Reverse List Python Without Inbuilt Function
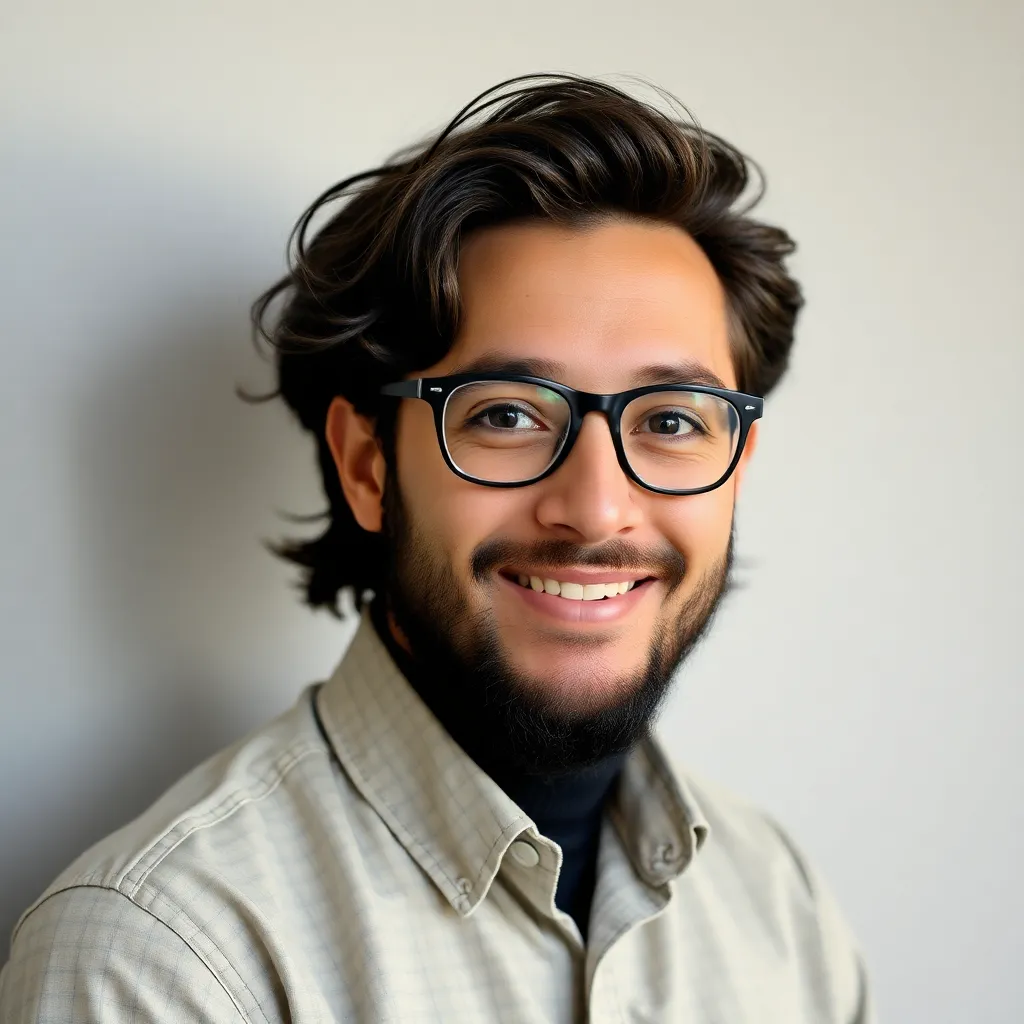
News Leon
Mar 18, 2025 · 6 min read
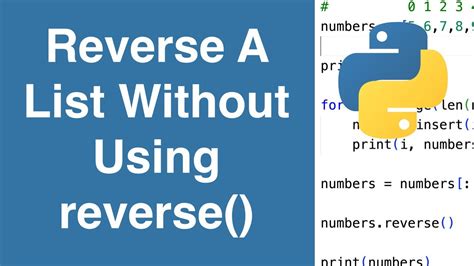
Table of Contents
Reverse a List in Python Without Using Built-in Functions
Reversing a list is a fundamental operation in programming. While Python offers convenient built-in functions like reverse()
and slicing ([::-1]
), understanding how to reverse a list without these tools provides valuable insight into list manipulation and algorithm design. This comprehensive guide explores several methods to achieve this, comparing their efficiency and showcasing practical implementations.
Why Reverse a List Manually?
Before diving into the techniques, let's address the "why." While built-in functions are efficient and concise, manually reversing a list offers several benefits:
- Deep Understanding: Implementing reversal algorithms helps you grasp the underlying mechanics of list manipulation and memory management. This knowledge is invaluable for tackling more complex data structures and algorithms.
- Problem-Solving Skills: Designing your own solution strengthens your problem-solving abilities and forces you to consider different approaches and their trade-offs.
- Interview Preparation: Manually reversing a list is a common interview question, testing your coding skills and algorithmic thinking.
- Customization: In specific scenarios, you might need more control over the reversal process than built-in functions provide. For instance, you might need to perform additional operations during the reversal.
Methods for Reversing a List in Python
We'll delve into several effective methods to reverse a list without relying on Python's built-in functions:
1. Using a for
loop and append()
This is perhaps the most intuitive approach. We iterate through the original list from the end to the beginning and append each element to a new list.
def reverse_list_append(original_list):
"""Reverses a list using a for loop and append().
Args:
original_list: The list to be reversed.
Returns:
A new list containing the reversed elements.
"""
reversed_list = []
for i in range(len(original_list) - 1, -1, -1):
reversed_list.append(original_list[i])
return reversed_list
my_list = [1, 2, 3, 4, 5]
reversed_list = reverse_list_append(my_list)
print(f"Original list: {my_list}")
print(f"Reversed list: {reversed_list}")
Explanation:
- We initialize an empty list called
reversed_list
. - The
for
loop iterates through the indices oforiginal_list
in reverse order, starting from the last element (len(original_list) - 1
) and going down to the first element (index 0). The step of -1 ensures reverse iteration. - In each iteration, the element at the current index is appended to
reversed_list
. - Finally, the
reversed_list
is returned.
This method is straightforward and easy to understand. However, it creates a new list, which might be less efficient in terms of memory usage for very large lists compared to in-place reversal methods.
2. Using a for
loop and insertion (insert()
)
This method modifies the original list directly, avoiding the creation of a new list. We iterate from the end of the list and insert elements at the beginning.
def reverse_list_insert(original_list):
"""Reverses a list in-place using a for loop and insert().
Args:
original_list: The list to be reversed. Modified in-place.
"""
for i in range(len(original_list) - 1, -1, -1):
original_list.insert(0, original_list.pop(i))
my_list = [1, 2, 3, 4, 5]
reverse_list_insert(my_list)
print(f"Reversed list (in-place): {my_list}")
Explanation:
- The
for
loop iterates through the indices in reverse order. - Inside the loop,
original_list.pop(i)
removes the element at indexi
and returns it. original_list.insert(0, element)
inserts the removed element at the beginning of the list.
This method is more memory-efficient as it doesn't create a new list. However, insert(0)
has a time complexity of O(n) because it shifts all existing elements. This makes it less efficient than some other methods for very large lists.
3. Using Two Pointers (In-place Reversal)
This highly efficient method uses two pointers, one at the beginning and one at the end of the list. We swap the elements pointed to by these pointers and move the pointers towards the center.
def reverse_list_pointers(original_list):
"""Reverses a list in-place using two pointers.
Args:
original_list: The list to be reversed. Modified in-place.
"""
left = 0
right = len(original_list) - 1
while left < right:
original_list[left], original_list[right] = original_list[right], original_list[left]
left += 1
right -= 1
my_list = [1, 2, 3, 4, 5]
reverse_list_pointers(my_list)
print(f"Reversed list (in-place): {my_list}")
Explanation:
left
andright
pointers are initialized to the beginning and end of the list, respectively.- The
while
loop continues as long asleft
is less thanright
. - Inside the loop, simultaneous assignment
original_list[left], original_list[right] = original_list[right], original_list[left]
swaps the elements atleft
andright
indices. left
andright
pointers are moved towards the center.
This method is highly efficient with a time complexity of O(n) and a space complexity of O(1) (in-place). It's generally the preferred method for reversing lists in terms of performance.
4. Using Recursion
Recursion offers an elegant, albeit less efficient, way to reverse a list.
def reverse_list_recursive(original_list):
"""Reverses a list using recursion.
Args:
original_list: The list to be reversed.
Returns:
A new list containing the reversed elements.
"""
if not original_list:
return []
else:
return [original_list[-1]] + reverse_list_recursive(original_list[:-1])
my_list = [1, 2, 3, 4, 5]
reversed_list = reverse_list_recursive(my_list)
print(f"Reversed list (recursive): {reversed_list}")
Explanation:
- The base case is an empty list, which returns an empty list.
- Otherwise, the function recursively calls itself with the list excluding the last element (
original_list[:-1]
). - The last element (
original_list[-1]
) is prepended to the result of the recursive call.
While elegant, recursion can be less efficient than iterative methods due to function call overhead. It also has a higher space complexity due to the recursive call stack. For extremely large lists, it might lead to stack overflow errors.
Comparison of Methods
Method | Time Complexity | Space Complexity | In-place | Memory Efficiency | Ease of Understanding |
---|---|---|---|---|---|
append() |
O(n) | O(n) | No | Low | High |
insert() |
O(n^2) | O(1) | Yes | High | Medium |
Two Pointers | O(n) | O(1) | Yes | High | Medium |
Recursion | O(n) | O(n) | No | Low | Medium |
Choosing the Right Method
The optimal method depends on your priorities:
- For simplicity and readability, the
append()
method is a good choice, although it's not the most memory-efficient. - For memory efficiency and in-place modification, the two-pointers method is generally preferred, offering excellent performance.
- The
insert()
method provides in-place modification, but its quadratic time complexity makes it unsuitable for large lists. - Recursion provides an elegant solution but is less efficient than iterative methods for large datasets.
This comprehensive exploration provides a deep dive into reversing lists in Python without using built-in functions. Understanding these methods enhances your programming skills and allows you to choose the most appropriate technique for different scenarios, optimizing for efficiency and readability. Remember to consider the trade-offs between time complexity, space complexity, and in-place modification when making your selection.
Latest Posts
Latest Posts
-
30 Is What Percent Of 48
Mar 18, 2025
-
What Is The Only Language That A Computer Can Understand
Mar 18, 2025
-
Word That Has Two Different Meanings
Mar 18, 2025
-
Is Soil A Homogeneous Or Heterogeneous Mixture
Mar 18, 2025
-
What Does Isinstance Do In Python
Mar 18, 2025
Related Post
Thank you for visiting our website which covers about Reverse List Python Without Inbuilt Function . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.