How To Initialize A Tuple In Python
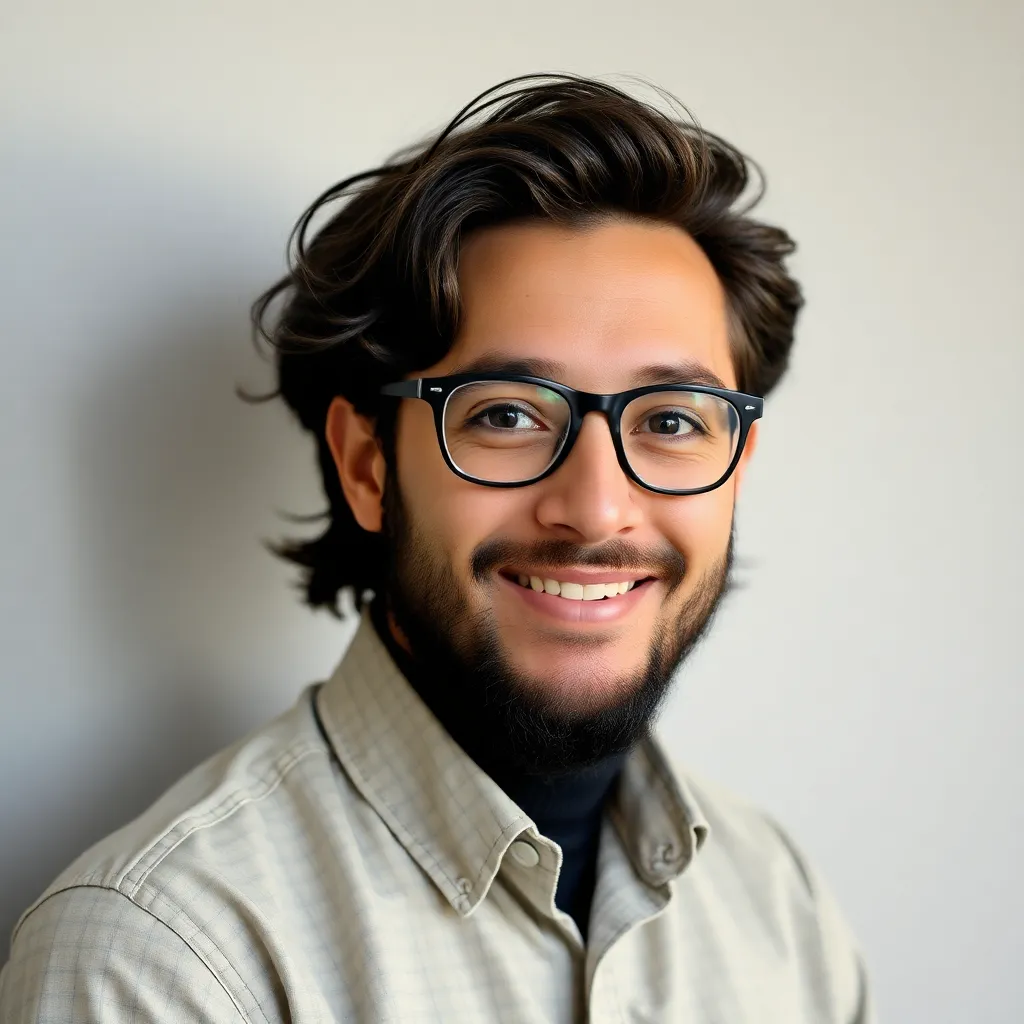
News Leon
Apr 02, 2025 · 6 min read
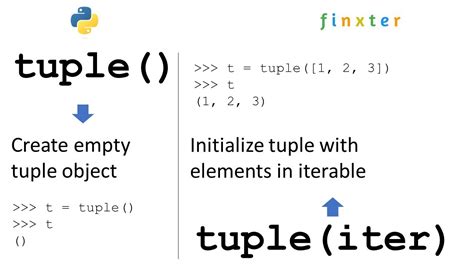
Table of Contents
How to Initialize a Tuple in Python: A Comprehensive Guide
Tuples, an integral part of Python's data structures, are ordered, immutable sequences of items. Understanding how to initialize them effectively is crucial for any Python programmer, regardless of experience level. This comprehensive guide will explore various methods for tuple initialization, offering practical examples and explaining the nuances of each approach. We'll delve into basic initialization, handling different data types, and advanced techniques for creating complex tuples.
Understanding Tuple Immutability
Before diving into initialization, it's vital to grasp the core characteristic of tuples: immutability. Once a tuple is created, its elements cannot be changed, added, or removed. This immutability offers several advantages:
- Data Integrity: Ensures that data within the tuple remains consistent throughout the program's execution, preventing accidental modification.
- Thread Safety: Makes tuples suitable for use in multi-threaded environments, as multiple threads can access a tuple concurrently without risking data corruption.
- Optimization: Python can optimize the memory management of tuples, leading to potential performance gains.
This immutability, however, means you need to create a new tuple if you need to alter its contents.
Basic Tuple Initialization: The Simplest Approach
The most straightforward way to initialize a tuple is by enclosing a sequence of items within parentheses ()
, separating them with commas ,
.
# Initializing an empty tuple
empty_tuple = ()
# Initializing a tuple with integers
integer_tuple = (1, 2, 3, 4, 5)
# Initializing a tuple with strings
string_tuple = ("apple", "banana", "cherry")
# Initializing a tuple with mixed data types
mixed_tuple = (1, "hello", 3.14, True)
# A single-element tuple requires a trailing comma
single_element_tuple = (1,) # Note the trailing comma
print(empty_tuple) # Output: ()
print(integer_tuple) # Output: (1, 2, 3, 4, 5)
print(string_tuple) # Output: ('apple', 'banana', 'cherry')
print(mixed_tuple) # Output: (1, 'hello', 3.14, True)
print(single_element_tuple) # Output: (1,)
The trailing comma in the single_element_tuple
is crucial. Without it, Python interprets (1)
as an integer in parentheses, not a tuple.
Using the tuple()
Constructor
Python's built-in tuple()
constructor provides a flexible alternative for creating tuples from various iterable objects like lists, strings, and ranges.
# Creating a tuple from a list
my_list = [10, 20, 30]
tuple_from_list = tuple(my_list)
print(tuple_from_list) # Output: (10, 20, 30)
# Creating a tuple from a string
my_string = "Python"
tuple_from_string = tuple(my_string)
print(tuple_from_string) # Output: ('P', 'y', 't', 'h', 'o', 'n')
# Creating a tuple from a range
tuple_from_range = tuple(range(5))
print(tuple_from_range) # Output: (0, 1, 2, 3, 4)
The tuple()
constructor efficiently converts iterables into tuples. This approach is especially useful when you're working with data from other sources or need to dynamically create tuples based on existing iterables.
Tuple Packing and Unpacking
Tuple packing and unpacking are elegant features that streamline tuple creation and data manipulation.
Packing: Automatically creates a tuple from a sequence of values.
packed_tuple = 1, 2, "three", 4.0
print(packed_tuple) # Output: (1, 2, 'three', 4.0)
Notice the absence of parentheses; the comma-separated values automatically become a tuple.
Unpacking: Assigns the elements of a tuple to individual variables.
a, b, c, d = packed_tuple
print(a) # Output: 1
print(b) # Output: 2
print(c) # Output: three
print(d) # Output: 4.0
This is incredibly useful for assigning multiple return values from a function to distinct variables.
Nested Tuples: Creating Complex Structures
Tuples can contain other tuples, creating nested structures for representing hierarchical data.
nested_tuple = (1, 2, (3, 4, (5, 6)), 7)
print(nested_tuple) # Output: (1, 2, (3, 4, (5, 6)), 7)
# Accessing nested elements
print(nested_tuple[2][1]) # Output: 4
print(nested_tuple[2][2][1]) # Output: 6
Nested tuples provide a powerful way to organize complex data, mimicking structures like trees or graphs. However, navigating nested tuples requires careful indexing.
Tuple Comprehension: A Concise Approach (for derived tuples)
While tuples themselves are immutable, you can create new tuples based on existing iterables using tuple comprehension – a concise way to create tuples by applying an expression to each item in an iterable. Remember, this creates a new tuple; it doesn't modify the original.
numbers = [1, 2, 3, 4, 5]
squared_numbers = tuple(x**2 for x in numbers)
print(squared_numbers) # Output: (1, 4, 9, 16, 25)
#More complex example: filtering and transforming
even_squares = tuple(x**2 for x in numbers if x % 2 == 0)
print(even_squares) #Output: (4, 16)
Tuple comprehension offers a compact and readable approach for generating tuples from existing iterables, particularly when combined with conditional logic.
Handling Errors During Tuple Initialization
While generally straightforward, errors can occur during tuple initialization. The most common include:
- SyntaxError: Forgetting the comma in a single-element tuple or incorrect parenthesis usage.
- TypeError: Attempting to modify a tuple after its creation (remember, tuples are immutable!).
- IndexError: Accessing an element beyond the tuple's bounds.
Best Practices for Tuple Initialization
- Clarity: Choose the initialization method that best reflects the data and enhances readability.
- Efficiency: For large datasets or frequent tuple creation, consider using the
tuple()
constructor for optimization. - Immutability awareness: Remember that tuples are immutable. Any operation that appears to modify a tuple actually creates a new one.
- Error handling: Implement appropriate error handling to gracefully manage potential issues during tuple creation and access.
Advanced Scenarios and Use Cases
Tuples find extensive application across various Python programming domains. Here are a few advanced use cases:
-
Representing Records: Tuples are ideal for representing records or structured data where the order of elements is significant. For example, a student record could be represented as a tuple:
student = ("John Doe", 20, "Computer Science")
. -
Function Arguments: Tuples can be used to pass multiple arguments to a function, especially when the number of arguments is not fixed.
-
Returning Multiple Values: Functions can return multiple values as a single tuple, facilitating the assignment of individual return values to different variables through unpacking.
-
Data Integrity in Concurrent Programming: As mentioned earlier, the immutability of tuples makes them safe for use in concurrent programming, minimizing the risk of data races or corruption.
-
Key-Value Pairs (with caution): While dictionaries are generally preferred for key-value pairs, tuples can be used in specific situations (e.g., when keys are immutable and order matters) but be mindful of the performance trade-offs compared to dictionaries.
Conclusion
Mastering tuple initialization is a cornerstone of proficient Python programming. This guide has provided a comprehensive overview of various initialization techniques, from basic methods to advanced scenarios. By understanding the nuances of tuple immutability and utilizing the appropriate initialization methods for your specific needs, you can leverage the power and efficiency of tuples to write cleaner, more robust, and efficient Python code. Remember to choose the method that best suits your needs, prioritizing readability and efficient code execution. The examples and explanations provided here serve as a solid foundation for building your Python skills and working effectively with tuples.
Latest Posts
Latest Posts
-
What Is The Molar Mass Of Phosphoric Acid
Apr 03, 2025
-
What Type Of Joint Is In The Skull
Apr 03, 2025
-
Which Of The Following Is An Implicit Cost Of Production
Apr 03, 2025
-
Select The Sentence That Is Punctuated Correctly
Apr 03, 2025
-
Which Of The Following Is The Correct Accounting Equation
Apr 03, 2025
Related Post
Thank you for visiting our website which covers about How To Initialize A Tuple In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.