How To Print A Function In Python
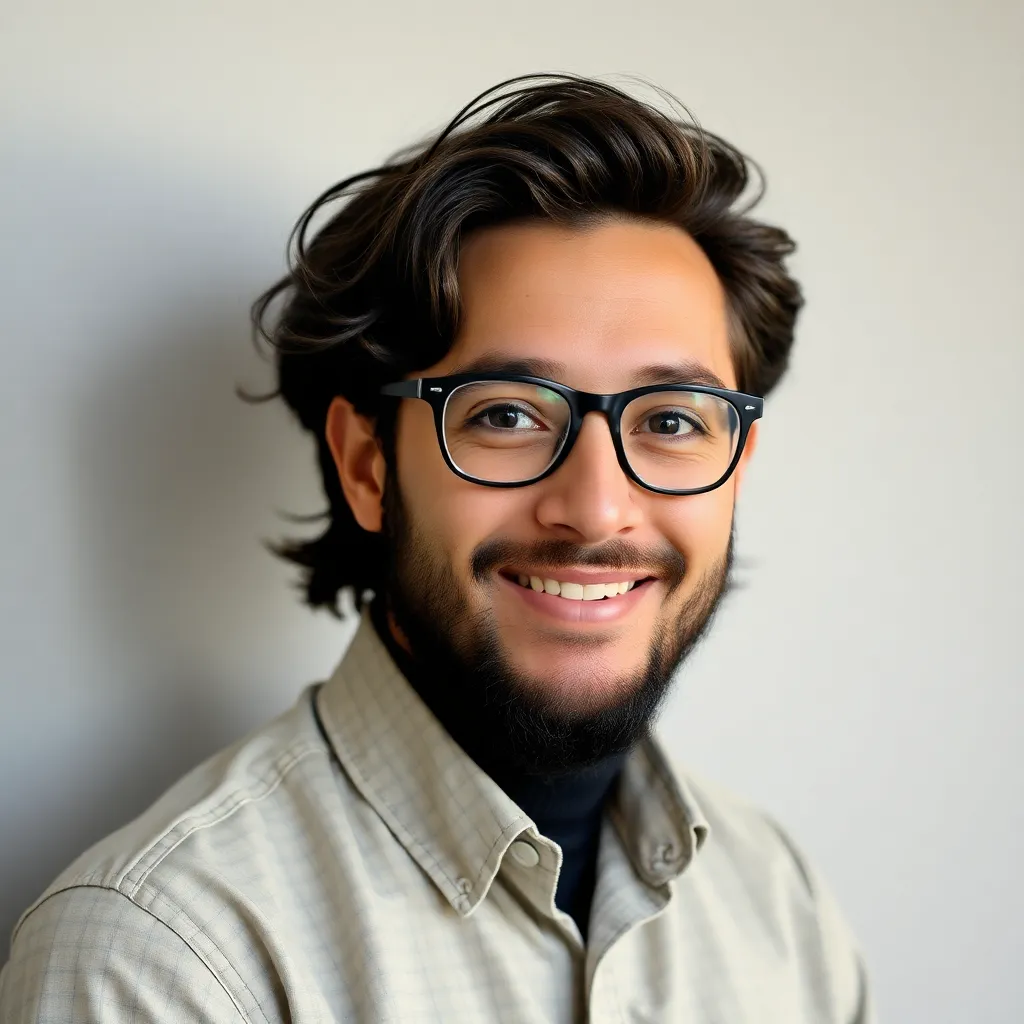
News Leon
Apr 22, 2025 · 5 min read

Table of Contents
How to Print a Function in Python: A Comprehensive Guide
Python, renowned for its readability and versatility, offers several ways to "print" a function. However, the term "print a function" is ambiguous. Are we aiming to display the function's source code, its name, its docstring, its memory address, or its output when called? This comprehensive guide clarifies these distinctions and explores the various techniques available, ensuring you choose the most appropriate method for your needs.
Understanding the Nuances of "Printing" a Function
Before diving into the methods, it's crucial to understand what you actually want to achieve. Different scenarios demand different approaches.
1. Printing the Function's Source Code
This involves displaying the actual Python code that defines the function. This is particularly useful for debugging, code review, or documentation generation.
Method: The simplest method leverages the inspect
module.
import inspect
def my_function(a, b):
"""This function adds two numbers."""
return a + b
print(inspect.getsource(my_function))
This will output the source code of my_function
, including its docstring. Note that this only works if the function is defined in a file or a module that can be inspected. Functions defined interactively in a Python interpreter might not be inspectable in this manner.
Handling Lambda Functions: inspect.getsource
also works with lambda functions:
lambda_func = lambda x: x**2
print(inspect.getsource(lambda_func))
However, the output might not be as visually appealing as for regular functions.
2. Printing the Function's Name
This is a straightforward task using the __name__
attribute of the function object.
def my_function(a, b):
"""This function adds two numbers."""
return a + b
print(my_function.__name__) # Output: my_function
This approach is ideal when you need to identify the function within a larger program or when dynamically working with functions.
3. Printing the Function's Docstring
The docstring provides a concise description of the function's purpose, parameters, and return value. Accessing and printing it enhances code readability and maintainability.
def my_function(a, b):
"""This function adds two numbers.
Args:
a: The first number.
b: The second number.
Returns:
The sum of a and b.
"""
return a + b
print(my_function.__doc__)
This will output the docstring of my_function
. Well-written docstrings are critical for creating self-documenting code.
4. Printing the Function's Memory Address (or ID)
While less common, you might sometimes need to know the function's memory location. Python's id()
function serves this purpose.
def my_function(a, b):
"""This function adds two numbers."""
return a + b
print(id(my_function))
This returns an integer representing the function's memory address. This is primarily useful for low-level debugging or when comparing function objects for identity.
5. Printing the Function's Output
This is the most practical scenario: executing the function and displaying its results.
def my_function(a, b):
"""This function adds two numbers."""
return a + b
result = my_function(5, 3)
print(result) # Output: 8
This method directly displays the value returned by the function. For functions that don't explicitly return a value (implicitly returning None
), printing the result will show None
.
Handling Functions with Side Effects: Some functions primarily perform actions (side effects) instead of returning a value. For example, functions that modify a list in place or print to the console directly. In such cases, the output you see might not be the function's return value, but rather the side effect itself.
Advanced Techniques and Considerations
1. Printing Functions within Classes (Methods)
Functions within classes (methods) are handled similarly, with the addition of needing to call them using an instance of the class.
class MyClass:
def my_method(self, x):
"""This method squares a number."""
return x**2
my_instance = MyClass()
result = my_instance.my_method(4)
print(result) #Output: 16
print(inspect.getsource(MyClass.my_method)) #Prints source code of the method
print(MyClass.my_method.__doc__) #Prints the docstring of the method
print(MyClass.my_method.__name__) #Prints the name of the method
Remember that you can access methods directly through the class using MyClass.my_method
, but calling them requires an instance.
2. Printing Nested Functions
Python supports nested functions (functions defined inside other functions). Printing them works the same as with regular functions, but you must ensure proper scoping.
def outer_function():
def inner_function(x):
return x * 2
return inner_function
inner = outer_function()
print(inspect.getsource(inner))
print(inner(5)) #Output: 10
inspect.getsource
can access the source code of inner_function
even though it's nested.
3. Handling Errors Gracefully
When working with functions dynamically (e.g., from user input or external sources), always implement error handling to prevent crashes.
import inspect
try:
function_name = input("Enter function name: ")
function_to_print = globals()[function_name] #Access function from global namespace. Be cautious with user input here!
print(inspect.getsource(function_to_print))
except KeyError:
print("Function not found.")
except TypeError:
print("Invalid input. Please enter a valid function name.")
except Exception as e:
print(f"An error occurred: {e}")
This example demonstrates how to handle KeyError
(if the function doesn't exist) and TypeError
(if the input is not a valid function name) using a try-except
block. Always include comprehensive error handling to robustly manage potential issues.
4. Debugging and Logging
Printing function outputs or source code is a valuable debugging tool. For more sophisticated debugging, consider using Python's logging
module. Logging provides a structured way to record messages during program execution, useful for tracing function calls and identifying errors.
import logging
logging.basicConfig(level=logging.DEBUG) # Set logging level to DEBUG to see all messages
def my_function(a, b):
logging.debug(f"Entering my_function with a={a}, b={b}")
result = a + b
logging.debug(f"Result: {result}")
return result
my_function(10,5)
This enhances debugging by providing timestamps and context information.
Conclusion: Choosing the Right Approach
The "best" way to "print a function" in Python depends entirely on your goal. If you need the function's source code, use inspect.getsource
. For its name, use __name__
. For its documentation, access __doc__
. To display the function's output, simply call the function and print the returned value. Remember to always handle potential errors and consider using logging for more advanced debugging scenarios. By understanding these different approaches and applying them appropriately, you can effectively manage and understand your Python functions, leading to cleaner, more efficient, and debuggable code. Consistent application of these methods contributes significantly to improving overall code quality and maintainability. Remember to consider best practices such as adding comprehensive docstrings and utilizing exception handling in your projects for enhanced robustness.
Latest Posts
Latest Posts
-
What Percentage Of The Cells Are In Interphase
Apr 22, 2025
-
A Threadlike Structure Of Dna That Carries Genes
Apr 22, 2025
-
During Diffusion In Which Way Do Molecules Move
Apr 22, 2025
-
Do Single Celled Organisms Like The Amoeba Maintain Homeostasis
Apr 22, 2025
-
60 Of 45 Is What Number
Apr 22, 2025
Related Post
Thank you for visiting our website which covers about How To Print A Function In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.