How To Do Summation In Python
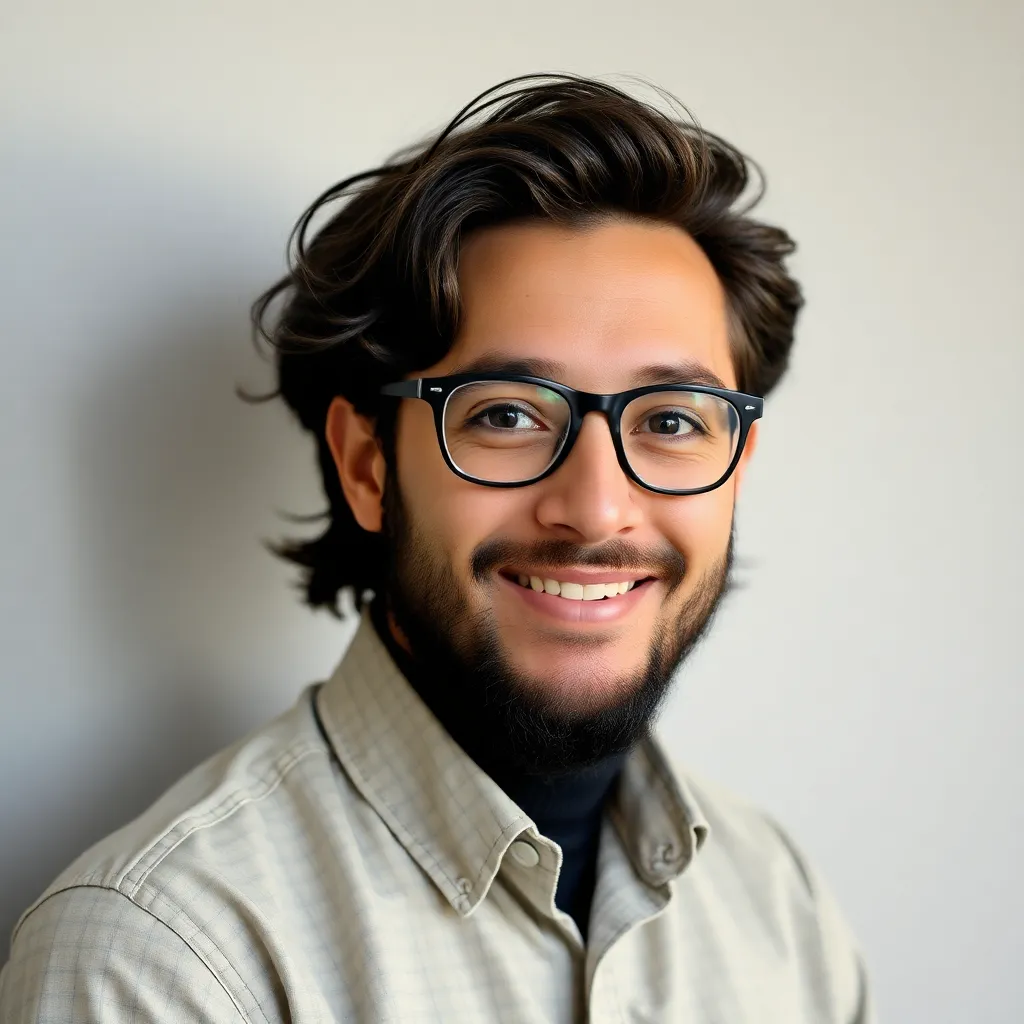
News Leon
Apr 16, 2025 · 6 min read

Table of Contents
Mastering Summation in Python: A Comprehensive Guide
Python, renowned for its readability and versatility, offers numerous approaches to perform summation. Whether you're dealing with simple arithmetic series or complex mathematical functions, Python provides efficient and elegant solutions. This comprehensive guide explores various methods for summation in Python, catering to beginners and experienced programmers alike. We'll delve into fundamental techniques, advanced strategies, and best practices to ensure you master the art of summation in Python.
Fundamental Methods: The Building Blocks of Summation
Let's start with the most straightforward approaches: using loops and the built-in sum()
function. These form the bedrock of summation in Python, providing a solid foundation for more complex calculations.
1. The for
Loop Approach: Iterative Summation
The for
loop is a fundamental construct in Python, perfectly suited for iterative summation. It allows you to iterate through a sequence (list, tuple, or range) and accumulate the values.
numbers = [1, 2, 3, 4, 5]
total = 0
for number in numbers:
total += number
print(f"The sum is: {total}")
This simple code iterates through the numbers
list, adding each element to the total
variable. This approach is intuitive and easy to understand, making it ideal for beginners. However, for large datasets, it can be less efficient than other methods.
2. Leveraging the sum()
Function: Concise Summation
Python's built-in sum()
function provides a more concise and often more efficient way to calculate the sum of elements in an iterable.
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(f"The sum is: {total}")
This single line of code achieves the same result as the for
loop example, significantly reducing code complexity. The sum()
function is optimized for speed and is generally the preferred method for summing numerical iterables.
3. Handling Different Data Structures: Adaptability is Key
The for
loop and sum()
function are versatile and can handle various data structures. Let's explore how to adapt them for different scenarios:
a. Summing elements in a tuple:
numbers_tuple = (1, 2, 3, 4, 5)
total = sum(numbers_tuple) # sum() works directly with tuples
print(f"The sum is: {total}")
b. Summing elements in a NumPy array:
NumPy arrays, used extensively in scientific computing, offer optimized summation capabilities.
import numpy as np
numbers_array = np.array([1, 2, 3, 4, 5])
total = np.sum(numbers_array) # NumPy's sum() function
print(f"The sum is: {total}")
NumPy's sum()
function often outperforms Python's built-in sum()
for large arrays due to its vectorized operations.
c. Summing values from a dictionary:
Summing dictionary values requires iterating through the dictionary's items.
data = {'a': 1, 'b': 2, 'c': 3}
total = sum(data.values())
print(f"The sum is: {total}")
Advanced Summation Techniques: Expanding Your Toolkit
Beyond the fundamentals, Python offers powerful tools for handling more complex summation scenarios. These techniques are crucial for tackling intricate mathematical problems and large datasets.
1. Summing with List Comprehension: Concise and Efficient
List comprehensions provide a compact way to generate lists and perform operations simultaneously. They can be incredibly efficient for summing elements based on specific conditions.
numbers = [1, 2, 3, 4, 5, 6]
even_sum = sum([number for number in numbers if number % 2 == 0])
print(f"The sum of even numbers is: {even_sum}")
This code elegantly sums only the even numbers in the numbers
list. The conciseness and efficiency of list comprehensions make them a valuable tool for advanced summation.
2. Numerical Integration: Approximating Definite Integrals
For continuous functions, numerical integration techniques approximate the definite integral, essentially summing infinitely small slices under the curve. Libraries like SciPy provide powerful tools for this:
from scipy import integrate
import numpy as np
def f(x):
return x**2
result, error = integrate.quad(f, 0, 1) # Integrate x^2 from 0 to 1
print(f"The definite integral is approximately: {result}")
integrate.quad()
is a powerful function for numerical integration, handling a wide range of functions.
3. Handling Infinite Series: Convergence and Precision
Many mathematical functions are represented by infinite series. Python can be used to approximate the sum of these series up to a certain level of precision. However, it's crucial to consider convergence: does the series approach a finite limit?
def approximate_e(n):
e = 0
factorial = 1
for i in range(n):
e += 1 / factorial
factorial *= (i + 1)
return e
approximation = approximate_e(10) # Approximating e using the first 10 terms
print(f"Approximation of e: {approximation}")
This example approximates the mathematical constant e using its Taylor series expansion. The accuracy improves with more terms (n
), but convergence must be considered.
4. Parallel Summation with Multiprocessing: Boosting Performance
For extremely large datasets, parallel processing can significantly accelerate summation. Python's multiprocessing
library allows you to distribute the summation task across multiple CPU cores.
import multiprocessing
def sum_chunk(chunk):
return sum(chunk)
numbers = list(range(1000000)) # a large list of numbers
chunk_size = 100000
chunks = [numbers[i:i + chunk_size] for i in range(0, len(numbers), chunk_size)]
with multiprocessing.Pool() as pool:
partial_sums = pool.map(sum_chunk, chunks)
total_sum = sum(partial_sums)
print(f"Total sum: {total_sum}")
This example divides the summation task into chunks and processes them concurrently, substantially reducing computation time for large lists. However, the overhead of managing processes can outweigh the benefits for smaller datasets.
Error Handling and Best Practices: Ensuring Robust Code
Robust code is crucial for reliable summation. Let's examine strategies for handling potential errors and writing efficient, maintainable code.
1. Input Validation: Protecting Against Unexpected Data
Always validate your input data to prevent errors. Check if the input is a numerical iterable, handle potential exceptions (like TypeError
if you try to sum non-numerical values), and ensure data integrity.
def safe_sum(data):
try:
if not isinstance(data, (list, tuple, np.ndarray)):
raise TypeError("Input must be a list, tuple, or NumPy array.")
if not all(isinstance(x, (int, float)) for x in data):
raise ValueError("Input must contain only numbers.")
return sum(data)
except TypeError as e:
print(f"Error: {e}")
return None
except ValueError as e:
print(f"Error: {e}")
return None
print(safe_sum([1,2,'a'])) # demonstrates error handling
2. Memory Management: Handling Large Datasets
For extremely large datasets that might exceed available memory, consider using generators or iterative approaches to process the data in chunks, preventing memory errors.
def sum_large_file(filepath):
total = 0
with open(filepath, 'r') as file:
for line in file:
try:
number = float(line.strip()) # Assumes each line contains a single number. Adapt as needed.
total += number
except ValueError:
print(f"Skipping invalid line: {line.strip()}")
return total
#Example usage:
#total = sum_large_file("large_numbers.txt")
#print(f"Sum from file: {total}")
This avoids loading the entire file into memory at once.
3. Documentation and Readability: Writing Maintainable Code
Always document your code clearly, including explanations of algorithms, input/output expectations, and any assumptions made. Use meaningful variable names and follow consistent coding style guidelines to ensure readability and maintainability.
Conclusion: Mastering the Art of Summation in Python
Python offers a rich toolkit for performing summation, from simple loops to advanced numerical integration and parallel processing techniques. By understanding these methods and employing best practices for error handling and memory management, you can effectively tackle diverse summation problems, regardless of their complexity or scale. Remember that choosing the optimal method depends on the specific context – the size of your data, the type of data, performance requirements, and the overall complexity of your task. Mastering these techniques empowers you to write efficient, reliable, and elegant Python code for any summation challenge.
Latest Posts
Latest Posts
-
Benzaldehyde And Acetone Aldol Condensation Mechanism
Apr 19, 2025
-
Give One Example Of A Chemical Change
Apr 19, 2025
-
A Compound A Has The Formula C8h10
Apr 19, 2025
-
Is Milk Of Magnesia An Acid Or A Base
Apr 19, 2025
-
Used In Remote Controls For Televisions
Apr 19, 2025
Related Post
Thank you for visiting our website which covers about How To Do Summation In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.