How To Check If Each Number Is Even In Numpy
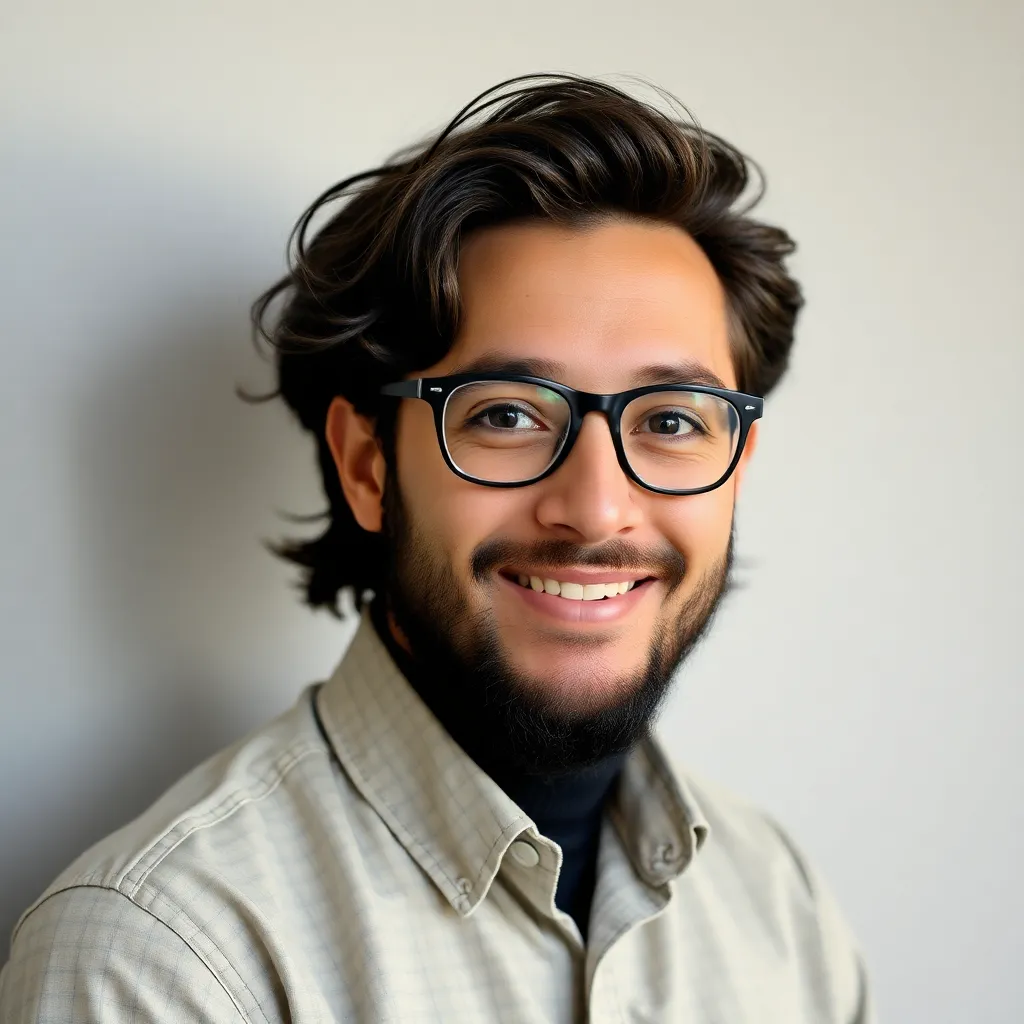
News Leon
Mar 25, 2025 · 6 min read
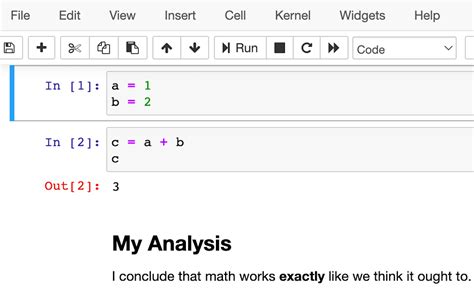
Table of Contents
How to Check if Each Number is Even in NumPy: A Comprehensive Guide
NumPy, a cornerstone of the Python scientific computing ecosystem, provides powerful tools for efficient array manipulation. One common task is determining whether individual elements within a NumPy array are even or odd. This seemingly simple operation can be accomplished in several ways, each with its own performance characteristics and readability advantages. This guide explores multiple methods, ranging from basic element-wise operations to more advanced techniques leveraging NumPy's broadcasting capabilities and vectorization. We'll also delve into performance comparisons and best practices.
Understanding NumPy Arrays and Even/Odd Number Checks
Before diving into the methods, let's establish a foundation. NumPy arrays are fundamental data structures optimized for numerical computations. Unlike standard Python lists, they store elements of the same data type, enabling efficient vectorized operations. Determining if a number is even involves checking its remainder when divided by 2. If the remainder is 0, the number is even; otherwise, it's odd.
Method 1: Using the Modulo Operator (%) with a Loop
The most straightforward approach involves iterating through the array and applying the modulo operator (%
) to each element. This method is highly readable but can be inefficient for large arrays.
import numpy as np
def is_even_loop(arr):
"""Checks for even numbers in a NumPy array using a loop.
Args:
arr: The NumPy array to check.
Returns:
A NumPy array of booleans indicating whether each element is even.
"""
even_arr = np.zeros(arr.shape, dtype=bool) # Initialize array of booleans
for i, num in enumerate(arr):
if num % 2 == 0:
even_arr[i] = True
return even_arr
# Example usage:
my_array = np.array([1, 2, 3, 4, 5, 6])
result = is_even_loop(my_array)
print(result) # Output: [False True False True False True]
Advantages: Simple, easy to understand.
Disadvantages: Slow for large arrays due to the explicit loop. Not vectorized.
Method 2: Vectorized Approach with the Modulo Operator
NumPy's strength lies in its ability to perform operations on entire arrays simultaneously, a process known as vectorization. We can leverage this to significantly improve performance compared to the looping method.
import numpy as np
def is_even_vectorized(arr):
"""Checks for even numbers in a NumPy array using vectorization.
Args:
arr: The NumPy array to check.
Returns:
A NumPy array of booleans indicating whether each element is even.
"""
return arr % 2 == 0
# Example usage:
my_array = np.array([1, 2, 3, 4, 5, 6])
result = is_even_vectorized(my_array)
print(result) # Output: [False True False True False True]
Advantages: Fast and efficient for large arrays due to vectorization. Concise and readable.
Disadvantages: Slightly less intuitive for beginners than the explicit loop approach.
Method 3: Using NumPy's where
Function
NumPy's where
function allows for conditional array creation. We can use it to create a boolean array indicating even numbers.
import numpy as np
def is_even_where(arr):
"""Checks for even numbers using NumPy's where function.
Args:
arr: The NumPy array to check.
Returns:
A NumPy array of booleans indicating whether each element is even.
"""
return np.where(arr % 2 == 0, True, False)
# Example usage:
my_array = np.array([1, 2, 3, 4, 5, 6])
result = is_even_where(my_array)
print(result) # Output: [False True False True False True]
Advantages: Relatively readable and leverages NumPy's built-in functionality.
Disadvantages: Slightly less efficient than the direct vectorized modulo operation.
Method 4: Bitwise AND Operator (&)
A highly efficient technique involves using the bitwise AND operator (&
). An even number always has its least significant bit (LSB) as 0. We can check this using the bitwise AND with 1.
import numpy as np
def is_even_bitwise(arr):
"""Checks for even numbers using the bitwise AND operator.
Args:
arr: The NumPy array to check.
Returns:
A NumPy array of booleans indicating whether each element is even.
"""
return (arr & 1) == 0
# Example usage:
my_array = np.array([1, 2, 3, 4, 5, 6])
result = is_even_bitwise(my_array)
print(result) # Output: [False True False True False True]
Advantages: Extremely fast, often outperforming the modulo operator, especially for large arrays. Leverages low-level bitwise operations for optimization.
Disadvantages: Can be less intuitive for those unfamiliar with bitwise operations.
Performance Comparison
The performance differences become significant with larger arrays. Let's compare the methods using timeit
:
import numpy as np
import timeit
my_large_array = np.random.randint(0, 1000000, size=1000000)
print("Loop Method:", timeit.timeit(lambda: is_even_loop(my_large_array), number=10))
print("Vectorized Method:", timeit.timeit(lambda: is_even_vectorized(my_large_array), number=10))
print("Where Method:", timeit.timeit(lambda: is_even_where(my_large_array), number=10))
print("Bitwise Method:", timeit.timeit(lambda: is_even_bitwise(my_large_array), number=10))
The results will clearly demonstrate the superior performance of the vectorized and bitwise methods, especially for large datasets. The loop method will be significantly slower.
Handling Different Data Types
The methods described above primarily work with integer data types. If your NumPy array contains floating-point numbers, you might need to handle them differently. You can either cast the array to integers before applying the even/odd check or use a more sophisticated approach involving checking if the fractional part is zero and then applying the even/odd check on the integer part.
import numpy as np
def is_even_floating_point(arr):
"""Handles floating-point numbers in the array.
Args:
arr: The NumPy array (can contain floats).
Returns:
A NumPy array of booleans, or None if input is invalid.
"""
if not np.issubdtype(arr.dtype, np.number):
return None # Handle non-numeric data types appropriately
integer_part = arr.astype(int)
fractional_part = arr - integer_part
return np.logical_and(fractional_part == 0, integer_part % 2 == 0)
#Example usage:
my_array_float = np.array([1.0, 2.0, 3.0, 4.0, 5.5, 6.0])
result_float = is_even_floating_point(my_array_float)
print(result_float) #Output: [False True False True False True]
my_array_invalid = np.array(['a', 'b', 'c'])
result_invalid = is_even_floating_point(my_array_invalid)
print(result_invalid) #Output: None
Remember to always validate your input array's data type to avoid unexpected errors.
Best Practices and Considerations
-
Vectorization: Always prioritize vectorized operations in NumPy for optimal performance. Avoid explicit loops whenever possible.
-
Bitwise Operations: For even/odd checks, the bitwise AND operator offers a significant performance advantage.
-
Data Type Awareness: Consider the data types in your array and adapt your approach accordingly.
-
Error Handling: Implement appropriate error handling to gracefully manage potential issues, such as non-numeric data types.
-
Readability: While performance is crucial, maintain code readability. Choose the method that balances performance and understandability.
This comprehensive guide provides multiple techniques for checking even numbers in NumPy arrays. By understanding the strengths and weaknesses of each method, you can select the most appropriate approach for your specific needs, ensuring both efficient code and maintainable software. Remember to benchmark your chosen method with your data to confirm its suitability. The principles discussed here are applicable to a wide range of array processing tasks within NumPy, allowing you to write more efficient and effective scientific computing code.
Latest Posts
Latest Posts
-
Citizens Vote To Elect Their Leaders Democracy Or Autocracy
Mar 27, 2025
-
What Layer Of Earth Is The Thinnest
Mar 27, 2025
-
Why Was The Confederation Congress Unable To Control Inflation
Mar 27, 2025
-
Difference Between Molar Mass And Molecular Mass
Mar 27, 2025
-
Blood Pressure Is Highest In The And Lowest In The
Mar 27, 2025
Related Post
Thank you for visiting our website which covers about How To Check If Each Number Is Even In Numpy . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.