Python Program For Addition Of Two Numbers
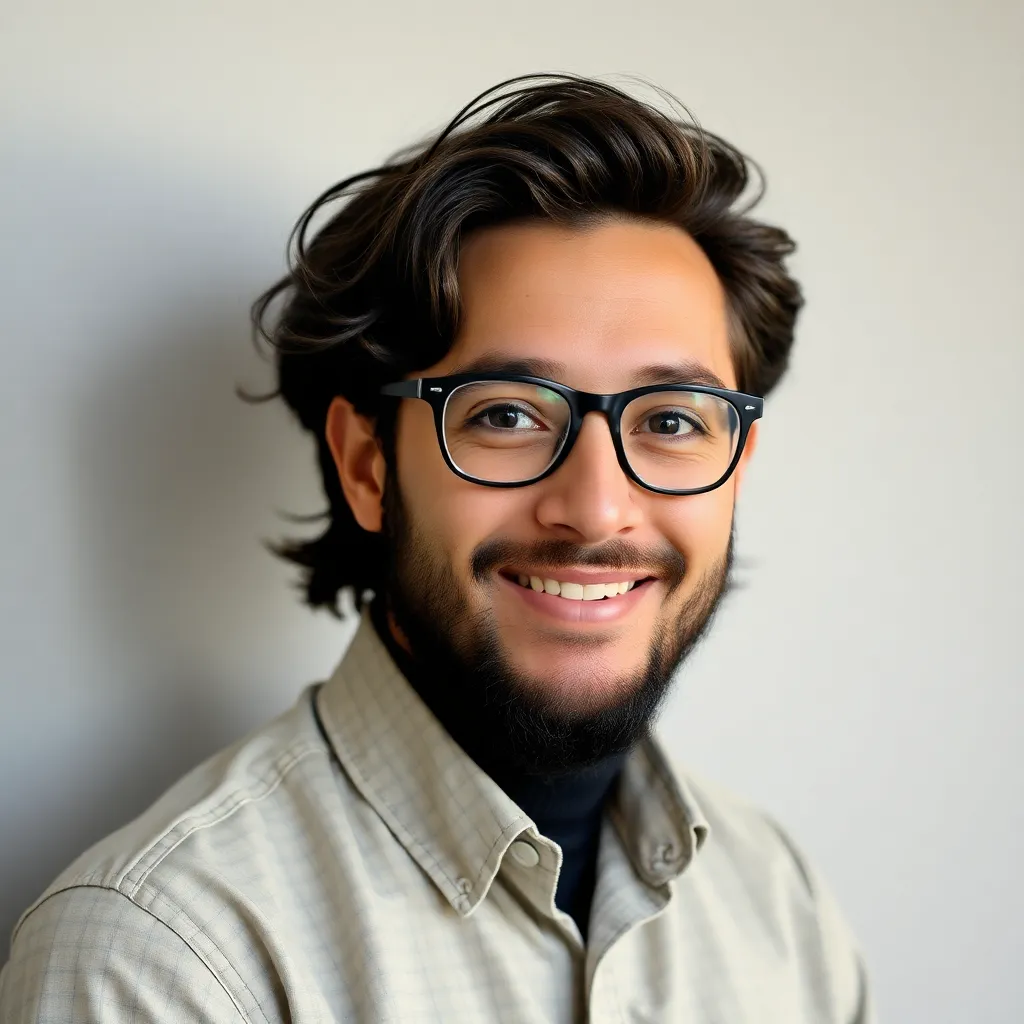
News Leon
Apr 13, 2025 · 5 min read

Table of Contents
Python Program for Addition of Two Numbers: A Comprehensive Guide
Python, renowned for its readability and versatility, offers a straightforward approach to even the most fundamental programming tasks. This article delves deep into creating a Python program for adding two numbers, exploring various methods, addressing potential challenges, and enhancing the program's functionality and robustness. We'll cover everything from basic input-output to error handling and advanced techniques. This comprehensive guide will equip you with a solid understanding of fundamental Python programming concepts and best practices.
The Basic Addition Program
The most elementary way to add two numbers in Python is using the +
operator. This involves taking user input, converting it to a numerical data type, performing the addition, and then displaying the result.
# Get input from the user
num1 = input("Enter the first number: ")
num2 = input("Enter the second number: ")
# Convert input strings to numbers (handling potential errors)
try:
num1 = float(num1)
num2 = float(num2)
# Perform the addition
sum = num1 + num2
# Display the result
print("The sum of", num1, "and", num2, "is:", sum)
except ValueError:
print("Invalid input. Please enter numbers only.")
This program uses a try-except
block to gracefully handle potential ValueError
exceptions that might arise if the user inputs non-numeric data. This error handling is crucial for creating robust and user-friendly applications. Error handling is a vital aspect of software development, ensuring your program doesn't crash unexpectedly.
Enhancing the Program: Function Definition
To improve code organization and reusability, we can encapsulate the addition logic within a function. This makes the code more modular and easier to maintain.
def add_numbers(x, y):
"""This function adds two numbers and returns the sum."""
try:
return float(x) + float(y)
except ValueError:
return "Invalid input. Please enter numbers only."
# Get input from the user
num1 = input("Enter the first number: ")
num2 = input("Enter the second number: ")
# Call the function and display the result
result = add_numbers(num1, num2)
print("The sum is:", result)
The add_numbers
function takes two arguments (x
and y
) and returns their sum. The function also includes error handling, returning an error message if the input is invalid. This structured approach makes the code cleaner and easier to understand. Functions are fundamental building blocks of modular and maintainable code.
Handling Multiple Numbers: Using Lists and Loops
What if we need to add more than just two numbers? We can use lists and loops to handle an arbitrary number of inputs.
def add_multiple_numbers(numbers):
"""This function adds multiple numbers provided in a list."""
total = 0
try:
for number in numbers:
total += float(number)
return total
except ValueError:
return "Invalid input. Please enter numbers only."
# Get input from the user (multiple numbers separated by spaces)
numbers_str = input("Enter numbers separated by spaces: ")
numbers = numbers_str.split()
# Call the function and display the result
result = add_multiple_numbers(numbers)
print("The sum is:", result)
This improved version takes a list of numbers as input, iterates through the list, and adds each number to the total
. The split()
method is used to efficiently parse the user's space-separated input. Utilizing lists and loops enhances the program's flexibility and scalability.
Advanced Techniques: Using Arbitrary Arguments (*args)
For even greater flexibility, we can use the *args
syntax to allow the function to accept any number of arguments without explicitly defining them.
def add_numbers_args(*args):
"""This function adds an arbitrary number of numbers."""
total = 0
try:
for number in args:
total += float(number)
return total
except ValueError:
return "Invalid input. Please enter numbers only."
# Example usage
result1 = add_numbers_args(10, 20, 30)
result2 = add_numbers_args(5, 15, 25, 35, 45)
print("Sum 1:", result1)
print("Sum 2:", result2)
The *args
parameter allows the add_numbers_args
function to accept any number of positional arguments, making it extremely versatile. This technique demonstrates powerful Python features for handling variable numbers of inputs.
Incorporating User Input Validation
To make the program even more robust, we can enhance the input validation. We can check for empty input, non-numeric characters, and even specific ranges of numbers depending on the application requirements.
def add_numbers_validated(*args):
"""This function adds numbers with enhanced input validation."""
total = 0
for arg in args:
try:
num = float(arg)
if not isinstance(num, (int, float)):
raise ValueError("Invalid Input: Must be a Number")
total += num
except ValueError as e:
return f"Error: {e}"
return total
#Example Usage with validation
result1 = add_numbers_validated("10", 20, 30) #Valid
result2 = add_numbers_validated("abc", 20) #Invalid
result3 = add_numbers_validated(10, 20, "30.5") #Valid
print("Result 1:", result1)
print("Result 2:", result2)
print("Result 3:", result3)
This version adds explicit type checking to ensure that input is indeed a number before proceeding. This improves the error handling significantly, making the code more resilient to unexpected inputs.
Extending Functionality: Complex Numbers
Python readily handles complex numbers. We can extend our addition function to accommodate complex number inputs.
def add_complex_numbers(x, y):
"""This function adds two complex numbers."""
try:
return complex(x) + complex(y)
except ValueError:
return "Invalid input. Please enter numbers (real or complex)."
# Example usage with complex numbers
complex_sum = add_complex_numbers("2+3j", "4-1j")
print("Sum of complex numbers:", complex_sum)
This demonstrates Python's ability to handle complex mathematical operations seamlessly.
Conclusion: Building a Robust Python Addition Program
This comprehensive guide has shown how to build a simple Python program for adding two numbers and progressively enhance it through function definition, efficient handling of multiple inputs, robust error handling, and advanced techniques like *args
and complex number support. Each improvement strengthens the program's resilience, readability, and maintainability. Remember, mastering these fundamental concepts is crucial for building more complex and sophisticated Python applications. The key takeaway is the iterative process of refinement, adding features and robustness layer by layer, to create clean, efficient, and reliable code. This approach is fundamental to good software engineering practices.
Latest Posts
Related Post
Thank you for visiting our website which covers about Python Program For Addition Of Two Numbers . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.