How To Create A List Of Numbers In Python
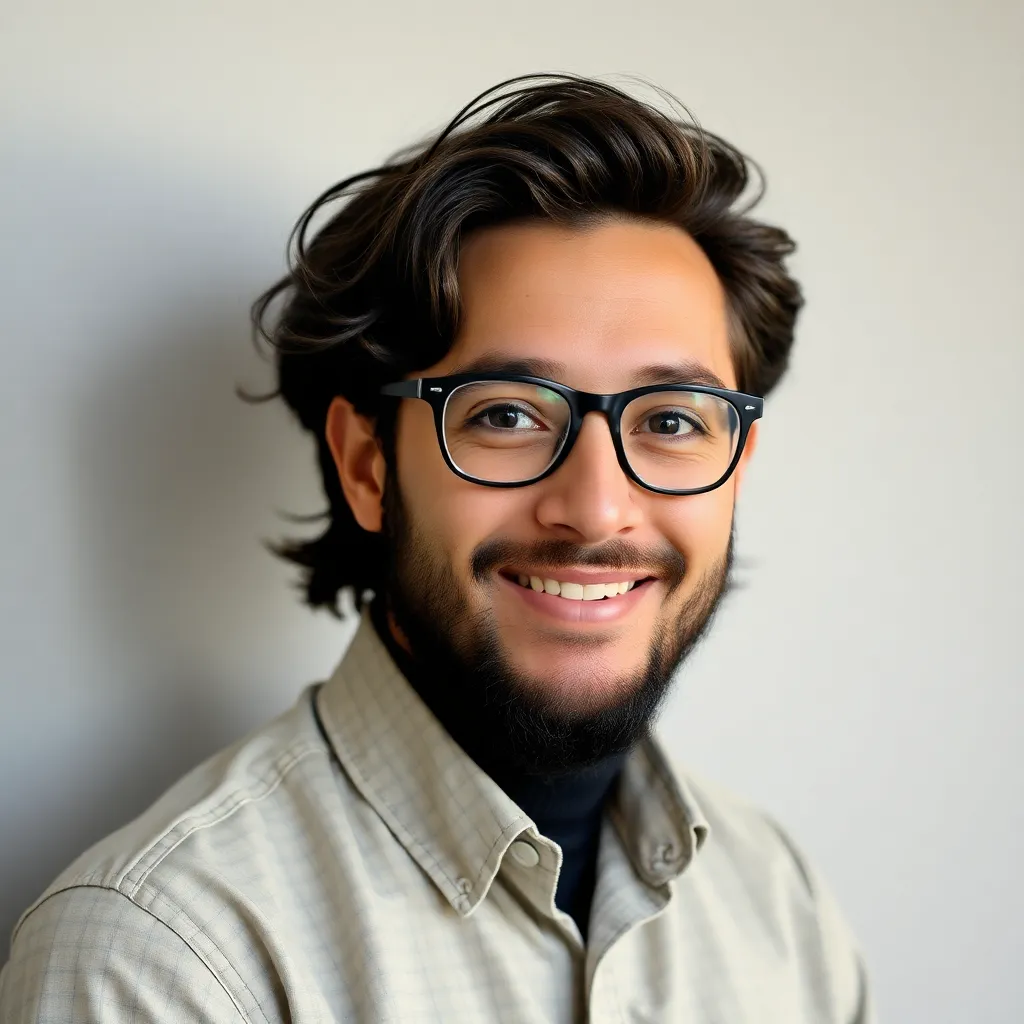
News Leon
Apr 07, 2025 · 5 min read
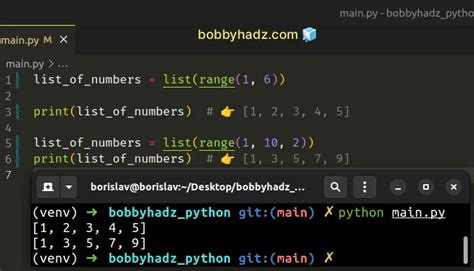
Table of Contents
How to Create a List of Numbers in Python: A Comprehensive Guide
Python, renowned for its readability and versatility, offers several elegant ways to generate lists of numbers. Whether you need a simple sequence, a range with specific steps, or a more complex pattern, Python provides the tools to accomplish this efficiently. This comprehensive guide will explore various techniques, from basic methods to advanced functionalities, equipping you with the knowledge to create any numerical list you need.
Understanding Python Lists
Before diving into the creation of numerical lists, let's establish a foundational understanding of Python lists. A list is an ordered, mutable (changeable) collection of items. These items can be of different data types – integers, floats, strings, even other lists – but for this guide, our focus will remain on numerical lists.
Lists are defined using square brackets []
with items separated by commas. For example:
my_list = [1, 2, 3, 4, 5]
Basic Methods for Creating Numerical Lists
Let's explore the fundamental approaches to generate lists of numbers in Python:
1. Manual List Creation
The most straightforward method is to explicitly define the list elements:
numbers = [1, 5, 10, 15, 20] #A simple list of numbers
This approach is suitable for short, predefined sequences but becomes cumbersome for longer lists.
2. Using range()
Function
The range()
function is a powerful tool for generating sequences of numbers. It produces a sequence of numbers starting from a specified start value (inclusive) up to an end value (exclusive) with an optional step size.
# Generate numbers from 0 to 9 (exclusive of 10)
my_range = list(range(10)) # Output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Generate numbers from 2 to 20 with a step of 2
even_numbers = list(range(2, 21, 2)) # Output: [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
# Generate numbers from 10 down to 0
reverse_range = list(range(10, -1, -1)) # Output: [10, 9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
Note: The list()
function is crucial here because range()
itself returns a range object, not a list. To work with the sequence as a list, you must convert it using list()
.
3. List Comprehension: A Concise Approach
List comprehension offers a highly concise and Pythonic way to generate lists. It allows you to create a list based on an existing iterable (like a range
object) and apply an expression to each element.
# Create a list of squares from 0 to 9
squares = [x**2 for x in range(10)] # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
# Create a list of even numbers from 0 to 20
even_numbers_comprehension = [x for x in range(21) if x % 2 == 0] #Output: [0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
# Create a list of numbers and their squares as tuples
number_squares = [(x, x**2) for x in range(1,6)] #Output: [(1, 1), (2, 4), (3, 9), (4, 16), (5, 25)]
List comprehensions are significantly more readable and efficient than traditional loops for list generation.
Advanced Techniques for Generating Numerical Lists
Beyond the basics, Python provides tools for generating more complex numerical lists:
1. Using numpy
for Numerical Computation
The numpy
library is indispensable for numerical computation in Python. Its arange()
and linspace()
functions offer superior performance for generating large numerical arrays (which are similar to lists but optimized for numerical operations):
import numpy as np
# Generate an array of numbers from 0 to 10 (exclusive of 11) with a step of 0.5
numpy_array = np.arange(0, 11, 0.5) # Output: array([ 0. , 0.5, 1. , 1.5, 2. , 2.5, 3. , 3.5, 4. , 4.5, 5. , 5.5, 6. , 6.5, 7. , 7.5, 8. , 8.5, 9. , 9.5, 10. ])
# Generate an array of 10 evenly spaced numbers between 0 and 1
linspace_array = np.linspace(0, 1, 10) # Output: array([0. , 0.11111111, 0.22222222, 0.33333333, 0.44444444,
# 0.55555556, 0.66666667, 0.77777778, 0.88888889, 1. ])
numpy
excels when dealing with large datasets and vectorized operations, significantly improving performance compared to standard Python lists.
2. Generating Random Number Lists
The random
module is essential for creating lists of random numbers. You can generate lists of random integers, floats, or even sample from existing distributions.
import random
# Generate a list of 10 random integers between 1 and 100 (inclusive)
random_integers = [random.randint(1, 100) for _ in range(10)]
# Generate a list of 5 random floating-point numbers between 0 and 1
random_floats = [random.random() for _ in range(5)]
# Generate a list of 5 random numbers from a normal distribution (mean=0, standard deviation=1)
import numpy as np
random_normal = np.random.normal(0,1,5).tolist() # .tolist() converts numpy array to a list
Remember to import the random
module before using its functions.
3. Fibonacci Sequence Generation
The Fibonacci sequence is a classic example of a numerical sequence where each number is the sum of the two preceding ones. Let's see how to generate it in Python:
def fibonacci(n):
"""Generates a Fibonacci sequence of length n."""
fib_sequence = []
a, b = 0, 1
for _ in range(n):
fib_sequence.append(a)
a, b = b, a + b
return fib_sequence
print(fibonacci(10)) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
This function uses an iterative approach to efficiently generate the sequence.
4. Generating Lists with Custom Patterns
You can create lists based on any mathematical formula or pattern using loops and conditional statements. For instance, let's create a list where each element is the square of its index:
indexed_squares = [i**2 for i in range(1,11)]
print(indexed_squares) #Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
This example demonstrates the flexibility of list comprehension to adapt to various patterns.
Choosing the Right Method
The optimal method for creating a numerical list depends on your specific needs:
- Simple, short lists: Manual creation is sufficient.
- Sequences with regular intervals:
range()
is the most efficient. - Concise generation with transformations: List comprehension shines.
- Large datasets, numerical computations:
numpy
offers superior performance. - Random numbers: Utilize the
random
module. - Custom patterns: Combine loops, conditionals, and potentially list comprehension.
By mastering these techniques, you'll be well-equipped to handle various numerical list generation tasks in your Python projects. Remember to choose the most efficient and readable method based on your specific context. The examples and explanations provided offer a strong foundation for creating any numerical list you require. Experimentation and further exploration of Python's rich functionalities will solidify your understanding and expand your capabilities.
Latest Posts
Latest Posts
-
Develop And Change Over A Period Of Time
Apr 10, 2025
-
All Irrational Numbers Are Real Numbers True False
Apr 10, 2025
-
What Is The Gram Formula Mass Of K2co3
Apr 10, 2025
-
Which Of The Following Is Abiotic
Apr 10, 2025
-
Do You Often Go To Restaurants
Apr 10, 2025
Related Post
Thank you for visiting our website which covers about How To Create A List Of Numbers In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.