Under Which Of The Following Conditions
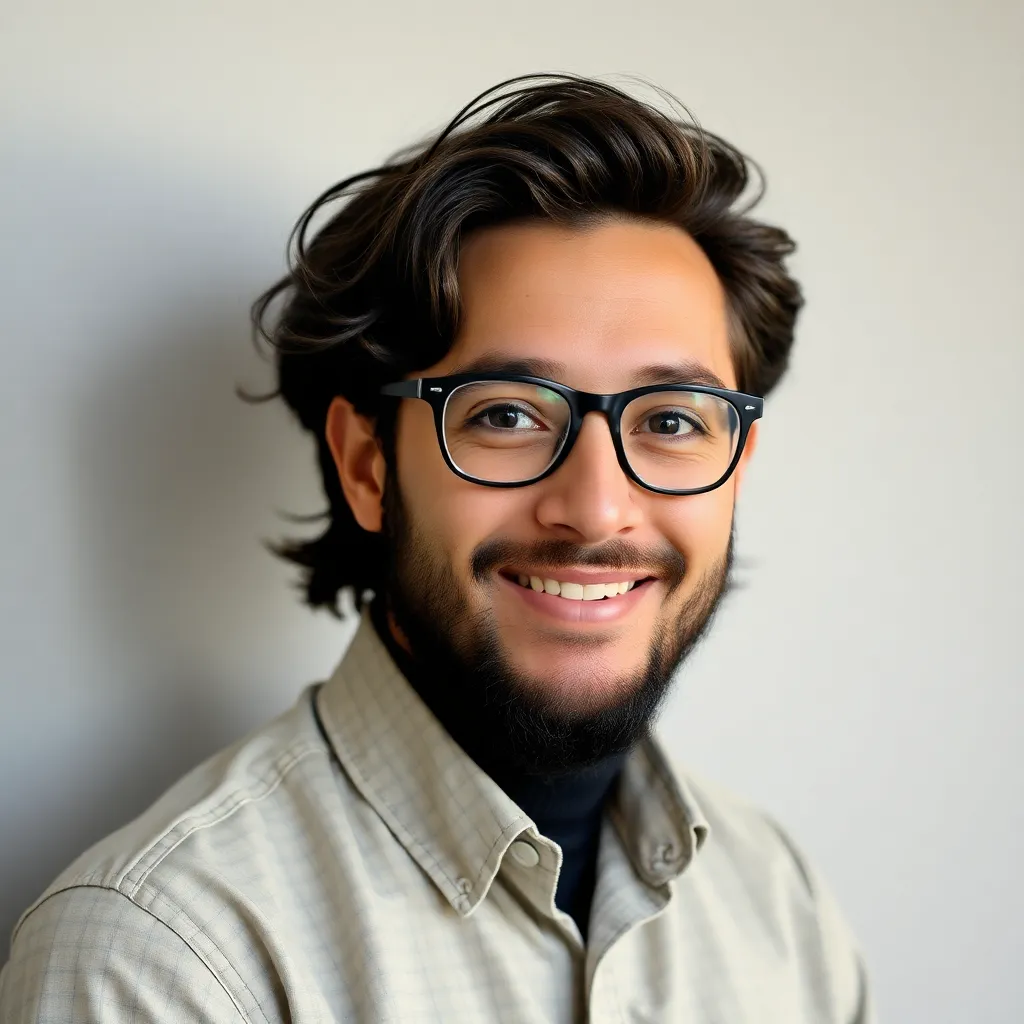
News Leon
Apr 23, 2025 · 6 min read

Table of Contents
Under Which of the Following Conditions: A Comprehensive Guide to Conditional Logic and Decision-Making
Conditional statements are the backbone of any programming language, and understanding them is crucial for building robust and efficient applications. This comprehensive guide delves into the intricacies of conditional logic, exploring various scenarios and demonstrating how to effectively utilize conditional statements to solve complex problems. We'll go beyond the basics, exploring nested conditionals, switch statements (or their equivalents), and the importance of error handling within conditional structures.
What are Conditional Statements?
Conditional statements, at their core, allow your program to make decisions based on certain conditions. They control the flow of execution, determining which blocks of code are executed and which are skipped. The most common type is the if
statement, but many languages offer variations such as if-else
, if-elseif-else
, and switch
(or case
) statements. These provide increasingly sophisticated ways to handle multiple potential conditions.
The Fundamental if
Statement
The simplest form is the if
statement. It checks a condition; if the condition evaluates to true
, the code block within the if
statement is executed. If the condition is false
, the code block is skipped.
int age = 25;
if (age >= 18) {
System.out.println("You are an adult.");
}
In this Java example, the condition age >= 18
is checked. Since age
is 25, the condition is true, and the message "You are an adult." is printed.
Expanding with if-else
The if-else
statement allows for handling both true and false conditions. If the if
condition is true, the code within the if
block is executed. Otherwise, the code within the else
block is executed.
temperature = 20
if temperature > 25:
print("It's a hot day!")
else:
print("It's not too hot.")
Here, if temperature
is greater than 25, the first message is printed; otherwise, the second message is printed.
Handling Multiple Conditions with if-elseif-else
When dealing with more than two possibilities, the if-elseif-else
structure (sometimes written as if-else if-else
) becomes necessary. This allows for a cascade of checks, executing the code block corresponding to the first true condition encountered.
let grade = 85;
if (grade >= 90) {
console.log("A");
} else if (grade >= 80) {
console.log("B");
} else if (grade >= 70) {
console.log("C");
} else {
console.log("F");
}
This JavaScript example assigns a letter grade based on the numerical grade
. The code evaluates each condition sequentially until a true condition is found.
The Efficiency of switch
Statements (or Case Statements)
For scenarios involving checking a single variable against multiple discrete values, switch
statements (or their equivalent case
statements in some languages) offer a more efficient and often more readable alternative to a chain of if-elseif-else
statements.
int dayOfWeek = 3;
switch (dayOfWeek) {
case 1:
Console.WriteLine("Monday");
break;
case 2:
Console.WriteLine("Tuesday");
break;
case 3:
Console.WriteLine("Wednesday");
break;
default:
Console.WriteLine("Weekend");
break;
}
This C# code prints the day of the week based on the integer dayOfWeek
. The break
statement is crucial; it prevents the code from "falling through" to the next case. The default
case handles values not explicitly listed.
Nested Conditional Statements
Conditional statements can be nested within each other, allowing for complex decision-making processes. This involves placing an if
, if-else
, or switch
statement inside another conditional statement. While powerful, nested conditionals can become difficult to read and maintain if they become overly complex. Careful planning and clear indentation are crucial for readability.
int age = 15;
int gradeLevel = 10;
if (age >= 18) {
cout << "You are an adult." << endl;
} else {
if (gradeLevel >= 9) {
cout << "You are in high school." << endl;
} else {
cout << "You are in middle school or younger." << endl;
}
}
This C++ example demonstrates nested conditionals to determine a person's status based on age and grade level.
The Importance of Error Handling in Conditional Statements
Robust code anticipates potential errors. Within conditional statements, this often involves checking for invalid input or unexpected conditions before performing operations that could lead to crashes or unexpected behavior.
Scanner scanner = new Scanner(System.in);
int num1, num2;
System.out.print("Enter number 1: ");
num1 = scanner.nextInt();
System.out.print("Enter number 2: ");
num2 = scanner.nextInt();
if (num2 != 0) {
double result = (double) num1 / num2;
System.out.println("Result: " + result);
} else {
System.out.println("Error: Cannot divide by zero.");
}
This Java example demonstrates error handling by explicitly checking if the denominator is zero before performing division. This prevents a runtime error.
Conditional Operators (Ternary Operator)
Many languages offer a concise way to express simple conditional logic using a ternary operator. It's a shorthand for a simple if-else
statement.
age = 20
status = "adult" if age >= 18 else "minor"
print(status) # Output: adult
This Python example uses the ternary operator to assign "adult" or "minor" to the status
variable based on the value of age
.
Boolean Logic within Conditional Statements
Conditional statements often involve complex boolean expressions using logical operators like AND
(&&
or &
), OR
(||
or |
), and NOT
(!
). Mastering these operators is crucial for creating sophisticated conditions.
int age = 22;
bool hasLicense = true;
if (age >= 18 && hasLicense) {
cout << "You can drive." << endl;
}
This C++ example uses AND
to ensure both conditions (age >= 18 and hasLicense) are true before allowing driving.
Short-Circuiting in Boolean Expressions
Some languages implement short-circuiting in boolean expressions. This means that if the outcome of a boolean expression can be determined from the first part of the expression, the remaining parts are not evaluated. This can be important for efficiency and error prevention. For example, in A && B
, if A is false, B is not evaluated. Similarly, in A || B
, if A is true, B is not evaluated.
Common Mistakes to Avoid
- Incorrect Indentation: Improper indentation can lead to logical errors and make your code hard to read.
- Confusing
=
and==
: Using=
(assignment) instead of==
(comparison) is a frequent source of bugs. - Overly Complex Nested Conditionals: Excessive nesting makes code difficult to understand and maintain. Refactor complex logic into smaller, more manageable functions.
- Ignoring Error Handling: Neglecting error handling can lead to crashes or unexpected behavior.
Conclusion:
Mastering conditional statements is essential for building dynamic and responsive applications. By understanding the nuances of if
, if-else
, if-elseif-else
, switch
statements, nested conditionals, error handling, and boolean logic, developers can create efficient and robust programs capable of handling a wide range of scenarios and inputs. Remember to prioritize code readability and maintainability, even when dealing with complex conditional logic. Regular code reviews and testing are vital to ensure the correctness and robustness of your conditional statements. By applying these principles, you can develop high-quality, reliable software.
Latest Posts
Latest Posts
-
A Solution With A Ph Of 6 Is
Apr 23, 2025
-
Which Of The Following Is Not True Regarding Ethics
Apr 23, 2025
-
Calculate The Density Of Co2 Gas At Stp
Apr 23, 2025
-
Average Velocity From Velocity Time Graph
Apr 23, 2025
-
Sphere Has How Many Faces Edges And Vertices
Apr 23, 2025
Related Post
Thank you for visiting our website which covers about Under Which Of The Following Conditions . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.