Check If A Letter Is Present In A String Python
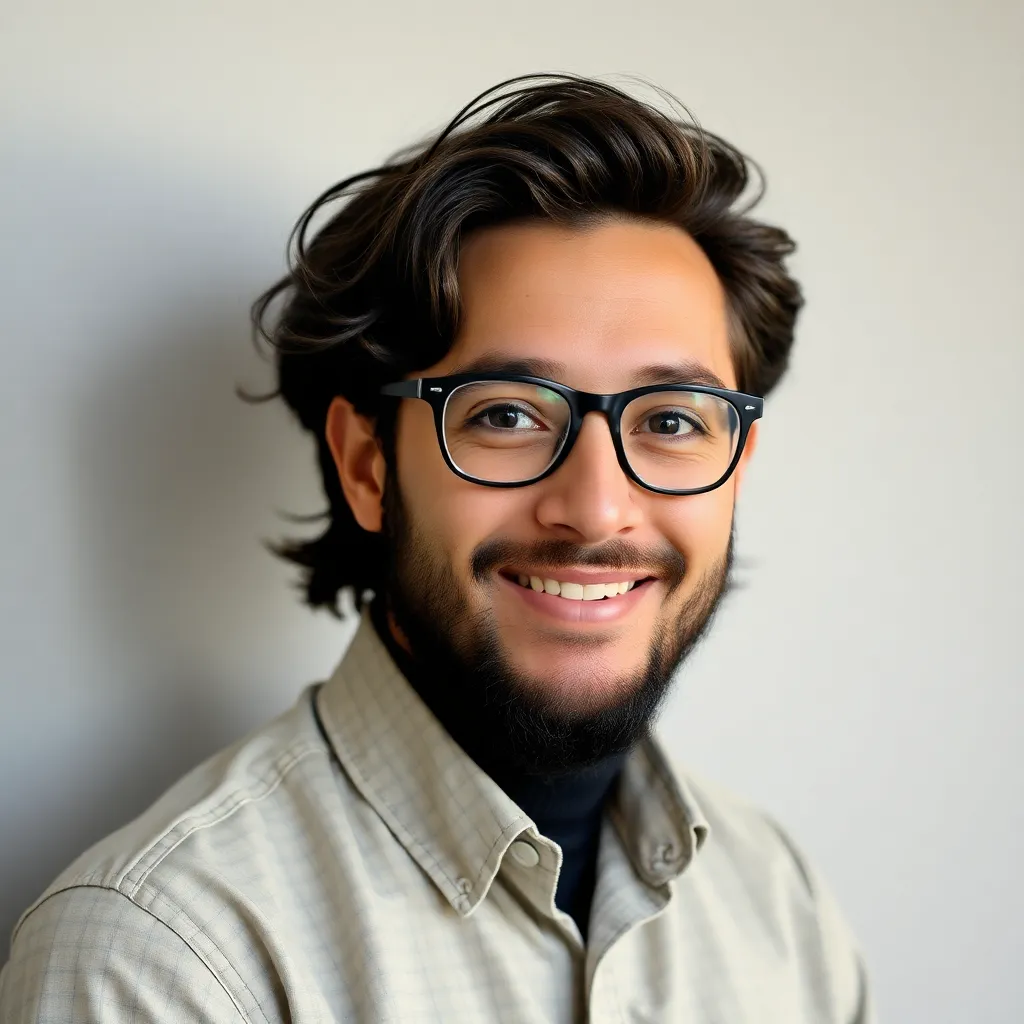
News Leon
Mar 25, 2025 · 5 min read
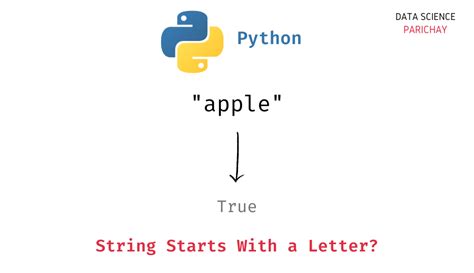
Table of Contents
Checking if a Letter is Present in a String: A Comprehensive Guide to Python Techniques
Determining whether a specific letter exists within a string is a fundamental task in many programming applications. Python, with its rich set of string manipulation functionalities, offers several elegant and efficient ways to accomplish this. This comprehensive guide will explore various techniques, ranging from simple built-in methods to more advanced approaches, focusing on efficiency and readability. We'll delve into the nuances of each method, highlighting their strengths and weaknesses, and providing practical examples to solidify your understanding.
The Power of in
Operator: The Simplest Approach
The most straightforward method to check for the presence of a letter (or any substring) within a Python string is using the in
operator. This operator returns True
if the substring is found, and False
otherwise. Its simplicity and readability make it the preferred choice for most common scenarios.
string = "Hello, World!"
letter = "o"
if letter in string:
print(f"The letter '{letter}' is present in the string.")
else:
print(f"The letter '{letter}' is not present in the string.")
This concise code snippet directly checks if 'o'
exists in "Hello, World!"
. The output clearly indicates the presence or absence of the letter. The in
operator is case-sensitive; searching for "O" would yield a different result.
Advantages:
- Simplicity: Extremely easy to understand and implement.
- Readability: The code is highly readable, enhancing maintainability.
- Efficiency: For single character checks, it's highly efficient.
Disadvantages:
- Case-sensitivity: It's case-sensitive, meaning "o" and "O" are treated as different characters.
- Limited functionality: Doesn't provide additional information like the count or index of the letter.
Leveraging find()
and index()
Methods: Retrieving Positional Information
While the in
operator confirms the presence of a letter, the find()
and index()
methods offer a more granular approach by returning the index (position) of the first occurrence of the letter. find()
returns -1 if the letter isn't found, while index()
raises a ValueError
in the same scenario.
string = "Hello, World!"
letter = "o"
index = string.find(letter)
if index != -1:
print(f"The letter '{letter}' is found at index {index}.")
else:
print(f"The letter '{letter}' is not found in the string.")
try:
index = string.index(letter)
print(f"The letter '{letter}' is found at index {index} using index().")
except ValueError:
print(f"The letter '{letter}' is not found in the string using index().")
This example demonstrates both methods. find()
provides a more robust way to handle cases where the letter might not be present, avoiding exceptions. index()
is efficient when you're certain the letter exists.
Advantages:
- Positional information: Provides the index of the first occurrence.
find()
's robustness: Handles the absence of the letter gracefully without raising exceptions.
Disadvantages:
index()
's exception:index()
can raise exceptions, requiring error handling.- Only first occurrence: Both methods only return the index of the first occurrence.
Regular Expressions: A Powerful Tool for Complex Patterns
For more intricate scenarios involving multiple letters, patterns, or case-insensitive searches, Python's regular expression module (re
) offers a powerful solution. Regular expressions provide a flexible and concise way to define search patterns.
import re
string = "Hello, World!"
letter = "o"
# Case-sensitive search
match = re.search(letter, string)
if match:
print(f"The letter '{letter}' is found (case-sensitive).")
# Case-insensitive search
match = re.search(letter, string, re.IGNORECASE)
if match:
print(f"The letter '{letter}' is found (case-insensitive).")
#Finding all occurrences
matches = re.findall(letter, string, re.IGNORECASE)
if matches:
print(f"All occurrences of '{letter}' (case-insensitive): {matches}")
else:
print(f"No occurrences of '{letter}' found.")
This code demonstrates both case-sensitive and case-insensitive searches using re.search()
. re.findall()
finds all occurrences of the letter. Regular expressions are particularly useful when dealing with complex patterns beyond simple letter searches.
Advantages:
- Flexibility: Handles complex patterns and multiple letters.
- Case-insensitive search: Easily accommodates case-insensitive searches.
- Multiple occurrences:
re.findall()
finds all occurrences.
Disadvantages:
- Complexity: Can be more complex to learn and use than simpler methods.
- Performance: For simple letter checks, it can be less efficient than the
in
operator.
Counting Occurrences: Beyond Simple Presence
Sometimes, you need to know not just if a letter is present, but how many times it appears. Python offers several ways to achieve this efficiently.
string = "Hello, World!"
letter = "o"
count = string.count(letter)
print(f"The letter '{letter}' appears {count} times.")
#Case-insensitive count using a loop and lower()
count = 0
for char in string.lower():
if char == letter.lower():
count += 1
print(f"The letter '{letter}' appears {count} times (case-insensitive).")
#Case-insensitive count using sum and a generator expression. More concise and potentially faster for very large strings.
count = sum(1 for char in string.lower() if char == letter.lower())
print(f"The letter '{letter}' appears {count} times (case-insensitive using sum).")
This example uses the built-in count()
method for a case-sensitive count. For a case-insensitive approach, we iterate through the lowercase version of the string.
Advantages:
- Quantifies occurrences: Provides the exact number of occurrences.
- Efficiency:
count()
is highly efficient for this specific task.
Disadvantages:
- Case-sensitivity of
count()
: Requires explicit handling for case-insensitive counting.
Optimizing for Performance: Considerations for Large Strings
For extremely large strings, the performance of different methods can vary significantly. The in
operator and count()
are generally efficient for most scenarios, but for extremely large datasets, carefully consider the algorithmic complexity. Using generator expressions or optimized loop structures can improve performance in such cases. Pre-processing the string (e.g., converting to lowercase) before searching can also be beneficial for case-insensitive searches.
Choosing the Right Method: A Practical Guide
The best method for checking the presence of a letter in a string depends on the specific requirements of your application:
- Simple presence check: Use the
in
operator. - Presence and index: Use
find()
for robustness orindex()
for speed if you're certain the letter exists. - Case-insensitive search: Use
re.search()
withre.IGNORECASE
or manual lowercase conversion. - Complex patterns: Use regular expressions.
- Counting occurrences: Use
count()
for case-sensitive counts, and a loop or generator expression for case-insensitive counts. - Extremely large strings: Optimize with generator expressions, pre-processing, and careful algorithm selection.
By understanding the strengths and limitations of each method, you can choose the most appropriate and efficient technique for your Python string manipulation tasks. Remember to prioritize readability and maintainability while striving for optimal performance. This comprehensive guide empowers you to tackle diverse letter-finding challenges effectively and elegantly within your Python programs.
Latest Posts
Latest Posts
-
Both Air And Food Travel Through The
Mar 28, 2025
-
Two Blocks Are In Contact On A Frictionless Table
Mar 28, 2025
-
A Production Possibilities Frontier Can Shift Outward If
Mar 28, 2025
-
What Is Embedded In The Phospholipid Bilayer
Mar 28, 2025
-
All Quadrilaterals Are Parallelograms True Or False
Mar 28, 2025
Related Post
Thank you for visiting our website which covers about Check If A Letter Is Present In A String Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.