Convert Timestamp To Date Time Python
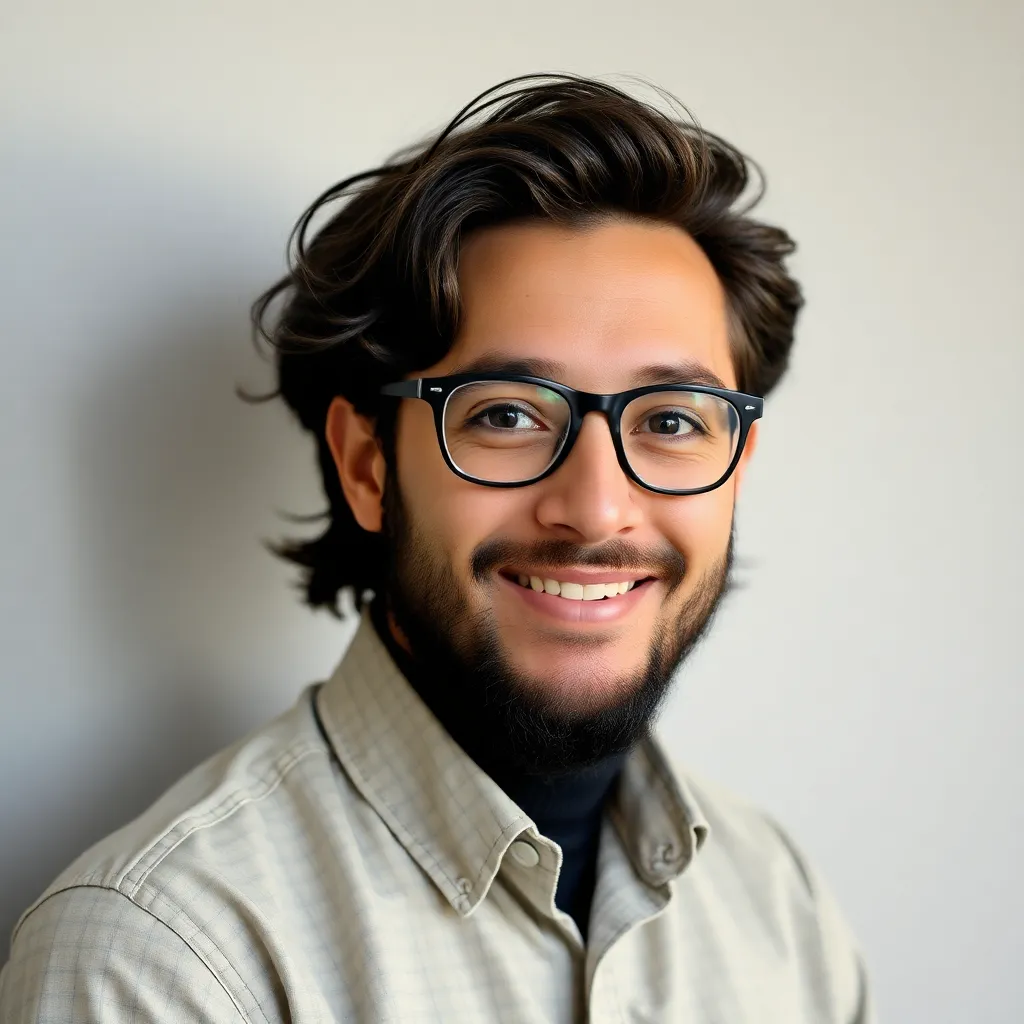
News Leon
Mar 14, 2025 · 4 min read
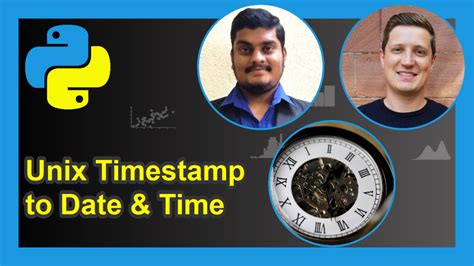
Table of Contents
Convert Timestamp to Datetime in Python: A Comprehensive Guide
Converting timestamps to human-readable datetime objects is a fundamental task in many Python programming projects, especially when dealing with data from databases, APIs, or log files. Timestamps, often represented as Unix timestamps (seconds since the epoch), lack the readability crucial for presenting information to users or performing date-based analysis. This comprehensive guide will explore various methods and techniques for efficiently and accurately converting timestamps to datetime objects in Python, covering different timestamp formats and potential challenges.
Understanding Timestamps and Datetime Objects
Before diving into the conversion process, it's crucial to understand the key differences between timestamps and datetime objects:
-
Timestamps: These are numerical representations of a point in time, typically the number of seconds (or milliseconds) since the Unix epoch (January 1, 1970, at 00:00:00 Coordinated Universal Time (UTC)). They are compact and efficient for storage but lack intuitive readability.
-
Datetime Objects: These are Python objects representing a specific date and time, offering methods for extracting individual components (year, month, day, hour, minute, second), performing date arithmetic, and formatting for display. They are human-friendly and support various operations crucial for data manipulation and analysis.
Python Libraries for Timestamp Conversion
Python offers several powerful libraries for handling timestamps and datetime objects. The most commonly used are:
-
datetime
: This built-in module provides classes for working with dates and times. It's the foundation for many timestamp conversion operations. -
time
: Another built-in module that provides time-related functions, including converting timestamps to other time representations. -
calendar
: Useful for calendar-related operations, which can be helpful in context of datetime manipulation. -
dateutil
(external): A powerful third-party library, often installed viapip install python-dateutil
, extending the capabilities of thedatetime
module with more advanced parsing and handling of various date and time formats.
Common Timestamp Formats and Conversion Techniques
Timestamps can appear in various formats. The most common are:
1. Unix Timestamp (Seconds since Epoch)
This is arguably the most prevalent timestamp format. Converting it to a datetime object is straightforward using the datetime.datetime.fromtimestamp()
method:
import datetime
timestamp = 1678886400 # Example Unix timestamp (seconds)
datetime_object = datetime.datetime.fromtimestamp(timestamp)
print(datetime_object) # Output: 2023-03-15 00:00:00
Handling Timezones: The fromtimestamp()
method defaults to your system's local timezone. For UTC, use datetime.datetime.utcfromtimestamp()
:
import datetime
utc_datetime_object = datetime.datetime.utcfromtimestamp(timestamp)
print(utc_datetime_object) # Output will be in UTC
2. Millisecond Timestamps
Some APIs or databases use milliseconds since the epoch. Adjust accordingly:
import datetime
millisecond_timestamp = 1678886400000 # Example millisecond timestamp
datetime_object = datetime.datetime.fromtimestamp(millisecond_timestamp / 1000)
print(datetime_object)
3. String Timestamps
Timestamps might be stored as strings. The dateutil
library is highly beneficial here:
from dateutil import parser
string_timestamp = "2023-03-15 00:00:00"
datetime_object = parser.parse(string_timestamp)
print(datetime_object)
The dateutil.parser.parse()
function is remarkably robust, handling a wide array of date and time string formats.
4. Handling Different Timezones
Accurately handling timezones is crucial for data integrity. The pytz
library (pip install pytz
) is invaluable:
import datetime
import pytz
timestamp = 1678886400
tz = pytz.timezone('America/New_York')
datetime_object = datetime.datetime.fromtimestamp(timestamp, tz)
print(datetime_object) # Output will reflect America/New_York timezone
Remember to replace 'America/New_York'
with the appropriate timezone string.
Formatting Datetime Objects for Output
Once you have a datetime object, formatting it for display is simple using the strftime()
method:
import datetime
datetime_object = datetime.datetime(2023, 3, 15, 10, 30, 0)
formatted_date = datetime_object.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_date) # Output: 2023-03-15 10:30:00
#Different Format Examples
formatted_date_2 = datetime_object.strftime("%B %d, %Y") #Output: March 15, 2023
formatted_date_3 = datetime_object.strftime("%I:%M %p") #Output: 10:30 AM
Consult the Python strftime()
documentation for the full range of formatting directives.
Advanced Techniques and Error Handling
1. Dealing with Invalid Timestamps
It's essential to implement error handling to gracefully manage situations where a timestamp is invalid or cannot be parsed:
import datetime
try:
timestamp = "invalid timestamp"
datetime_object = datetime.datetime.fromtimestamp(int(timestamp))
except (ValueError, TypeError) as e:
print(f"Error converting timestamp: {e}")
2. Batch Timestamp Conversion
For large datasets, optimize conversion using techniques like list comprehensions:
import datetime
timestamps = [1678886400, 1678890000, 1678893600]
datetime_objects = [datetime.datetime.fromtimestamp(ts) for ts in timestamps]
print(datetime_objects)
3. Using Pandas for Efficient DataFrame Manipulation
If working with large datasets in a tabular format, Pandas excels at efficient timestamp conversion:
import pandas as pd
data = {'timestamp': [1678886400, 1678890000, 1678893600]}
df = pd.DataFrame(data)
df['datetime'] = pd.to_datetime(df['timestamp'], unit='s')
print(df)
Pandas to_datetime()
handles various timestamp formats and provides robust error handling.
Conclusion
Converting timestamps to datetime objects in Python is a fundamental skill for any programmer working with time-series data. This guide has explored various methods, libraries, and best practices to efficiently and accurately handle this conversion, including robust error handling and advanced techniques for optimizing performance with large datasets. Remember to choose the appropriate library and method based on your specific timestamp format and requirements. Always consider timezone handling for accurate and reliable results, especially when dealing with data from different geographical locations. By mastering these techniques, you'll greatly enhance your ability to manipulate and analyze time-based data in your Python projects.
Latest Posts
Latest Posts
-
People Who Buy Stock In A Company Are Known As
Mar 15, 2025
-
The Cell Walls Of Fungi Are Made Up Of Cellulose
Mar 15, 2025
-
What Is The Basic Unit Of Heredity
Mar 15, 2025
-
9 Is What Percent Of 72
Mar 15, 2025
-
How Many Valence Electrons Are In H
Mar 15, 2025
Related Post
Thank you for visiting our website which covers about Convert Timestamp To Date Time Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.