How To Multiply Matrices In Python
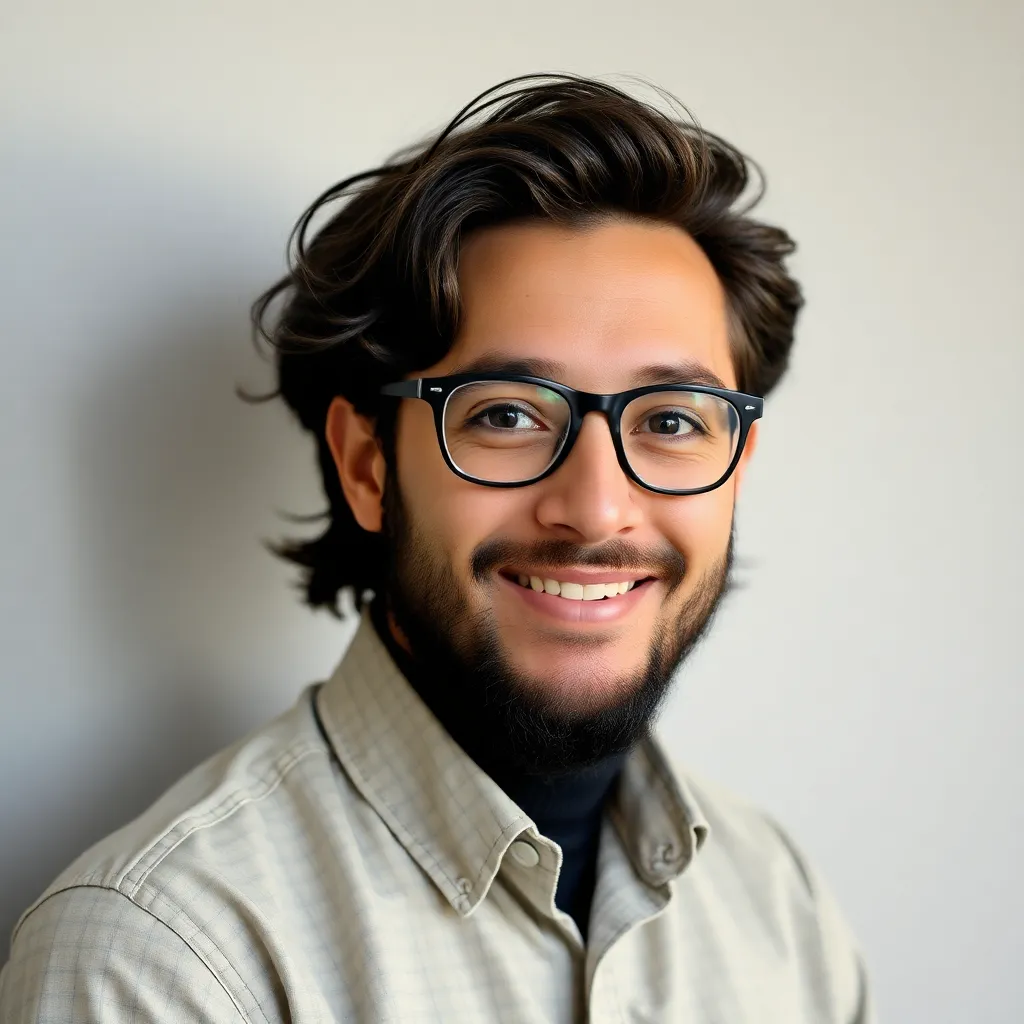
News Leon
Apr 24, 2025 · 6 min read

Table of Contents
How to Multiply Matrices in Python: A Comprehensive Guide
Matrix multiplication is a fundamental operation in linear algebra with widespread applications in various fields like computer graphics, machine learning, and data science. Python, with its rich ecosystem of libraries like NumPy, offers efficient ways to perform matrix multiplication. This comprehensive guide delves into the intricacies of matrix multiplication in Python, covering different methods, their efficiency, and practical examples.
Understanding Matrix Multiplication
Before diving into Python implementations, let's revisit the core concept of matrix multiplication. Matrix multiplication is not element-wise multiplication; instead, it's a more complex operation defined as follows:
Rule: The number of columns in the first matrix must equal the number of rows in the second matrix. The resulting matrix will have the number of rows from the first matrix and the number of columns from the second matrix.
Let's consider two matrices, A and B:
Matrix A (m x n):
[a11, a12, ..., a1n]
[a21, a22, ..., a2n]
...
[am1, am2, ..., amn]
Matrix B (n x p):
[b11, b12, ..., b1p]
[b21, b22, ..., b2p]
...
[bn1, bn2, ..., bnp]
The resulting matrix C (m x p) is calculated as:
cij = Σ(aik * bkj) for k = 1 to n
where cij
represents the element in the i-th row and j-th column of matrix C. This means each element in the resulting matrix is the dot product of a row from the first matrix and a column from the second matrix.
Python Implementation using Nested Loops
The most basic approach involves using nested loops to implement the matrix multiplication algorithm. This method is conceptually straightforward but can be computationally expensive for large matrices.
def matrix_multiply_nested_loops(A, B):
"""
Multiplies two matrices using nested loops.
Args:
A: The first matrix (list of lists).
B: The second matrix (list of lists).
Returns:
The resulting matrix (list of lists), or None if multiplication is not possible.
"""
rows_A = len(A)
cols_A = len(A[0])
rows_B = len(B)
cols_B = len(B[0])
if cols_A != rows_B:
print("Error: Incompatible matrix dimensions for multiplication.")
return None
C = [[0 for row in range(cols_B)] for col in range(rows_A)] # Initialize the result matrix
for i in range(rows_A):
for j in range(cols_B):
for k in range(cols_A):
C[i][j] += A[i][k] * B[k][j]
return C
# Example usage:
matrix_A = [[1, 2], [3, 4]]
matrix_B = [[5, 6], [7, 8]]
result_matrix = matrix_multiply_nested_loops(matrix_A, matrix_B)
print(f"Resultant Matrix:\n{result_matrix}")
This code first checks for compatibility of matrix dimensions. If compatible, it initializes a result matrix filled with zeros and then iterates through rows and columns performing the dot product calculation as defined above. While functional, this approach becomes inefficient for larger matrices due to its cubic time complexity (O(n³)).
Utilizing NumPy for Efficient Matrix Multiplication
NumPy is a powerful Python library specifically designed for numerical computations, including efficient matrix operations. NumPy's dot()
function provides a highly optimized way to perform matrix multiplication.
import numpy as np
def matrix_multiply_numpy(A, B):
"""
Multiplies two matrices using NumPy's dot() function.
Args:
A: The first matrix (NumPy array).
B: The second matrix (NumPy array).
Returns:
The resulting matrix (NumPy array), or None if multiplication is not possible.
"""
try:
C = np.dot(A, B)
return C
except ValueError as e:
print(f"Error during NumPy matrix multiplication: {e}")
return None
# Example usage:
matrix_A_np = np.array([[1, 2], [3, 4]])
matrix_B_np = np.array([[5, 6], [7, 8]])
result_matrix_np = matrix_multiply_numpy(matrix_A_np, matrix_B_np)
print(f"Resultant Matrix (NumPy):\n{result_matrix_np}")
This NumPy implementation is significantly more efficient than the nested loop approach, leveraging optimized C code under the hood. It handles potential errors gracefully using a try-except
block and is considerably faster, especially for large matrices. The time complexity is typically O(n²).
@ operator for Matrix Multiplication
Python 3.5 and later versions introduced the @
operator as a more concise and readable way to perform matrix multiplication, particularly when using NumPy arrays.
import numpy as np
matrix_A_np = np.array([[1, 2], [3, 4]])
matrix_B_np = np.array([[5, 6], [7, 8]])
result_matrix_at = matrix_A_np @ matrix_B_np
print(f"Resultant Matrix (@ operator):\n{result_matrix_at}")
The @
operator provides a cleaner syntax for matrix multiplication, making the code more readable and intuitive. Under the hood, it still relies on NumPy's optimized dot()
function for efficient computation.
Handling Different Matrix Types and Error Conditions
The code examples above demonstrated matrix multiplication with numerical data. However, it’s crucial to handle various scenarios and potential errors:
-
Non-numerical data: While NumPy primarily works with numerical data, you might need to handle matrices with other data types. In such cases, ensure appropriate type conversions or use alternative methods depending on the data type.
-
Non-square matrices: The code should explicitly handle cases where matrices are not square (number of rows ≠ number of columns). The nested loop approach already includes this check, while NumPy's
dot()
function automatically handles this constraint by raising aValueError
if incompatible dimensions are provided. -
Empty matrices: Your functions should gracefully handle empty matrices, either returning an empty matrix or indicating an error appropriately.
-
Singular matrices: When dealing with square matrices, there might be situations where the matrix is singular (its determinant is zero). This can lead to issues in some calculations, so it's good practice to include checks or employ techniques that handle these cases.
Advanced Matrix Operations and Libraries
Beyond basic multiplication, Python offers extensive libraries for more advanced matrix operations:
-
SciPy: This library builds upon NumPy and provides additional functionalities like linear algebra solvers, eigenvalue decomposition, and more sophisticated matrix operations.
-
Eigen: This is a powerful C++ library with excellent performance for linear algebra. You can integrate it with Python using libraries like
pybind11
. -
BLAS and LAPACK: These are highly optimized linear algebra libraries (often written in Fortran or C) used as backends for NumPy and SciPy. Understanding their role helps in optimizing matrix operations for maximum performance.
Choosing the right library depends on the complexity of your matrix operations and performance requirements. For most common matrix multiplication tasks, NumPy's dot()
function or the @
operator are sufficient. For complex or large-scale computations, consider using SciPy or integrating more specialized libraries for optimal speed and efficiency.
Conclusion
Matrix multiplication is a crucial operation in numerous applications. Python, with its libraries like NumPy and SciPy, offers flexible and efficient methods for handling matrix multiplication. While nested loops provide a basic understanding, leveraging NumPy's capabilities drastically improves performance, particularly for large-scale matrices. Understanding the intricacies of matrix dimensions, error handling, and utilizing advanced libraries are crucial steps to building robust and efficient matrix manipulation code in Python. Remember to choose the most suitable approach based on your project's complexity and performance needs, optimizing for speed and readability. The @
operator provides a concise syntax for matrix multiplication that further enhances the code's clarity. This comprehensive guide provides a solid foundation for anyone working with matrix operations in Python.
Latest Posts
Latest Posts
-
A Semipermeable Membrane Is Placed Between The Following Solutions
Apr 24, 2025
-
Which Of The Following Pancreatic Enzymes Acts On Peptide Bonds
Apr 24, 2025
-
The Noble Gas With The Smallest Atomic Radius
Apr 24, 2025
-
What Is The Value Of Log3 81
Apr 24, 2025
-
As You Move Left To Right On The Periodic Table
Apr 24, 2025
Related Post
Thank you for visiting our website which covers about How To Multiply Matrices In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.