How To Index A Tuple In Python
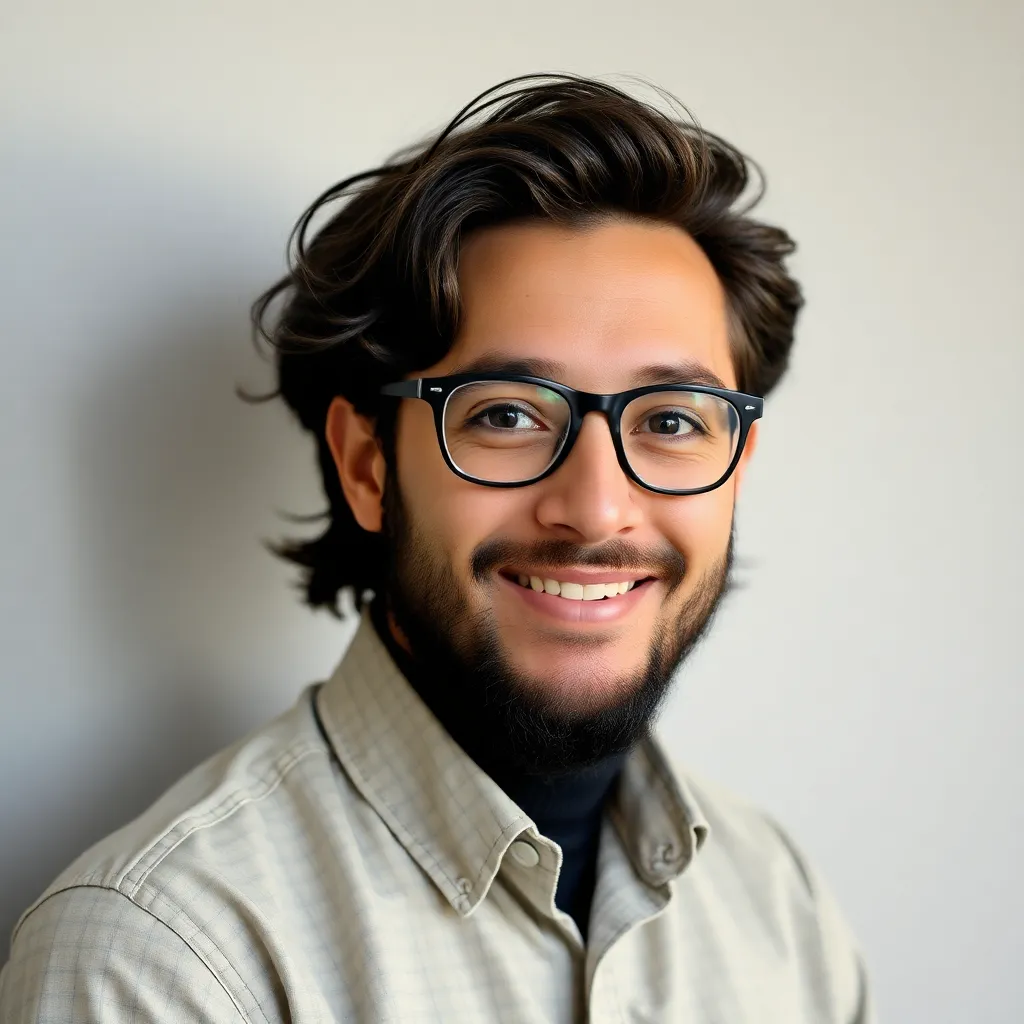
News Leon
Apr 22, 2025 · 5 min read

Table of Contents
How to Index a Tuple in Python: A Comprehensive Guide
Python tuples, immutable sequences of items, are fundamental data structures offering efficient storage and retrieval. Understanding how to index these sequences is crucial for effectively working with Python. This comprehensive guide dives deep into tuple indexing, covering various methods, edge cases, and best practices. We'll explore slicing, negative indexing, and multi-dimensional indexing, equipping you with the skills to confidently manipulate tuples in your programs.
Understanding Tuple Indexing
Before we delve into the specifics, let's establish a foundational understanding. Tuples, like lists and strings, are ordered collections. This means each item occupies a specific position, identified by its index. Python uses zero-based indexing, meaning the first element has an index of 0, the second has an index of 1, and so on.
Let's consider a sample tuple:
my_tuple = ("apple", "banana", "cherry", "date", "elderberry")
"apple"
is at index 0."banana"
is at index 1."cherry"
is at index 2."date"
is at index 3."elderberry"
is at index 4.
Accessing Elements Using Positive Indexing
The most straightforward method of accessing tuple elements is through positive indexing. You simply specify the index within square brackets []
after the tuple variable name:
first_fruit = my_tuple[0] # Accesses "apple"
third_fruit = my_tuple[2] # Accesses "cherry"
print(first_fruit, third_fruit) # Output: apple cherry
This is intuitive and widely used for accessing elements at known positions.
Negative Indexing: Accessing Elements from the End
Python's negative indexing allows you to access elements starting from the end of the tuple. The last element has an index of -1, the second to last is -2, and so on:
last_fruit = my_tuple[-1] # Accesses "elderberry"
second_last_fruit = my_tuple[-2] # Accesses "date"
print(last_fruit, second_last_fruit) # Output: elderberry date
Negative indexing is particularly useful when you need to access elements without knowing their exact position from the beginning.
Slicing Tuples: Extracting Subsequences
Slicing allows you to extract a portion of a tuple, creating a new tuple containing a subsequence. The syntax is tuple[start:stop:step]
:
start
: The index of the first element to include (inclusive). Defaults to 0 if omitted.stop
: The index of the element to stop before (exclusive). Defaults to the length of the tuple if omitted.step
: The increment between indices. Defaults to 1 if omitted.
Let's illustrate:
some_fruits = my_tuple[1:4] # Extracts "banana", "cherry", "date"
print(some_fruits) # Output: ('banana', 'cherry', 'date')
every_other_fruit = my_tuple[::2] # Extracts every other element
print(every_other_fruit) # Output: ('apple', 'cherry', 'elderberry')
reversed_fruits = my_tuple[::-1] # Reverses the tuple
print(reversed_fruits) # Output: ('elderberry', 'date', 'cherry', 'banana', 'apple')
Slicing is a powerful tool for manipulating and extracting specific parts of your tuples.
Handling IndexErrors: Out-of-Bounds Access
Attempting to access an element using an index that's out of range will result in an IndexError
. This is a common error, particularly when working with dynamically sized tuples or when using calculations to determine indices.
try:
invalid_access = my_tuple[5] # Index 5 is out of bounds
except IndexError:
print("Index out of range!")
Always validate your indices to prevent IndexError
exceptions.
Multi-Dimensional Tuples (Nested Tuples): Indexing Techniques
Tuples can contain other tuples, creating nested or multi-dimensional structures. Indexing these requires specifying multiple indices, one for each level of nesting:
nested_tuple = (("a", "b"), ("c", "d"), ("e", "f"))
# Access "b"
element_b = nested_tuple[0][1]
print(element_b) # Output: b
# Access "e"
element_e = nested_tuple[2][0]
print(element_e) # Output: e
# Access "d"
element_d = nested_tuple[1][1]
print(element_d) # Output: d
Each index selects an element from the corresponding level. Remember that the outermost tuple's index comes first, followed by the next inner level, and so on.
Tuple Indexing vs. List Indexing: Key Differences
While tuples and lists share similar indexing mechanisms, their immutability is a critical distinction. You can modify lists in-place using indexing and assignment:
my_list = ["apple", "banana", "cherry"]
my_list[0] = "grape"
print(my_list) # Output: ['grape', 'banana', 'cherry']
Attempting to modify a tuple element using indexing will raise a TypeError
:
try:
my_tuple[0] = "grape"
except TypeError:
print("Tuples are immutable!")
This immutability is a key characteristic of tuples and affects how you interact with them.
Best Practices for Tuple Indexing
- Clear Variable Names: Use descriptive variable names to improve code readability, especially when working with indices.
- Input Validation: Always validate inputs (especially user inputs) to ensure indices are within the bounds of the tuple.
- Error Handling: Implement appropriate
try-except
blocks to handle potentialIndexError
exceptions gracefully. - Use Slicing Effectively: Utilize slicing to extract subsequences, making your code concise and efficient.
- Avoid Unnecessary Indexing: For simple access to the first or last elements, negative indexing can be more readable.
Advanced Indexing Scenarios and Techniques
While basic indexing is straightforward, more complex scenarios might require additional techniques:
-
Conditional Indexing: Using boolean indexing and list comprehensions (though not directly applicable to tuples, as they are immutable), you can select elements based on certain conditions. This is often done with lists and then converted to tuples if needed.
-
Iterating with Indices: You can iterate through a tuple using a
for
loop andenumerate
to access both elements and their indices.
for index, element in enumerate(my_tuple):
print(f"Element at index {index}: {element}")
- Working with Large Tuples: For extremely large tuples, consider optimizing access patterns to minimize computation. If possible, pre-calculate indices or use more efficient data structures for specific tasks.
Conclusion
Mastering tuple indexing is crucial for proficient Python programming. Understanding positive and negative indexing, slicing, and handling multi-dimensional structures empowers you to effectively manipulate and extract data from tuples. Remember to prioritize code readability, error handling, and efficient indexing techniques, especially when working with larger or nested tuples. By applying these strategies, your Python code will be more robust, maintainable, and efficient. This comprehensive guide provides a solid foundation for your work with Python tuples, equipping you to tackle even the most complex indexing challenges. Remember to practice regularly, experiment with different scenarios, and consult the Python documentation for more detailed information.
Latest Posts
Latest Posts
-
Which Of The Following Is Not A Pure Substance
Apr 22, 2025
-
Metals Are Good Conductors Of Heat And Electricity
Apr 22, 2025
-
Mending Wall Summary Line By Line
Apr 22, 2025
-
Can Mixtures Be Separated By Chemical Means
Apr 22, 2025
-
Find The Cosine Of The Angle Between The Vectors
Apr 22, 2025
Related Post
Thank you for visiting our website which covers about How To Index A Tuple In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.