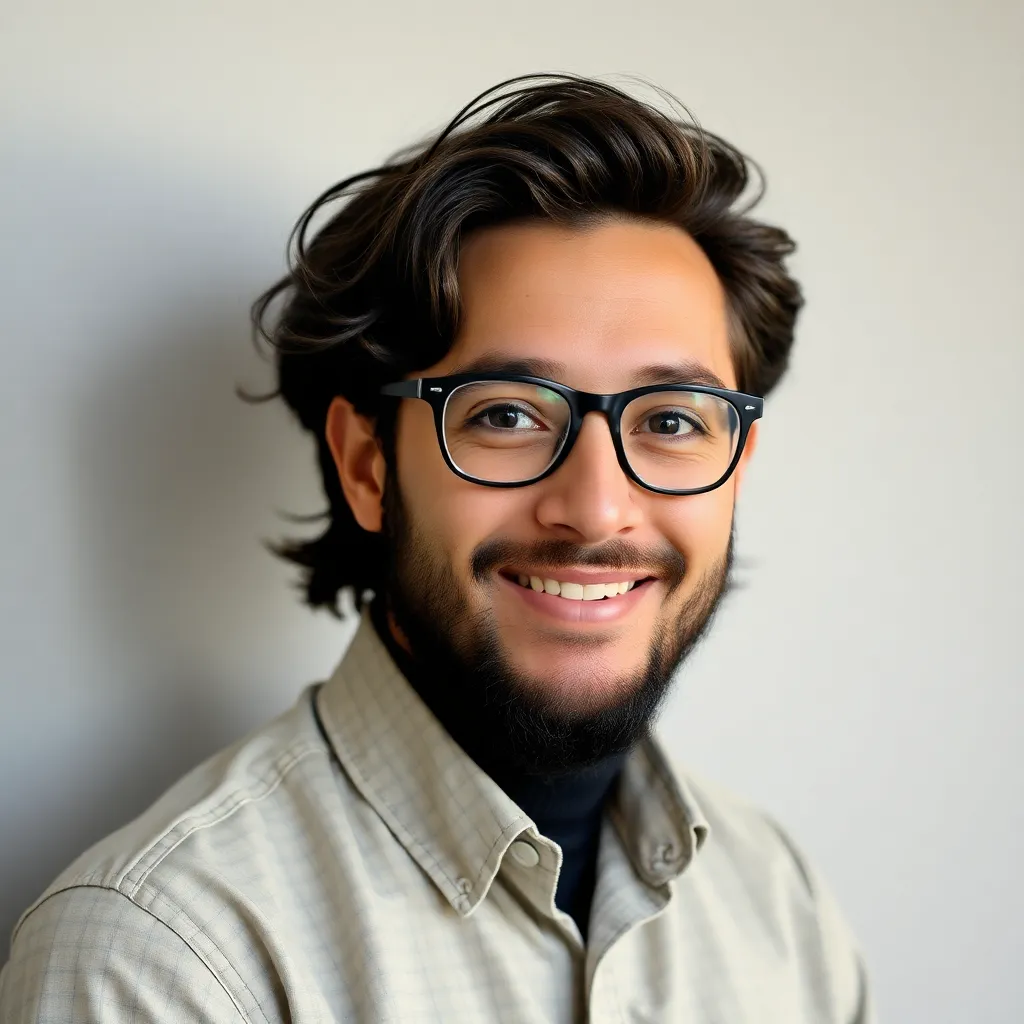
News Leon
Apr 18, 2025 · 6 min read

Table of Contents
Mastering Summation in Python: A Comprehensive Guide
Summation, the process of adding a sequence of numbers, is a fundamental operation in mathematics and programming. Python, with its rich libraries and flexible syntax, offers several efficient ways to perform summation. This comprehensive guide will explore various techniques, from basic loops to leveraging powerful libraries like NumPy, demonstrating how to handle different types of summations and optimizing your code for speed and readability. We'll cover everything from simple arithmetic series to more complex scenarios involving conditional summations and custom functions.
Understanding Summation
Before diving into Python implementations, let's briefly review the mathematical concept of summation. Summation is represented using the sigma notation (Σ). A typical summation looks like this:
Σ<sub>i=1</sub><sup>n</sup> f(i)
This notation signifies the sum of f(i) for all values of 'i' ranging from 1 to n. 'i' is the index variable, '1' is the lower limit, 'n' is the upper limit, and f(i) is the function or expression being summed.
Basic Summation in Python using Loops
The most straightforward approach to summation in Python is using a for
loop. This method is highly versatile and easily understandable, especially for beginners.
Example 1: Summing a sequence of numbers
Let's say we want to sum the numbers from 1 to 10:
total = 0
for i in range(1, 11):
total += i
print(f"The sum of numbers from 1 to 10 is: {total}")
This code initializes a variable total
to 0. The loop iterates through numbers 1 to 10, adding each number to total
. The final total
holds the sum.
Example 2: Summing elements of a list
Suppose we have a list of numbers:
numbers = [15, 20, 25, 30, 35]
total = 0
for number in numbers:
total += number
print(f"The sum of the numbers in the list is: {total}")
This loop iterates through each element in the numbers
list, accumulating the sum in total
. This method works for any iterable, including tuples and sets.
Using the sum()
function
Python's built-in sum()
function provides a more concise and efficient way to sum the elements of an iterable:
numbers = [15, 20, 25, 30, 35]
total = sum(numbers)
print(f"The sum of the numbers in the list is: {total}")
The sum()
function directly calculates the sum, eliminating the need for explicit looping. This improves readability and often performance, especially for large datasets.
Summation with Conditional Logic
Often, you'll need to sum only specific elements based on certain conditions. This can be easily achieved by incorporating conditional statements within the loop or using list comprehensions.
Example 3: Summing even numbers
Let's sum only the even numbers from 1 to 20:
total = 0
for i in range(1, 21):
if i % 2 == 0:
total += i
print(f"The sum of even numbers from 1 to 20 is: {total}")
Here, the if
statement filters out odd numbers, only adding even numbers to total
.
Example 4: Summation using List Comprehension
List comprehensions provide a compact way to achieve the same result:
numbers = list(range(1, 21))
even_sum = sum([i for i in numbers if i % 2 == 0])
print(f"The sum of even numbers from 1 to 20 is: {even_sum}")
This single line of code creates a list containing only even numbers and then sums them using sum()
.
Advanced Summation Techniques with NumPy
For numerical computations involving large datasets, NumPy is a game-changer. Its vectorized operations significantly boost performance compared to loop-based approaches.
Example 5: Summing a NumPy array
import numpy as np
numbers = np.array([15, 20, 25, 30, 35])
total = np.sum(numbers)
print(f"The sum of the numbers in the NumPy array is: {total}")
NumPy's np.sum()
function directly operates on the entire array, resulting in much faster computation, especially for large arrays.
Example 6: Summing along specific axes in multi-dimensional arrays
NumPy's power truly shines when dealing with multi-dimensional arrays. The axis
parameter in np.sum()
allows you to control which dimension is summed along.
import numpy as np
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
row_sums = np.sum(matrix, axis=1) # Sum along rows
column_sums = np.sum(matrix, axis=0) # Sum along columns
print(f"Row sums: {row_sums}")
print(f"Column sums: {column_sums}")
This demonstrates how easily you can compute row-wise and column-wise sums using NumPy.
Summation of Series using Mathematical Formulas
For specific types of series (arithmetic, geometric, etc.), using the corresponding mathematical formulas is often more efficient than iterative methods.
Example 7: Sum of an Arithmetic Series
The sum of an arithmetic series can be calculated using the formula: S = n/2 * (2a + (n-1)d), where 'n' is the number of terms, 'a' is the first term, and 'd' is the common difference.
def arithmetic_series_sum(n, a, d):
"""Calculates the sum of an arithmetic series."""
return n / 2 * (2 * a + (n - 1) * d)
n = 10 # Number of terms
a = 1 # First term
d = 1 # Common difference
sum_arithmetic = arithmetic_series_sum(n, a, d)
print(f"Sum of arithmetic series: {sum_arithmetic}")
Example 8: Sum of a Geometric Series
The sum of a geometric series is given by: S = a(1 - r^n) / (1 - r), where 'a' is the first term, 'r' is the common ratio, and 'n' is the number of terms.
def geometric_series_sum(a, r, n):
"""Calculates the sum of a geometric series."""
if abs(r) >= 1 and n > 0:
raise ValueError("Sum diverges for |r| >= 1 and n > 0")
return a * (1 - r**n) / (1 - r)
a = 1 # First term
r = 0.5 # Common ratio
n = 5 # Number of terms
sum_geometric = geometric_series_sum(a, r, n)
print(f"Sum of geometric series: {sum_geometric}")
Handling Complex Summations
More complex summation problems might involve nested loops, recursive functions, or custom functions within the summation.
Example 9: Double Summation
A double summation involves summing over two indices. This can be implemented using nested loops:
total = 0
for i in range(1, 3):
for j in range(1, 3):
total += i * j
print(f"Double summation result: {total}")
Example 10: Summation with a Custom Function
You might need to sum the results of a custom function applied to each element:
def my_function(x):
return x**2
numbers = [1, 2, 3, 4, 5]
sum_of_squares = sum(my_function(x) for x in numbers)
print(f"Sum of squares: {sum_of_squares}")
Optimization Strategies for Large Datasets
For extremely large datasets, optimizing your summation process is crucial. Here are some key strategies:
- Vectorization with NumPy: Always prioritize NumPy's vectorized operations for significant performance gains.
- Efficient Data Structures: Choose appropriate data structures (e.g., NumPy arrays) that are optimized for numerical computations.
- Parallel Processing: For massive datasets, consider using parallel processing libraries like multiprocessing or Dask to distribute the summation across multiple cores.
Conclusion
Python provides a variety of tools and techniques for performing summations, from simple loops to powerful libraries like NumPy. The best approach depends on the complexity of your summation problem and the size of your data. Understanding these methods empowers you to write efficient and readable code for a wide range of summation tasks. Remember to consider optimization strategies when dealing with large datasets to ensure your code remains performant. Mastering summation is a crucial step in your journey towards advanced programming and data analysis.
Latest Posts
Latest Posts
-
Identify The Following Salts As Acidic Basic Or Neutral
Apr 19, 2025
-
Which Of The Following Is Not A Consumer
Apr 19, 2025
-
Which Of The Following Is Not An Optical Storage Device
Apr 19, 2025
-
The Mean Of A Sample Is
Apr 19, 2025
-
Which Of The Following Are Manufactured By Microbial Fermentation
Apr 19, 2025
Related Post
Thank you for visiting our website which covers about How To Use Summation In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.