How To Center Text In Python
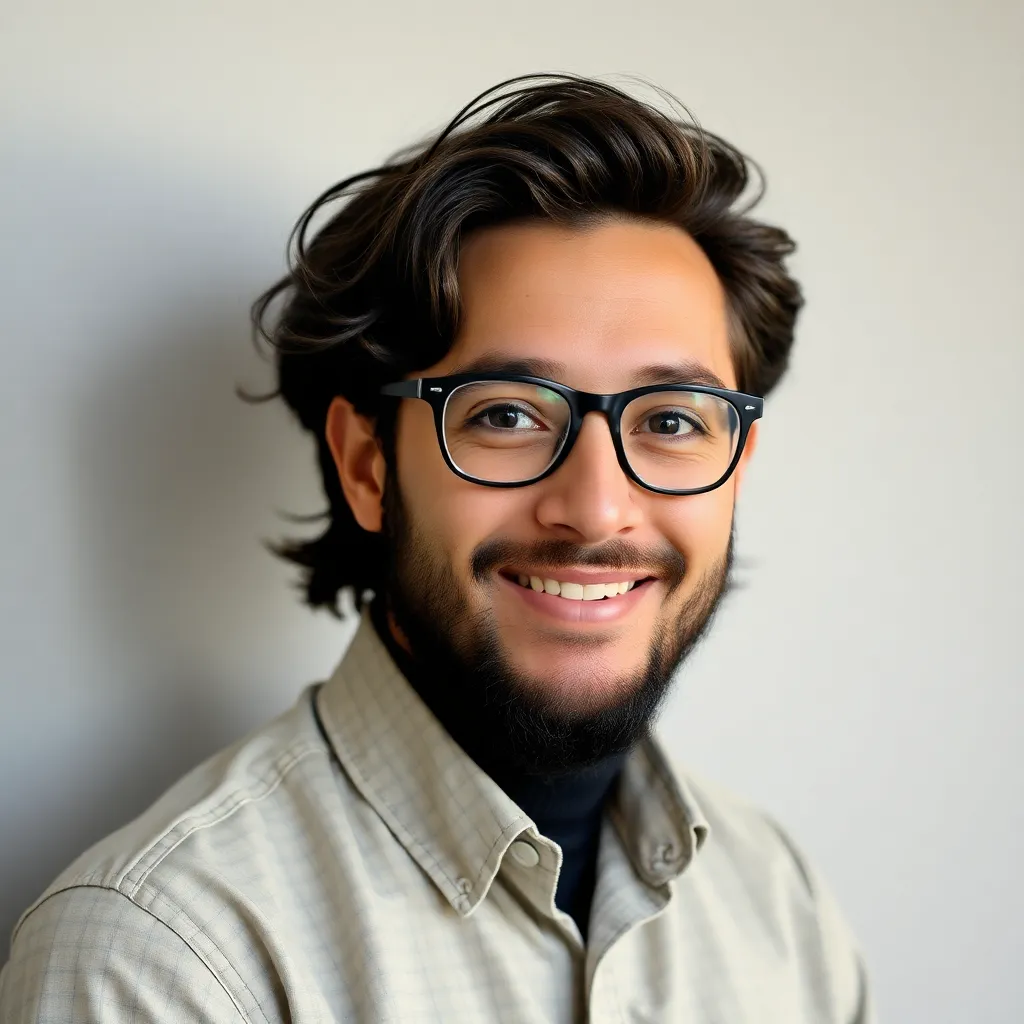
News Leon
Mar 22, 2025 · 6 min read
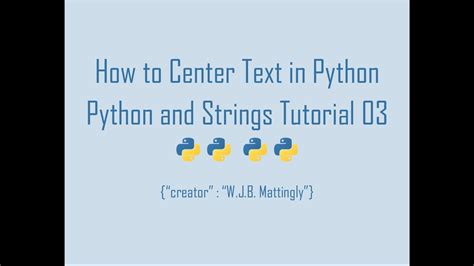
Table of Contents
How to Center Text in Python: A Comprehensive Guide
Centering text is a fundamental task in many Python programming projects, from simple scripts to complex applications. Whether you're creating user interfaces, generating reports, or formatting data for output, knowing how to center text effectively is crucial. This comprehensive guide dives deep into various methods for centering text in Python, catering to different scenarios and skill levels. We'll explore techniques for centering text within a fixed width, centering text within a terminal or console, and handling more advanced scenarios like centering text within a graphical user interface (GUI).
Understanding the Need for Text Centering
Before jumping into the code, let's establish why centering text is so important. In many applications, visually appealing and well-organized output is key to a positive user experience. Centered text contributes significantly to:
- Improved Readability: Centered text can make text blocks easier to read, especially when dealing with titles, headings, or important messages.
- Enhanced Aesthetics: Proper text alignment contributes significantly to the overall visual appeal of any output, be it a simple print statement or a complex GUI.
- Clear Communication: In data presentation, centered text can help highlight key data points and improve the overall clarity of the information being conveyed.
- Professionalism: Well-formatted output demonstrates attention to detail and professionalism, reflecting positively on your work.
Methods for Centering Text in Python
Python offers several approaches to centering text, depending on your specific needs and context. We'll examine the most common and effective methods.
1. Centering Text within a Fixed Width Using String Manipulation
This is the most straightforward approach for centering text within a predetermined width. It involves calculating the padding needed on either side of the text and then using string formatting to achieve the desired effect.
def center_text(text, width):
"""Centers text within a specified width.
Args:
text: The text to be centered.
width: The desired width of the output.
Returns:
The centered text string. Returns the original text if width is too small.
"""
if len(text) >= width:
return text # Return original text if it's wider than the width
padding = width - len(text)
left_padding = padding // 2
right_padding = padding - left_padding
return " " * left_padding + text + " " * right_padding
# Example Usage
text = "Hello, world!"
width = 25
centered_text = center_text(text, width)
print(centered_text)
text2 = "This is a longer string that might exceed the specified width"
width2 = 40
centered_text2 = center_text(text2, width2)
print(centered_text2)
This function calculates the necessary padding and adds spaces accordingly. The //
operator performs integer division, ensuring that the padding is evenly distributed. The function also includes error handling for cases where the text is longer than the specified width.
2. Centering Text in a Terminal or Console
For console applications, you might need to consider the terminal's width dynamically. The shutil
module can help determine the terminal size. However, this approach relies on the terminal correctly reporting its size, which isn't always guaranteed.
import shutil
import os
def center_text_console(text):
"""Centers text within the console window. Handles potential errors gracefully."""
try:
terminal_width, _ = shutil.get_terminal_size()
return center_text(text, terminal_width)
except OSError:
# Handle cases where terminal size cannot be retrieved (e.g., in IDEs)
print("Warning: Could not determine terminal size. Text may not be centered correctly.")
return text
#Example usage
my_text = "This text will be centered in your console!"
centered_console_text = center_text_console(my_text)
print(centered_console_text)
#Testing error handling
os.environ['TERM'] = 'dumb' #Simulate a terminal that doesn't report size
test_text = "This should not center perfectly due to simulated error"
error_handled_text = center_text_console(test_text)
print(error_handled_text)
This version adapts to the console width, making it more versatile. The try-except
block adds robustness by handling potential errors gracefully if the terminal size cannot be determined.
3. Centering Text in a Graphical User Interface (GUI)
Centering text within a GUI framework like Tkinter, PyQt, or Kivy requires utilizing the framework's specific layout managers and widgets. The exact implementation varies depending on the chosen framework. Below is an example using Tkinter:
import tkinter as tk
def center_text_tkinter(text, master):
"""Centers text within a Tkinter label.
Args:
text: The text to center.
master: The parent widget (e.g., a window).
"""
label = tk.Label(master, text=text)
label.pack(expand=True) #Causes Label to expand to fill parent widget
#To center without expanding the label:
#label.pack(fill=tk.BOTH, expand=False)
#label.place(relx=0.5, rely=0.5, anchor=tk.CENTER)
#Example usage in Tkinter
root = tk.Tk()
root.title("Centered Text in Tkinter")
center_text_tkinter("This text is centered in a Tkinter window!", root)
root.mainloop()
This example demonstrates centering text within a Tkinter label using the pack
geometry manager. Other layout managers (like grid
or place
) can also be used, offering more precise control over text positioning. Remember to adapt this to your chosen GUI framework and its specific layout mechanisms. The use of pack()
with expand=True
here centers the label, but it's also important to note the alternative using place()
that would center the text within the label itself without expanding the label to fill the parent widget.
4. Centering Multiline Text
Centering multiline text requires a slightly more sophisticated approach. You'll need to center each line individually and then join them together.
def center_multiline_text(text, width):
"""Centers each line of multiline text within a specified width."""
lines = text.splitlines()
centered_lines = [center_text(line, width) for line in lines]
return "\n".join(centered_lines)
# Example Usage
multiline_text = """This is a multiline
string that needs to be
centered."""
width = 30
centered_multiline = center_multiline_text(multiline_text, width)
print(centered_multiline)
This function iterates through each line, centers it using the center_text
function from earlier, and then rejoins the lines to create the final centered multiline text.
5. Advanced Techniques: Using Rich Text Libraries
For more complex formatting needs, consider using rich text libraries like rich
which offer advanced features beyond simple string manipulation. Libraries like these provide features for styling, color, and more sophisticated text layout options.
Choosing the Right Method
The optimal method for centering text in Python depends heavily on the context of your project:
- Simple text output: String manipulation is sufficient.
- Console applications: Utilize
shutil.get_terminal_size()
for dynamic width adjustment. - GUI applications: Leverage the layout managers and widgets provided by your GUI framework (Tkinter, PyQt, etc.).
- Multiline text: Adapt the multiline text centering function.
- Complex formatting: Explore rich text libraries for advanced control.
Best Practices and Considerations
- Error Handling: Always include error handling (like
try-except
blocks) to gracefully manage potential issues, such as failure to retrieve terminal size. - Readability: Prioritize code clarity and maintainability. Well-structured code is easier to understand and maintain.
- Efficiency: Consider efficiency, especially when dealing with large amounts of text. Optimize your algorithms to avoid unnecessary computations.
- Flexibility: Design your functions to be versatile and adaptable to various input sizes and scenarios.
- Testing: Thoroughly test your code with different inputs and edge cases to ensure correctness.
This comprehensive guide provides you with a robust foundation for centering text in Python, covering various scenarios and techniques. Remember to choose the method that best suits your specific needs and project requirements, and always strive for clean, efficient, and well-tested code. By mastering these techniques, you can significantly enhance the visual appeal and usability of your Python applications.
Latest Posts
Latest Posts
-
What Nitrogenous Base Is Part Of Dna But Not Rna
Mar 24, 2025
-
In Mechanism Photophosphorylation Is Most Similar To
Mar 24, 2025
-
The Figure Shows The Circuit Of A Flashing Lamp
Mar 24, 2025
-
How Many Germ Layers Do Sponges Have
Mar 24, 2025
-
3 Is What Percentage Of 18
Mar 24, 2025
Related Post
Thank you for visiting our website which covers about How To Center Text In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.