How To Round Numbers In Python
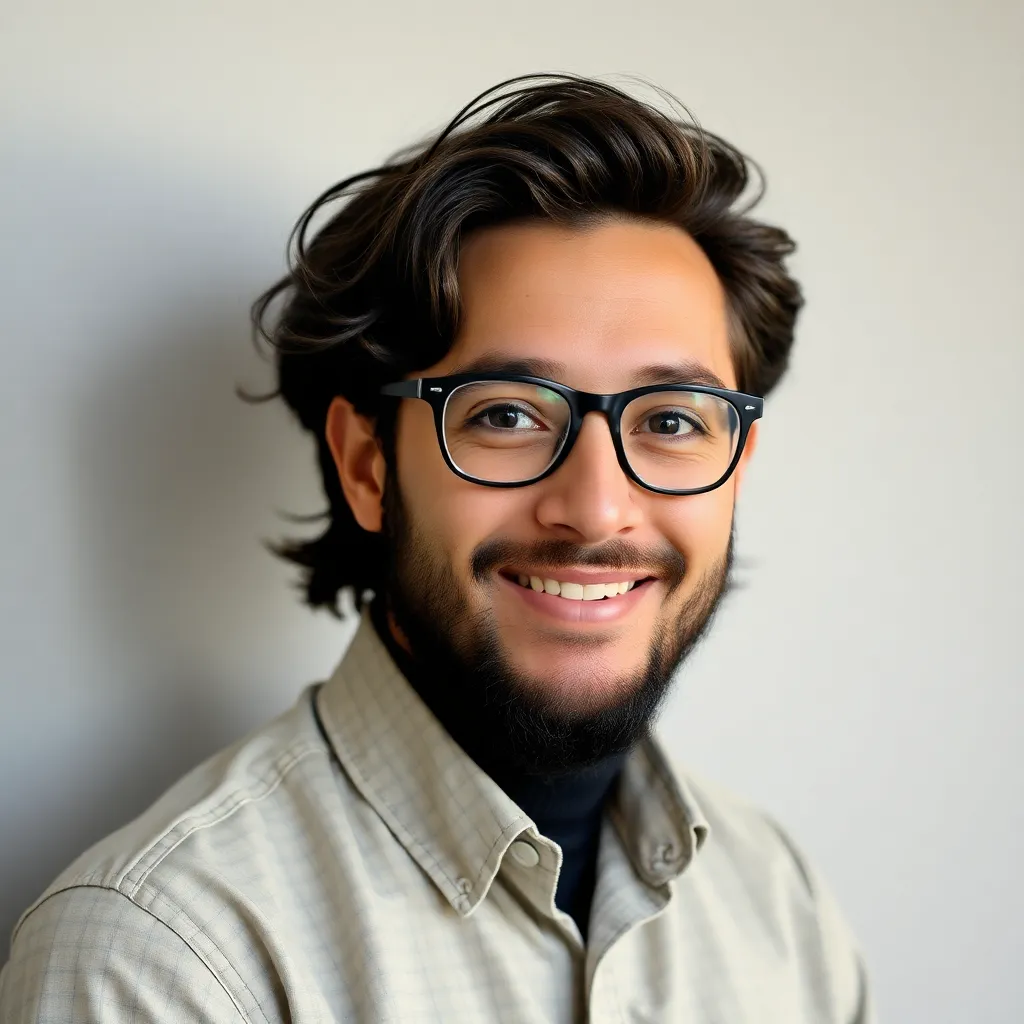
News Leon
Mar 19, 2025 · 5 min read
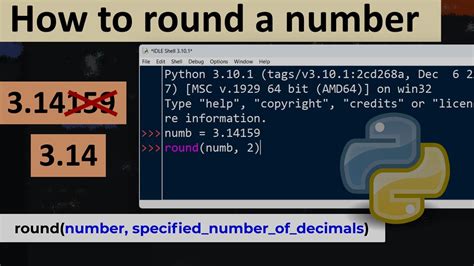
Table of Contents
Mastering the Art of Rounding Numbers in Python
Rounding numbers is a fundamental operation in various programming tasks, from data analysis and scientific computing to financial applications and general-purpose programming. Python, with its rich set of built-in functions and libraries, offers several ways to round numbers to varying degrees of precision. Understanding these methods and choosing the appropriate technique is crucial for accurate and efficient code. This comprehensive guide dives deep into the intricacies of rounding in Python, exploring different approaches and scenarios to solidify your understanding.
Understanding Rounding Fundamentals
Before delving into Python's specific functionalities, let's revisit the basic principles of rounding. The goal is to approximate a number to a certain level of precision, typically to a specified number of decimal places or significant figures. The most common rounding method is round-to-nearest, where a number is rounded to the nearest integer or decimal value. If the fractional part is exactly 0.5, the tie-breaker rules vary, and we'll explore those variations within Python's context.
Python's Built-in round()
Function
Python's built-in round()
function is the primary tool for rounding numbers. It's straightforward to use and handles most common rounding scenarios effectively.
Basic Usage
The round()
function takes two arguments:
- number: The number to be rounded. This can be an integer or a floating-point number.
- ndigits (optional): The number of decimal places to round to. If omitted, the number is rounded to the nearest integer.
# Rounding to the nearest integer
x = 3.14
rounded_x = round(x) # rounded_x will be 3
y = 7.99
rounded_y = round(y) # rounded_y will be 8
# Rounding to a specific number of decimal places
z = 3.14159
rounded_z = round(z, 2) # rounded_z will be 3.14
a = 12.5
rounded_a = round(a,0) #rounded_a will be 12.0
b = 12.5
rounded_b = round(b) #rounded_b will be 13
c = 12.50000001
rounded_c = round(c) #rounded_c will be 13
d = -2.5
rounded_d = round(d) #rounded_d will be -2
Tie-Breaker Rules
The round()
function employs a "round half to even" strategy, also known as banker's rounding. This means that when the fractional part is exactly 0.5, the number is rounded to the nearest even integer. This approach minimizes bias over many rounding operations.
x = 2.5
rounded_x = round(x) # rounded_x will be 2 (even)
y = 3.5
rounded_y = round(y) # rounded_y will be 4 (even)
z = 1.5
rounded_z = round(z,0) #rounded_z will be 2.0 (even)
a = -1.5
rounded_a = round(a,0) #rounded_a will be -2.0 (even)
This is different from the common "round half up" method, where 0.5 is always rounded up. While banker's rounding might seem unusual at first, it leads to more statistically accurate results in the long run.
Rounding to Significant Figures
The round()
function directly rounds to a specified number of decimal places. However, sometimes you need to round to a specific number of significant figures. This requires a slightly more involved approach.
import math
def round_to_sigfigs(num, sigfigs):
"""Rounds a number to a specified number of significant figures."""
if num == 0:
return 0
order = math.floor(math.log10(abs(num)))
rounded_num = round(num * (10**(-order)), sigfigs -1)
return rounded_num * (10**order)
# Example
num = 3.14159
rounded_num = round_to_sigfigs(num, 3) # rounded_num will be 3.14
num = 123456.789
rounded_num = round_to_sigfigs(num, 2) # rounded_num will be 120000.0
num = 0.00012345
rounded_num = round_to_sigfigs(num, 2) # rounded_num will be 0.00012
This round_to_sigfigs
function determines the order of magnitude, scales the number accordingly, performs rounding using round()
, and then scales back to the original order of magnitude. This ensures that rounding is performed with respect to significant figures rather than decimal places.
Handling Large and Small Numbers
When dealing with extremely large or small numbers in scientific notation, standard rounding might lead to unexpected results due to floating-point limitations. In such cases, consider using the decimal
module for higher precision.
from decimal import Decimal, ROUND_HALF_UP
num = Decimal("1.2345e+20")
rounded_num = num.quantize(Decimal("1e+18"), ROUND_HALF_UP) #rounding to 2 significant figures
print(rounded_num)
num = Decimal("1.2345e-20")
rounded_num = num.quantize(Decimal("1e-22"), ROUND_HALF_UP) #rounding to 2 significant figures
print(rounded_num)
The decimal
module provides arbitrary-precision decimal arithmetic, mitigating potential inaccuracies inherent in floating-point representation. The quantize()
method allows for precise rounding based on a specified scale.
Rounding to Nearest Multiple
Often, you might need to round a number to the nearest multiple of a specific value (e.g., rounding to the nearest 5, 10, or 100). A simple approach involves using the round()
function in conjunction with division and multiplication.
def round_to_nearest_multiple(num, multiple):
"""Rounds a number to the nearest multiple of a given value."""
remainder = num % multiple
if remainder >= multiple / 2:
return num + (multiple - remainder)
else:
return num - remainder
# Example: Round to the nearest 5
num = 23
rounded_num = round_to_nearest_multiple(num, 5) # rounded_num will be 25
num = 17
rounded_num = round_to_nearest_multiple(num, 5) # rounded_num will be 15
num = 22.5
rounded_num = round_to_nearest_multiple(num, 5) #rounded_num will be 25
num = -22.5
rounded_num = round_to_nearest_multiple(num, 5) #rounded_num will be -20
This function calculates the remainder after division by the specified multiple. If the remainder is greater than or equal to half the multiple, it rounds up; otherwise, it rounds down.
Choosing the Right Rounding Method
The selection of the appropriate rounding method depends heavily on the context of your application. Consider the following factors:
- Precision Requirements: For applications requiring high precision, such as financial calculations or scientific simulations, consider using the
decimal
module. - Rounding Rules: Choose between "round half to even" (banker's rounding) or "round half up" based on your specific needs and statistical implications.
- Significant Figures vs. Decimal Places: Ensure you're using the correct rounding approach based on whether you need to round to a fixed number of decimal places or significant figures.
- Performance Considerations: For simple rounding tasks, the built-in
round()
function is highly efficient. For more complex scenarios or high-precision calculations, the trade-off between precision and performance might need to be carefully evaluated.
Conclusion
Mastering the art of rounding in Python is essential for writing robust and accurate code. Python provides a range of tools to handle various rounding scenarios, from simple integer rounding to advanced, high-precision decimal arithmetic. By understanding the nuances of each approach and carefully considering your specific requirements, you can ensure your programs handle numerical data with precision and efficiency. Remember to always prioritize selecting the rounding method that best suits the context of your task, balancing the need for accuracy with computational efficiency. This comprehensive guide has equipped you with the knowledge to confidently tackle various rounding challenges in your Python projects. Happy coding!
Latest Posts
Latest Posts
-
What Is 8 Percent As A Decimal
Mar 19, 2025
-
What Is Conjugate Base Of Hso4
Mar 19, 2025
-
55 Miles Per Hour To Meters Per Second
Mar 19, 2025
-
The Twinkling Of Stars Is Caused By
Mar 19, 2025
-
Literary Elements In The Road Not Taken
Mar 19, 2025
Related Post
Thank you for visiting our website which covers about How To Round Numbers In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.