How To Add Numbers In Python
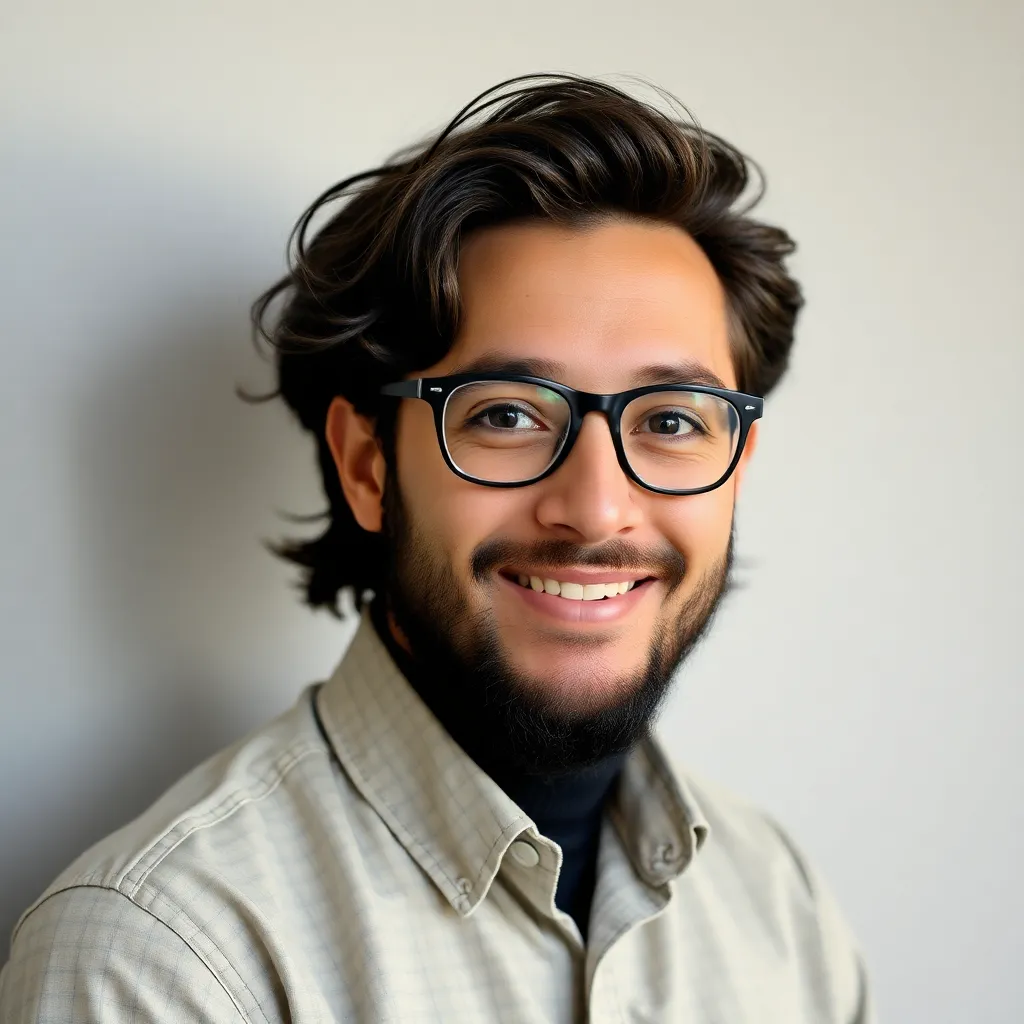
News Leon
Mar 19, 2025 · 6 min read
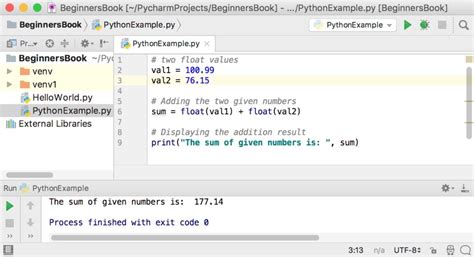
Table of Contents
How to Add Numbers in Python: A Comprehensive Guide
Python, renowned for its readability and versatility, offers several elegant ways to add numbers. Whether you're dealing with single digits, large lists, or complex data structures, Python provides the tools to perform addition efficiently and effectively. This comprehensive guide explores various methods, catering to different scenarios and skill levels. We'll cover basic arithmetic, working with lists and arrays, utilizing NumPy for enhanced performance, and handling potential errors.
1. Basic Arithmetic: The +
Operator
The simplest way to add numbers in Python is by using the +
operator. This works perfectly for adding two or more individual numbers directly.
# Adding two integers
result = 10 + 5
print(f"The sum is: {result}") # Output: The sum is: 15
# Adding floating-point numbers
result = 3.14 + 2.71
print(f"The sum is: {result}") # Output: The sum is: 5.85
# Adding integers and floats
result = 10 + 3.14
print(f"The sum is: {result}") # Output: The sum is: 13.14
This method is intuitive and straightforward, making it ideal for basic arithmetic operations.
2. Adding Numbers in Lists and Tuples
When dealing with collections of numbers, such as lists or tuples, you can't directly use the +
operator to add all elements. Instead, you'll need to use loops or built-in functions like sum()
.
2.1 Using Loops:
Loops provide a clear and easily understandable approach for iterating through a list and accumulating the sum.
numbers = [1, 2, 3, 4, 5]
total = 0
for number in numbers:
total += number
print(f"The sum of the list is: {total}") # Output: The sum of the list is: 15
# Handling empty lists to avoid errors:
empty_list = []
total = 0
if empty_list: #Check if list is not empty
for number in empty_list:
total += number
else:
print("The list is empty. Cannot calculate the sum.")
numbers_tuple = (10, 20, 30)
total = 0
for number in numbers_tuple:
total += number
print(f"The sum of the tuple is: {total}") # Output: The sum of the tuple is: 60
This approach is highly flexible, allowing for additional logic within the loop if needed (e.g., conditional summing).
2.2 Using the sum()
function:
Python's built-in sum()
function offers a more concise way to add numbers in a list or tuple.
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(f"The sum of the list is: {total}") # Output: The sum of the list is: 15
numbers_tuple = (10, 20, 30)
total = sum(numbers_tuple)
print(f"The sum of the tuple is: {total}") # Output: The sum of the tuple is: 60
#Handling empty lists gracefully:
empty_list = []
total = sum(empty_list, 0) #The second argument provides a default value if the list is empty.
print(f"The sum of the empty list is: {total}") #Output: The sum of the empty list is: 0
The sum()
function is generally preferred for its efficiency and readability, especially when dealing with large lists. The optional second argument provides a default starting value for the sum, crucial for handling empty lists.
3. Adding Numbers in Multi-Dimensional Lists (Matrices)
For multi-dimensional lists (essentially matrices), nested loops are required to traverse and sum all the elements.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = 0
for row in matrix:
for element in row:
total += element
print(f"The sum of all elements in the matrix is: {total}") # Output: 45
This approach iterates through each row and then each element within each row, accumulating the sum. While functional, this can become less readable and more prone to errors with higher dimensions.
4. Leveraging NumPy for Efficient Array Operations
NumPy, a powerful library for numerical computation in Python, significantly enhances the efficiency of array operations, particularly for large datasets. NumPy's sum()
function is highly optimized.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
total = np.sum(array)
print(f"The sum of the array is: {total}") # Output: 15
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
total = np.sum(matrix)
print(f"The sum of the matrix is: {total}") # Output: 45
# Summing along specific axes:
row_sums = np.sum(matrix, axis=0) #Sum across rows (columns)
col_sums = np.sum(matrix, axis=1) #Sum across columns (rows)
print(f"Row sums: {row_sums}") #Output: Row sums: [12 15 18]
print(f"Column sums: {col_sums}") #Output: Column sums: [ 6 15 24]
NumPy's vectorized operations drastically improve performance, making it the preferred choice for numerical computations involving large arrays and matrices. The ability to specify the axis
parameter for summation along rows or columns adds substantial flexibility.
5. Handling Potential Errors: Exception Handling
Robust code anticipates and handles potential errors. For example, attempting to sum elements in a list containing non-numeric values will raise a TypeError
. Exception handling gracefully manages such situations.
mixed_list = [1, 2, 'a', 4, 5]
try:
total = sum(mixed_list)
print(f"The sum is: {total}")
except TypeError:
print("Error: List contains non-numeric values.")
#Alternative approach using a list comprehension for filtering:
numeric_list = [x for x in mixed_list if isinstance(x, (int, float))]
total = sum(numeric_list)
print(f"The sum of numeric values is: {total}")
The try-except
block prevents the program from crashing due to unexpected input. A more proactive approach involves pre-processing the list to filter out non-numeric elements.
6. Adding Numbers from User Input
Frequently, you'll need to add numbers provided by the user. This involves input validation to ensure the user enters valid numerical data.
num1 = input("Enter the first number: ")
num2 = input("Enter the second number: ")
try:
num1 = float(num1)
num2 = float(num2)
total = num1 + num2
print(f"The sum is: {total}")
except ValueError:
print("Error: Invalid input. Please enter numbers only.")
This example demonstrates input validation to catch potential ValueError
exceptions if the user enters non-numeric characters.
7. Advanced Techniques: Recursive Summation
While less commonly used for simple addition, recursion offers an alternative approach, particularly useful for summing elements in recursively defined data structures.
def recursive_sum(numbers):
if not numbers:
return 0
else:
return numbers[0] + recursive_sum(numbers[1:])
numbers = [1, 2, 3, 4, 5]
total = recursive_sum(numbers)
print(f"The recursive sum is: {total}") # Output: 15
This recursive function adds the first element to the sum of the remaining elements until the base case (empty list) is reached. Recursion can be elegant for specific scenarios but often sacrifices efficiency for readability in simple addition tasks.
Conclusion: Choosing the Right Method
The optimal method for adding numbers in Python depends on the context. For simple additions of individual numbers, the +
operator is sufficient. Lists and tuples are efficiently handled with the built-in sum()
function. For large arrays and matrices, NumPy offers significant performance benefits. Always incorporate error handling to create robust and reliable code. Finally, while recursion provides an alternative approach, it's generally less efficient than iterative methods for straightforward summation tasks. By mastering these techniques, you'll be well-equipped to handle a wide range of numerical addition problems in Python with confidence and efficiency.
Latest Posts
Latest Posts
-
Which Of The Following Are Homogeneous Mixtures
Mar 19, 2025
-
A Parallel Plate Capacitor Has Circular Plates
Mar 19, 2025
-
Distance From Earth To Moon In Meters Scientific Notation
Mar 19, 2025
-
What Is A 1 1 Ratio
Mar 19, 2025
-
5 Paragraph Essay Benefits Of Exercise
Mar 19, 2025
Related Post
Thank you for visiting our website which covers about How To Add Numbers In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.