Count Vowels In A String Python
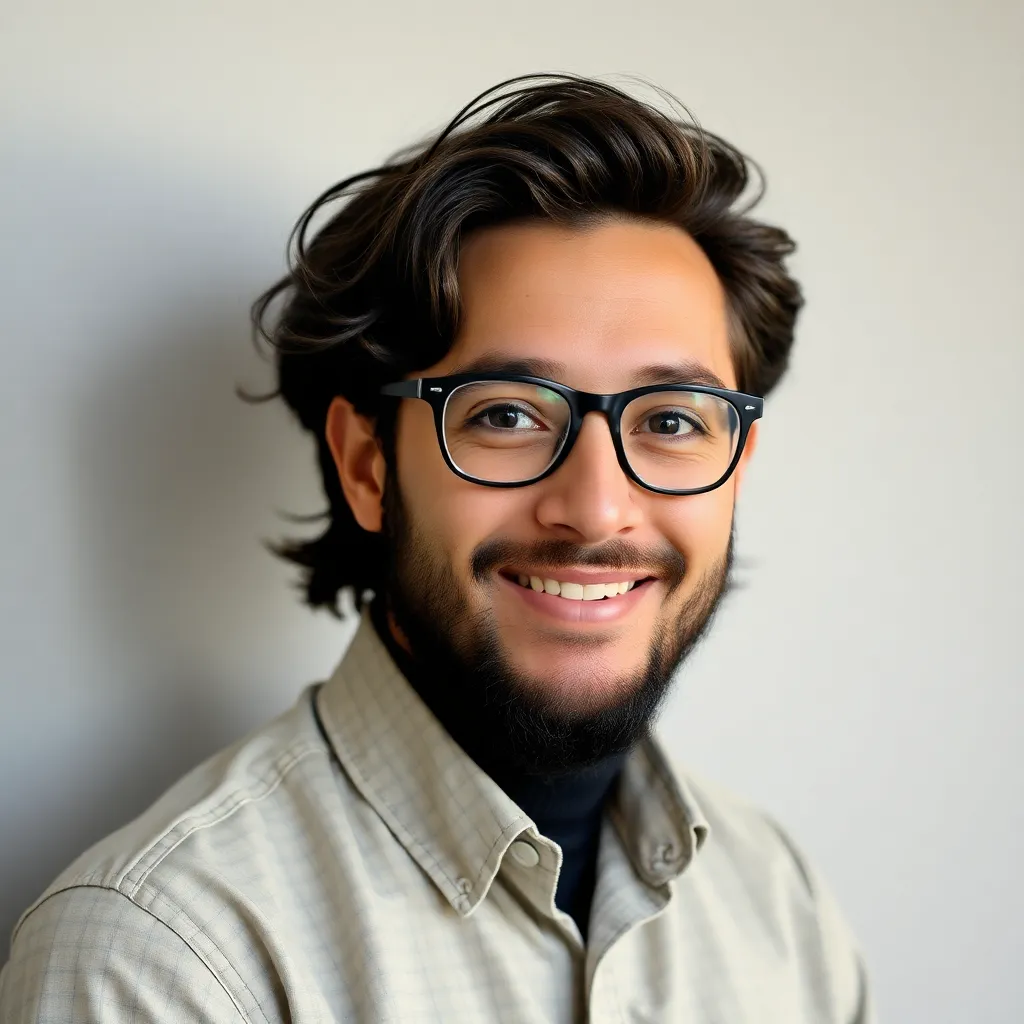
News Leon
Apr 01, 2025 · 6 min read
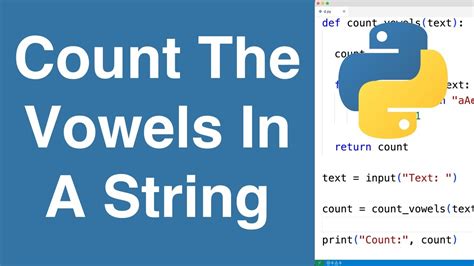
Table of Contents
Counting Vowels in a String: A Comprehensive Guide to Python Techniques
Counting vowels in a string is a fundamental task in programming, frequently encountered in various applications like text processing, data analysis, and linguistic studies. Python, with its versatility and rich libraries, offers multiple approaches to efficiently solve this problem. This comprehensive guide delves into different methods, exploring their complexities and advantages, enabling you to choose the most suitable technique for your specific needs. We'll move from the most basic approaches to more advanced and optimized solutions.
Method 1: The Manual Approach – Looping and Conditional Statements
This is the most straightforward method, perfect for beginners to grasp the core logic. We iterate through the string and check each character against a set of vowels.
def count_vowels_manual(input_string):
"""Counts vowels (a, e, i, o, u) in a string using a loop.
Args:
input_string: The string to process.
Returns:
The number of vowels in the string.
"""
vowels = "aeiouAEIOU"
vowel_count = 0
for char in input_string:
if char in vowels:
vowel_count += 1
return vowel_count
#Example usage
string = "Hello, World!"
vowel_count = count_vowels_manual(string)
print(f"The number of vowels in '{string}' is: {vowel_count}")
This method is highly readable and easily understandable. However, for very large strings, its performance might become less efficient compared to other techniques. The time complexity is O(n), where n is the length of the string.
Advantages:
- Simplicity: Easy to understand and implement, ideal for educational purposes.
- Readability: Code is clear and concise.
Disadvantages:
- Performance: Can be slower for large strings.
- Case Sensitivity: Requires handling uppercase and lowercase vowels separately unless you convert the entire string to lowercase beforehand.
Method 2: Leveraging count()
method for each vowel
Python's built-in count()
method offers a slightly more concise way to count individual vowels. We can call this method for each vowel and sum the results.
def count_vowels_count_method(input_string):
"""Counts vowels using the string's count() method.
Args:
input_string: The string to process.
Returns:
The number of vowels in the string.
"""
input_string = input_string.lower() #handle case sensitivity
return sum(input_string.count(vowel) for vowel in "aeiou")
#Example usage
string = "Hello, World!"
vowel_count = count_vowels_count_method(string)
print(f"The number of vowels in '{string}' is: {vowel_count}")
This approach reduces the explicit looping, making the code more compact. It is also relatively efficient for smaller strings. However, it still iterates through the string multiple times (once for each vowel).
Advantages:
- Conciseness: More compact than the manual loop approach.
- Readability: Still relatively easy to understand.
Disadvantages:
- Multiple Iterations: Iterates through the string multiple times, impacting performance for larger strings.
- Case sensitivity (handled): Requires converting string to lower case to handle both upper and lower case vowels.
Method 3: Using Regular Expressions – The Power of re.findall()
Regular expressions provide a powerful and flexible way to search for patterns within strings. The re.findall()
function is particularly useful for this task.
import re
def count_vowels_regex(input_string):
"""Counts vowels using regular expressions.
Args:
input_string: The string to process.
Returns:
The number of vowels in the string.
"""
vowels = re.findall(r'[aeiouAEIOU]', input_string) #Find all vowels (case-insensitive could be used here)
return len(vowels)
#Example usage
string = "Hello, World!"
vowel_count = count_vowels_regex(string)
print(f"The number of vowels in '{string}' is: {vowel_count}")
Regular expressions offer a concise solution. The re.findall()
method efficiently finds all occurrences of the vowel pattern, and len()
directly provides the count. This method is generally efficient even for larger strings.
Advantages:
- Efficiency: Generally efficient, especially for large strings.
- Flexibility: Easily adaptable for more complex vowel counting scenarios (e.g., accented vowels).
- Conciseness: Compact and expressive code.
Disadvantages:
- Learning Curve: Requires familiarity with regular expressions.
- Potential Overhead: Regular expression matching might have some slight overhead compared to simpler methods for very small strings.
Method 4: List Comprehension – A Pythonic Approach
List comprehension offers a Pythonic and efficient way to achieve the same result in a single line of code.
def count_vowels_comprehension(input_string):
"""Counts vowels using list comprehension.
Args:
input_string: The string to process.
Returns:
The number of vowels in the string.
"""
return len([char for char in input_string.lower() if char in 'aeiou'])
# Example usage
string = "Hello, World!"
vowel_count = count_vowels_comprehension(string)
print(f"The number of vowels in '{string}' is: {vowel_count}")
This approach combines the iteration and conditional check into a single, elegant expression. It is generally as efficient as the manual loop approach, but with more compact code.
Advantages:
- Efficiency: Comparable to the manual loop for large strings; very efficient for medium-sized strings.
- Readability (debatable): Can be more readable than the manual loop to experienced Python programmers.
- Conciseness: Extremely compact code.
Disadvantages:
- Readability (debatable): Might be less readable for beginners.
- Slightly less flexible than regex: For complex vowel counting scenarios, regex is more adaptable.
Method 5: Using filter
and sum
– Functional Programming Style
Python's functional programming capabilities can also be used to elegantly count vowels. filter
selects the vowels, and sum
counts them.
def count_vowels_functional(input_string):
"""Counts vowels using filter and sum.
Args:
input_string: The string to process.
Returns:
The number of vowels in the string.
"""
return sum(1 for char in input_string.lower() if char in 'aeiou')
# Example usage
string = "Hello, World!"
vowel_count = count_vowels_functional(string)
print(f"The number of vowels in '{string}' is: {vowel_count}")
This approach is concise and leverages Python's functional features. It’s efficient, particularly for larger strings. The use of a generator expression ((1 for ... )
) avoids creating an intermediate list, enhancing performance.
Advantages:
- Efficiency: Efficient for larger strings due to the generator expression.
- Elegance: Demonstrates Python's functional programming capabilities.
- Readability (debatable): Can be very readable to programmers familiar with functional programming.
Disadvantages:
- Readability (debatable): Might be less immediately understandable to beginners.
- Less Flexible than regex: Limited adaptability for complex scenarios.
Choosing the Right Method
The optimal method depends on several factors:
- String size: For very small strings, the differences in performance are negligible. For larger strings, regular expressions or list comprehensions generally offer better performance.
- Code readability: The manual loop is easiest to understand, while list comprehensions and functional approaches are more concise but might require more experience to grasp immediately.
- Complexity of the task: For simple vowel counting, any method will suffice. If you need to handle accented vowels or other complex patterns, regular expressions are the most flexible.
- Programming style preference: Choose the method that best aligns with your preferred coding style (imperative, functional, etc.).
This detailed comparison helps you select the most efficient and readable approach for your specific vowel counting task in Python. Remember to consider the trade-offs between performance, readability, and the complexity of your needs. For most common scenarios, the regular expression or list comprehension methods provide an excellent balance of efficiency and readability.
Latest Posts
Latest Posts
-
Why Are Fleas Hard To Squish
Apr 03, 2025
-
How Many Chromosomes In A Daughter Cell
Apr 03, 2025
-
Why Do Bones Heal Quicker Than Cartilage
Apr 03, 2025
-
Can Magnitude Be Negative In Physics
Apr 03, 2025
-
What Is The Area Of Triangle Rst
Apr 03, 2025
Related Post
Thank you for visiting our website which covers about Count Vowels In A String Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.