How To Use Sum In Python
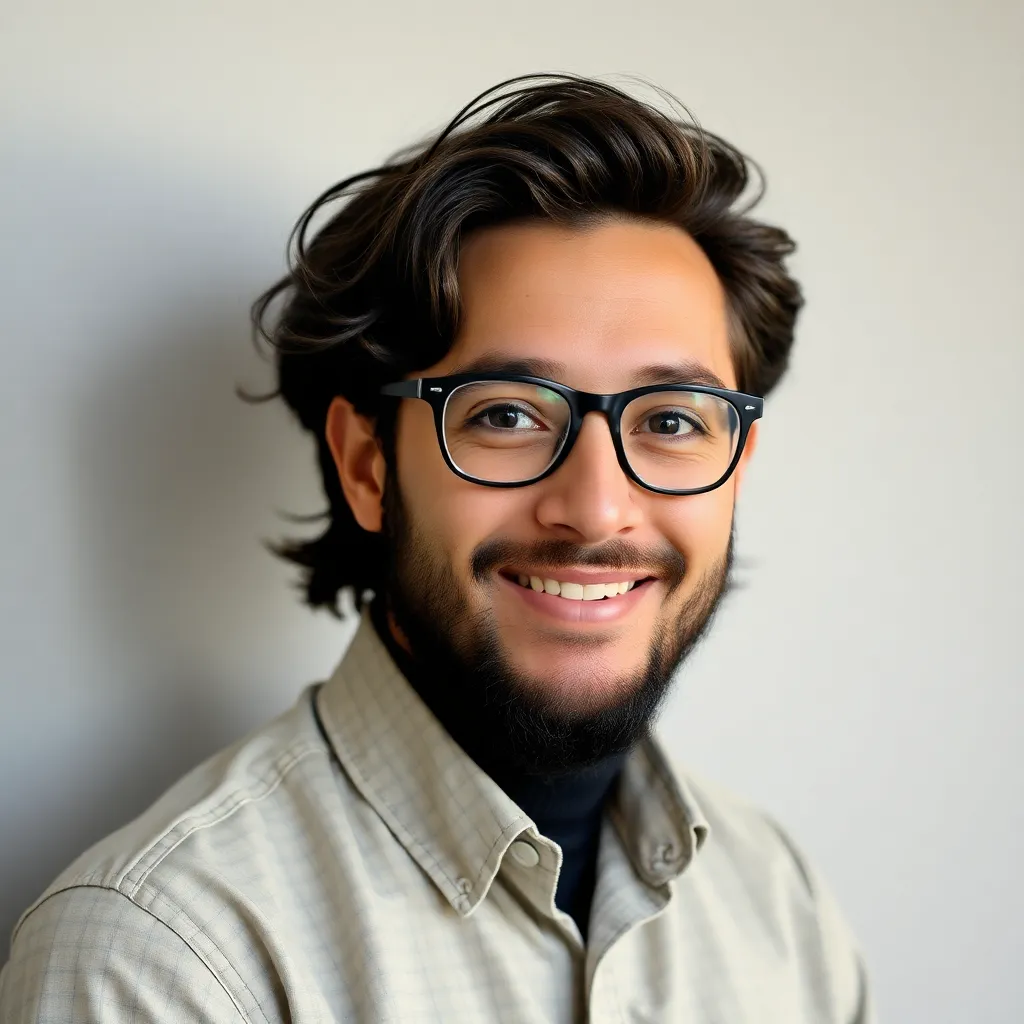
News Leon
Mar 24, 2025 · 5 min read
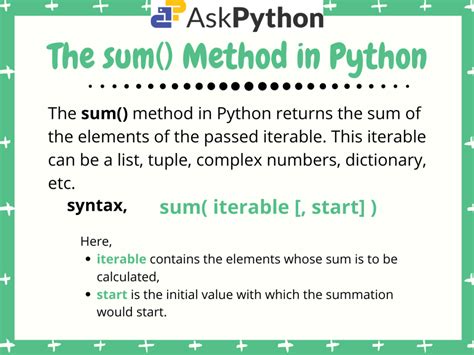
Table of Contents
Mastering the sum()
Function in Python: A Comprehensive Guide
Python's built-in sum()
function is a powerful and versatile tool for performing arithmetic operations on iterable objects. While seemingly simple at first glance, understanding its nuances and applications unlocks a wide array of possibilities for data manipulation and analysis. This comprehensive guide delves into the intricacies of sum()
, exploring its core functionality, advanced usage scenarios, and potential pitfalls to avoid.
Understanding the Basics of sum()
At its core, the sum()
function calculates the total of all numerical elements within an iterable, such as a list, tuple, or set. The syntax is straightforward:
sum(iterable, start=0)
iterable
: This argument is mandatory. It represents the sequence of numbers you want to sum. This could be a list ([1, 2, 3]
), a tuple ((1, 2, 3)
), a set ({1, 2, 3}
), or any other object that supports iteration and yields numerical values.start
: This argument is optional. It specifies a value to add to the sum before iterating through the iterable. By default,start
is 0.
Example 1: Basic Summation
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(f"The sum is: {total}") # Output: The sum is: 15
Example 2: Using the start
Parameter
numbers = [1, 2, 3, 4, 5]
total = sum(numbers, start=10)
print(f"The sum is: {total}") # Output: The sum is: 25
Handling Different Data Types
While sum()
primarily works with numerical data, understanding how it handles different numerical types is crucial.
Example 3: Mixing Integer and Float Types
mixed_numbers = [1, 2.5, 3, 4.7, 5]
total = sum(mixed_numbers)
print(f"The sum is: {total}") # Output: The sum is: 16.2
Python seamlessly handles the combination of integers and floats, automatically promoting the result to a float if necessary to maintain precision.
Example 4: Error Handling with Non-Numerical Data
mixed_data = [1, 2, 'a', 4, 5]
try:
total = sum(mixed_data)
print(f"The sum is: {total}")
except TypeError as e:
print(f"Error: {e}") # Output: Error: unsupported operand type(s) for +: 'int' and 'str'
Attempting to sum an iterable containing non-numerical elements (like strings) will result in a TypeError
. Robust code should include error handling (like the try-except
block above) to gracefully manage such situations.
Advanced Applications of sum()
Beyond simple summations, sum()
can be used in conjunction with other Python features to achieve sophisticated data processing.
1. Summing with List Comprehension
List comprehension provides a concise way to generate new lists based on existing ones. Combining this with sum()
allows for efficient calculations on filtered or transformed data.
Example 5: Summing Even Numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_sum = sum([num for num in numbers if num % 2 == 0])
print(f"The sum of even numbers is: {even_sum}") # Output: The sum of even numbers is: 30
2. Summing Elements from Nested Iterables
sum()
can effectively handle nested iterables, although it requires careful consideration of how you structure your summation.
Example 6: Summing Numbers in a Nested List
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = sum(sum(inner_list) for inner_list in nested_list)
print(f"The sum of all numbers is: {total}") # Output: The sum of all numbers is: 45
#Alternative using nested list comprehension
total = sum([sum(inner_list) for inner_list in nested_list])
print(f"The sum of all numbers (list comprehension): {total}") # Output: The sum of all numbers (list comprehension): 45
This example uses nested list comprehension to efficiently flatten the nested list before summation.
3. Custom Summation with lambda
Functions
lambda
functions, or anonymous functions, can be combined with sum()
to perform more complex calculations during the summation process.
Example 7: Summing Squares
numbers = [1, 2, 3, 4, 5]
sum_of_squares = sum(map(lambda x: x**2, numbers))
print(f"The sum of squares is: {sum_of_squares}") # Output: The sum of squares is: 55
Here, a lambda
function squares each number before it's added to the total. map
applies the lambda function to each element in the list.
4. Working with NumPy Arrays
For numerical computation on large datasets, NumPy arrays offer significant performance advantages. sum()
works seamlessly with NumPy arrays.
Example 8: Summing a NumPy Array
import numpy as np
array = np.array([1, 2, 3, 4, 5])
total = np.sum(array) # Or sum(array) - both work
print(f"The sum is: {total}") # Output: The sum is: 15
NumPy's np.sum()
function is often preferred for its optimized performance, especially when dealing with very large arrays. However, Python's built-in sum()
also functions correctly.
Potential Pitfalls and Best Practices
While sum()
is generally straightforward, some potential issues warrant attention.
- Type Errors: Always ensure your iterable contains only numerical data. Pre-processing your data to handle potential non-numerical elements can prevent runtime errors.
- Large Datasets: For extremely large datasets, consider using NumPy or other optimized libraries for better performance. Python's built-in
sum()
might become slow for datasets with millions of elements. - Mutable Iterables: Avoid modifying the iterable while it's being summed. This can lead to unpredictable results.
- Clarity and Readability: For complex summations involving multiple operations or transformations, consider breaking down the process into smaller, more readable steps instead of trying to cram everything into a single
sum()
call with a complex lambda function.
Conclusion
Python's sum()
function is a fundamental tool for any programmer working with numerical data. Understanding its basic usage, exploring its advanced applications with list comprehensions, lambda functions, and NumPy arrays, and being mindful of potential pitfalls enables you to write efficient and robust Python code for a wide range of data processing tasks. Mastering sum()
empowers you to write more concise, readable, and performant code for your numerical computations. Remember to choose the most appropriate approach—whether it's the built-in sum()
function or NumPy's np.sum()
—based on the size and nature of your dataset and performance requirements.
Latest Posts
Latest Posts
-
Correctly Label The Following Parts Of The Testis
Mar 26, 2025
-
Which Of The Following Equations Is True
Mar 26, 2025
-
The Molar Mass Of Cuso4 5h2o Is 249
Mar 26, 2025
-
Enzymes Belong To Which Group Of Macromolecules
Mar 26, 2025
-
A Word On A Web Page That When Clicked
Mar 26, 2025
Related Post
Thank you for visiting our website which covers about How To Use Sum In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.