Python Convert Unix Timestamp To Datetime
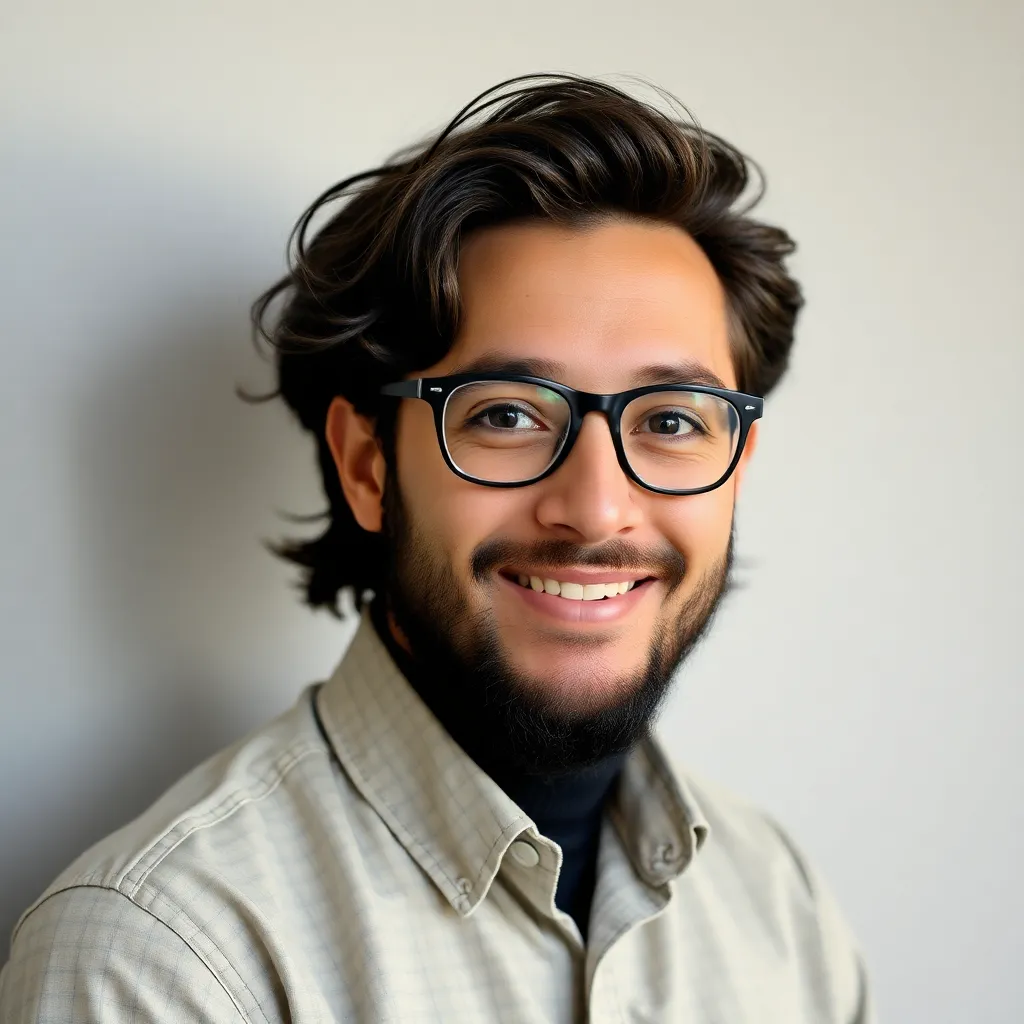
News Leon
Mar 12, 2025 · 5 min read

Table of Contents
Python: Converting Unix Timestamps to Datetime Objects – A Comprehensive Guide
Unix timestamps, representing the number of seconds passed since January 1, 1970, are a ubiquitous format for storing and exchanging time information. However, they aren't very human-readable. This comprehensive guide dives deep into the art of converting these numerical representations into more user-friendly datetime objects in Python, covering various scenarios and best practices. We'll equip you with the knowledge and techniques to smoothly handle timestamp conversions in your Python projects.
Understanding Unix Timestamps and Datetime Objects
Before we jump into the conversion process, let's establish a clear understanding of the two key players:
Unix Timestamp: A single integer representing the number of seconds that have elapsed since the Unix epoch (January 1, 1970, at 00:00:00 Coordinated Universal Time (UTC)).
Datetime Object: A Python object (from the datetime
module) that encapsulates date and time information in a structured and easily manipulated format. It provides attributes for year, month, day, hour, minute, second, and more, enabling various date and time-related operations.
Core Python Modules for Timestamp Conversion
Python offers several powerful modules to facilitate the conversion:
datetime
: The core module for working with dates and times. It provides thedatetime
class and related functionalities.time
: Provides time-related functions, including thelocaltime()
function which converts a Unix timestamp to a time tuple.
Converting Unix Timestamps to Datetime Objects: The Fundamental Methods
The most straightforward way to convert a Unix timestamp to a datetime object is using the datetime.fromtimestamp()
method.
import datetime
unix_timestamp = 1678886400 # Example timestamp
datetime_object = datetime.datetime.fromtimestamp(unix_timestamp)
print(datetime_object) # Output: 2023-03-15 00:00:00
This single line of code elegantly handles the conversion. The output shows the equivalent datetime object, clearly displaying the year, month, day, hour, minute, and second.
Handling Timezones: The fromtimestamp()
method, by default, assumes the timestamp is in UTC. If your timestamp represents a different timezone, you need to adjust accordingly using the pytz
library (or similar timezone-handling libraries).
import datetime
import pytz
unix_timestamp = 1678886400
timezone = pytz.timezone('America/New_York')
datetime_object_utc = datetime.datetime.utcfromtimestamp(unix_timestamp)
datetime_object_ny = timezone.localize(datetime_object_utc)
print(datetime_object_ny) # Output will reflect the adjusted timezone
Advanced Techniques and Error Handling
While the basic method is efficient, let's explore scenarios demanding more robust handling:
1. Handling Potential Errors: Unix timestamps can be invalid (e.g., negative values). Adding error handling ensures graceful management:
import datetime
def convert_timestamp(timestamp):
try:
return datetime.datetime.fromtimestamp(timestamp)
except (OSError, ValueError, OverflowError) as e:
print(f"Error converting timestamp: {e}")
return None
timestamp = 1678886400
dt = convert_timestamp(timestamp)
print(dt)
invalid_timestamp = -100
dt = convert_timestamp(invalid_timestamp)
print(dt) # Output will indicate an error
This improved function includes a try-except
block to capture potential OSError
, ValueError
, or OverflowError
exceptions, returning None
if the conversion fails.
2. Precision Control: For more granular control over the output format, consider using strftime()
to customize the datetime string representation:
import datetime
unix_timestamp = 1678886400
datetime_object = datetime.datetime.fromtimestamp(unix_timestamp)
formatted_datetime = datetime_object.strftime("%Y-%m-%d %H:%M:%S %Z%z") #Customize format
print(formatted_datetime)
This allows you to tailor the output to your exact needs. Explore the strftime()
documentation for a comprehensive list of format codes.
3. Working with Milliseconds or Microseconds: Some timestamps are expressed in milliseconds or microseconds instead of seconds. Adjust accordingly:
import datetime
milliseconds_timestamp = 1678886400000 # Timestamp in milliseconds
datetime_object = datetime.datetime.fromtimestamp(milliseconds_timestamp / 1000)
print(datetime_object)
microseconds_timestamp = 1678886400000000 # Timestamp in microseconds
datetime_object = datetime.datetime.fromtimestamp(microseconds_timestamp / 1000000)
print(datetime_object)
4. Leveraging the time
module: The time
module offers an alternative approach, converting the timestamp to a time tuple first:
import time
import datetime
unix_timestamp = 1678886400
time_tuple = time.localtime(unix_timestamp)
datetime_object = datetime.datetime(*time_tuple[:6]) # unpack the time tuple
print(datetime_object)
This approach uses time.localtime()
to get a time tuple, then constructs a datetime
object from its components. Note the slicing [:6]
to exclude the weekday and year day information.
Optimizing for Performance in Large Datasets
When dealing with a large number of timestamps, efficiency becomes crucial. Vectorized operations using NumPy can significantly speed up the conversion process:
import numpy as np
import datetime
timestamps = np.array([1678886400, 1678972800, 1679059200]) # Example array of timestamps
datetime_objects = np.array([datetime.datetime.fromtimestamp(ts) for ts in timestamps])
print(datetime_objects)
While list comprehensions can be efficient, NumPy's vectorized operations generally provide better performance for large datasets.
Real-World Applications and Use Cases
The ability to convert Unix timestamps to datetime objects is fundamental in numerous applications:
- Log File Analysis: Analyzing log files containing timestamps to track events and identify patterns.
- Data Visualization: Preparing data for plotting and visualization libraries like Matplotlib, where datetime objects are essential for creating time-series graphs.
- Database Interactions: Converting timestamps stored in databases into a user-friendly format for display or further processing.
- Web Application Development: Displaying timestamps in a user-friendly format on websites or web applications.
- Scientific Computing: Handling time-stamped data in simulations and scientific experiments.
Conclusion
Converting Unix timestamps to datetime objects is a cornerstone skill for any Python programmer working with time-related data. This guide has covered the foundational methods, advanced techniques for error handling and optimization, and practical applications. Mastering these techniques empowers you to effectively manage and analyze time-stamped data, building robust and efficient Python applications. Remember to choose the method that best suits your specific needs and dataset size, prioritizing error handling and optimization for optimal performance. Remember to consult the official Python documentation for the most up-to-date information and details on these modules and functions.
Latest Posts
Related Post
Thank you for visiting our website which covers about Python Convert Unix Timestamp To Datetime . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.