How To Use Round In Python
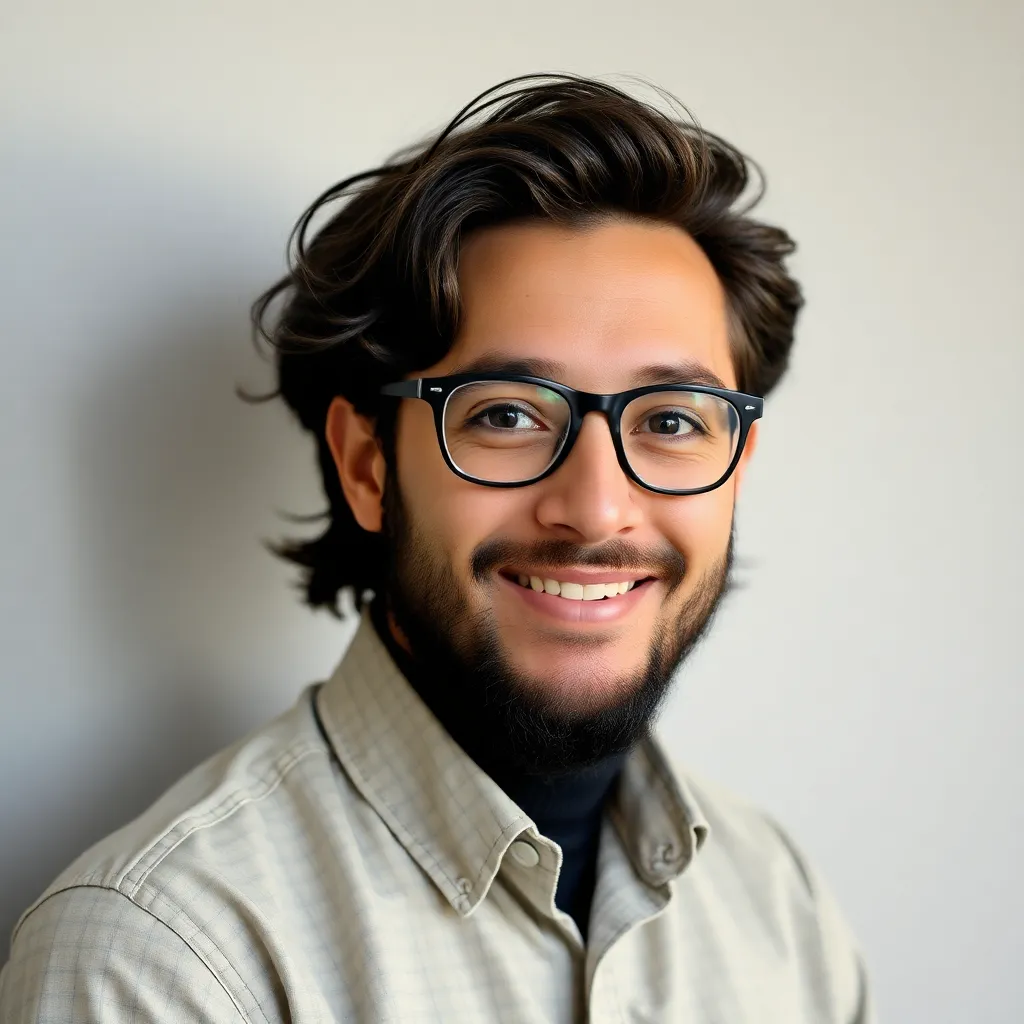
News Leon
Apr 07, 2025 · 5 min read
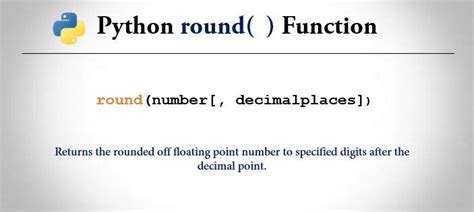
Table of Contents
Mastering Python's round()
Function: A Comprehensive Guide
Python's built-in round()
function is a deceptively simple yet powerful tool for handling floating-point numbers. While its primary purpose seems straightforward – rounding numbers to a specified number of decimal places – a deeper understanding reveals nuances and unexpected behaviors that can significantly impact your code's accuracy and efficiency. This comprehensive guide explores the intricacies of round()
, covering its basic usage, advanced techniques, and potential pitfalls to avoid.
Understanding the Basics: Rounding to the Nearest Integer
At its core, round()
takes a single numerical argument and returns the nearest integer. If the fractional part of the number is exactly 0.5, the function rounds to the nearest even integer. This is known as "banker's rounding" or "round half to even," and it helps minimize bias over many rounding operations.
print(round(3.14)) # Output: 3
print(round(3.5)) # Output: 4
print(round(4.5)) # Output: 4
print(round(5.5)) # Output: 6
print(round(-2.5)) # Output: -2
Notice how round(3.5)
and round(4.5)
yield different results. This is precisely due to banker's rounding. This seemingly subtle detail is crucial for maintaining statistical accuracy in applications involving many rounding operations. It ensures that rounding errors are evenly distributed, avoiding systematic bias towards higher or lower values.
Rounding to a Specified Number of Decimal Places
The round()
function becomes even more versatile when you provide a second argument: the number of decimal places to round to. This argument specifies the precision of the rounding.
print(round(3.14159, 2)) # Output: 3.14
print(round(12.34567, 3)) # Output: 12.346
print(round(0.005, 2)) # Output: 0.01
print(round(99.995, 2)) #Output: 100.0
Here, round(3.14159, 2)
rounds the number to two decimal places, effectively truncating the digits beyond the second decimal point and applying banker's rounding as necessary. Note that this rounding still follows the "round half to even" rule.
Handling Negative Decimal Places: Rounding to Tens, Hundreds, etc.
You can even use negative numbers as the second argument to round()
. This allows you to round to multiples of 10, 100, 1000, and so on. This is particularly useful in scenarios where you need to round to significant figures.
print(round(1234, -1)) # Output: 1230 (Rounding to the nearest ten)
print(round(12345, -2)) # Output: 12300 (Rounding to the nearest hundred)
print(round(123456, -3))# Output: 123000 (Rounding to the nearest thousand)
print(round(1234567,-4))# Output: 1230000 (Rounding to nearest ten thousand)
Rounding to tens, hundreds, or thousands can be useful in various contexts like financial reporting, data visualization, and statistical summaries where simplifying numbers while retaining essential information is crucial.
Beyond Integers: Rounding Floats and Scientific Notation
The round()
function isn't limited to integers; it seamlessly handles floating-point numbers and numbers represented in scientific notation.
print(round(1.23456e-5, 3)) # Output: 1.235e-05
print(round(1.2345e10, 2)) # Output: 12345000000.0
The function accurately applies rounding even in scenarios involving very large or very small numbers, preserving the correct magnitude and precision.
Important Considerations: Limitations and Unexpected Behavior
While generally reliable, round()
has some quirks that require careful consideration.
Floating-Point Precision Issues
Floating-point numbers are inherently imprecise due to the limitations of binary representation. This can lead to unexpected results when using round()
.
x = 2.675
print(round(x, 2)) # Output: 2.67 (This might seem counterintuitive!)
While mathematically 2.675
should round up to 2.68
, floating-point representation might store it as a value slightly less than 2.675
. This subtle difference is amplified by round()
's banker's rounding logic.
Rounding to Zero: A Special Case
Rounding very small numbers to a significant number of decimal places can unexpectedly produce zero.
x = 1e-10
print(round(x, 5)) # Output: 0.0
Dealing with Non-Numeric Inputs: Exception Handling
Attempting to use round()
with non-numeric inputs will raise a TypeError
. Robust code should include error handling to gracefully manage such situations.
try:
print(round("3.14"))
except TypeError as e:
print(f"Error: {e}") # Output: Error: type str doesn't define __round__ method
Advanced Techniques: Combining round()
with Other Functions
The power of round()
truly shines when combined with other Python functions and techniques.
Formatting Output: String Formatting and f-strings
To control the visual representation of rounded numbers, use Python's string formatting capabilities, such as f-strings. This provides fine-grained control over decimal places and overall formatting.
x = 123.456789
formatted_number = f"{round(x, 2):.2f}" # Output: 123.46
Working with NumPy Arrays: Vectorized Rounding
When working with NumPy arrays, the round()
function can be applied element-wise, providing efficient vectorized operations:
import numpy as np
arr = np.array([1.234, 5.678, 9.012])
rounded_arr = np.round(arr, 1) # Output: [1.2 5.7 9. ]
Custom Rounding Functions: Extending Functionality
For highly specialized rounding requirements beyond what round()
offers (e.g., rounding to specific increments), consider creating custom functions.
def round_to_nearest_half(num):
return round(num * 2) / 2
print(round_to_nearest_half(2.3)) # Output: 2.5
print(round_to_nearest_half(2.7)) # Output: 2.5
print(round_to_nearest_half(2.2)) # Output: 2.0
print(round_to_nearest_half(2.8)) # Output: 3.0
Conclusion: Mastering the Art of Rounding in Python
The Python round()
function is a fundamental tool for numerical computation, offering versatility and accuracy in various applications. By understanding its core functionality, limitations, and advanced usage patterns, you can write efficient and reliable Python code that effectively handles numerical rounding. Remember to consider floating-point precision issues and to handle potential TypeError
exceptions appropriately for robust and error-free code. Mastering round()
helps elevate your Python skills and empowers you to tackle more complex numerical tasks with confidence. This comprehensive understanding will make you a more effective and efficient Python programmer.
Latest Posts
Latest Posts
-
In Which Continent Is India Located
Apr 07, 2025
-
Which One Of The Following Is A Diprotic Acid
Apr 07, 2025
-
Freezing Point Of Nacl In Water
Apr 07, 2025
-
What Is Dispersion Of Light In Physics
Apr 07, 2025
-
The Odontoid Process Is Found On The
Apr 07, 2025
Related Post
Thank you for visiting our website which covers about How To Use Round In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.