How To Access Dict Values In Python
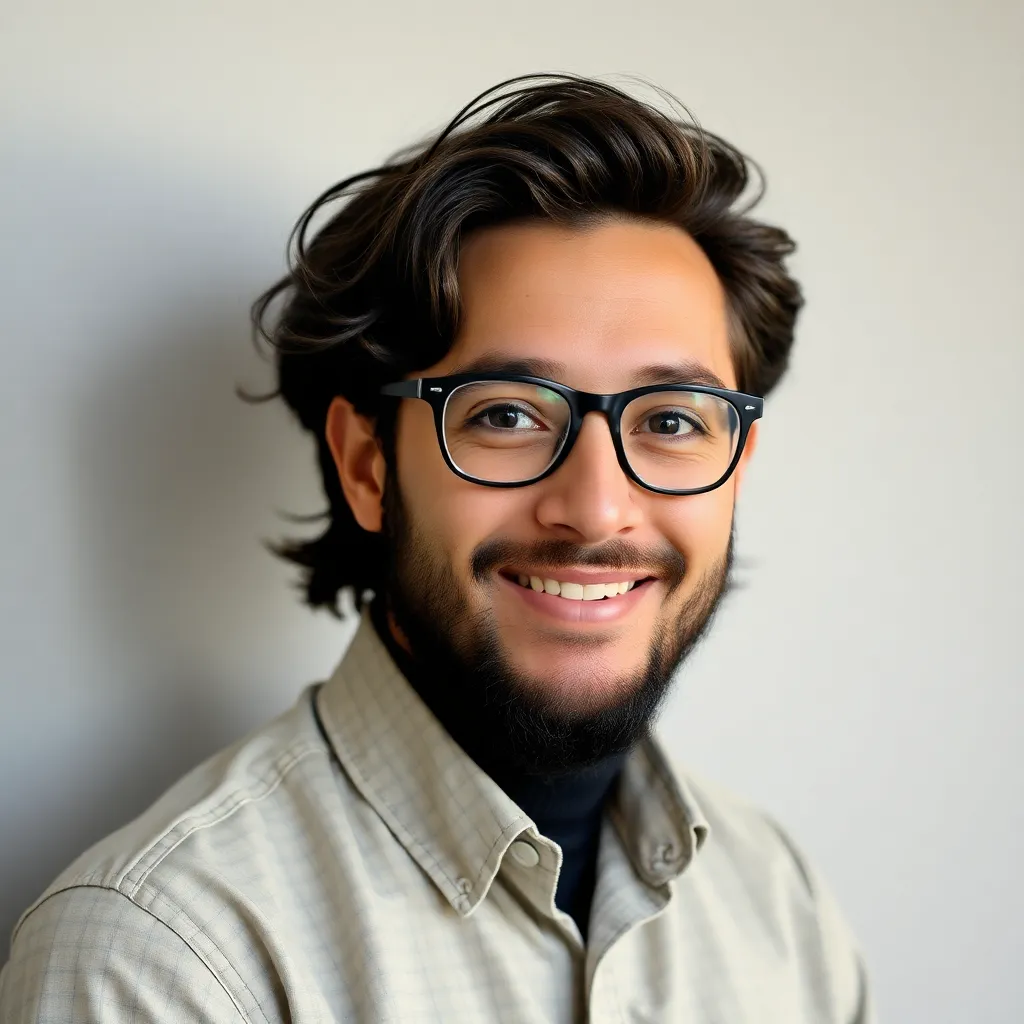
News Leon
Apr 12, 2025 · 5 min read

Table of Contents
How to Access Dict Values in Python: A Comprehensive Guide
Python dictionaries are fundamental data structures offering efficient key-value storage. Mastering dictionary value access is crucial for any Python programmer. This comprehensive guide delves into various methods, showcasing practical examples and best practices to ensure you confidently navigate the world of Python dictionaries.
Understanding Python Dictionaries
Before diving into accessing values, let's solidify our understanding of Python dictionaries. Dictionaries are unordered collections of key-value pairs. Each key must be unique and immutable (like strings, numbers, or tuples), while values can be of any data type, including other dictionaries (nested dictionaries). This flexibility makes dictionaries highly versatile for representing structured data.
Example:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York",
"address": {
"street": "123 Main St",
"zip": "10001"
}
}
This dictionary stores information about Alice, including nested data within the address
key.
Primary Methods for Accessing Dictionary Values
Python provides several ways to retrieve values from dictionaries, each with its own advantages and use cases.
1. Direct Access Using Square Brackets []
The most straightforward approach uses square brackets with the key inside. This method returns the value associated with the specified key.
Example:
name = my_dict["name"] # Accesses the value associated with the key "name"
print(name) # Output: Alice
city = my_dict["city"]
print(city) # Output: New York
street = my_dict["address"]["street"] # Accessing value from nested dictionary
print(street) # Output: 123 Main St
Important Note: Attempting to access a non-existent key using this method raises a KeyError
. Therefore, it's crucial to handle potential KeyError
exceptions using try-except
blocks for robust code.
Example with Error Handling:
try:
occupation = my_dict["occupation"]
print(occupation)
except KeyError:
print("The key 'occupation' does not exist in the dictionary.")
2. Using the get()
Method
The get()
method provides a safer alternative to direct bracket access. It takes the key as the first argument and an optional second argument representing a default value. If the key exists, it returns the corresponding value; otherwise, it returns the default value (which defaults to None
if not specified).
Example:
occupation = my_dict.get("occupation") # Returns None if "occupation" doesn't exist
print(occupation) # Output: None
occupation = my_dict.get("occupation", "Unknown") # Returns "Unknown" if key doesn't exist
print(occupation) # Output: Unknown
age = my_dict.get("age")
print(age) # Output: 30
The get()
method prevents KeyError
exceptions, making your code cleaner and less prone to errors.
3. Iterating Through Dictionary Values
Python's for
loop provides elegant ways to iterate over dictionary values. The values()
method returns a view object containing all the values in the dictionary.
Example:
for value in my_dict.values():
print(value)
This loop will print each value in the my_dict
one by one. Note that the order might not be consistent across different Python versions because dictionaries are unordered.
4. Iterating Through Key-Value Pairs Using items()
The items()
method returns a view object containing key-value pairs as tuples. This is particularly useful when you need both the key and its corresponding value.
Example:
for key, value in my_dict.items():
print(f"Key: {key}, Value: {value}")
This loop neatly prints each key-value pair. This approach is highly beneficial when processing data where both key and value context are important.
Advanced Techniques for Accessing Dict Values
Beyond the basic methods, several advanced techniques facilitate efficient value access in complex scenarios:
1. Accessing Values Based on Conditions
You can combine conditional statements with value access to retrieve values based on specific criteria.
Example:
if my_dict.get("age", 0) > 25:
print("Alice is over 25 years old.")
This example checks if Alice's age is greater than 25 before printing a message.
2. Nested Dictionaries and Deep Access
Accessing values within nested dictionaries requires chaining access methods.
Example:
zip_code = my_dict["address"].get("zip", "N/A")
print(zip_code) # Output: 10001
# safer approach using get() method multiple times
street_name = my_dict.get("address", {}).get("street", "Address not found")
print(street_name)
The get()
method with default values is crucial here to gracefully handle cases where nested keys might be missing.
3. Using List Comprehension for Concise Value Extraction
List comprehensions offer a compact way to extract values based on conditions.
Example:
ages = [person["age"] for person in my_list_of_dictionaries if person.get("city") == "New York"]
Assuming my_list_of_dictionaries
contains a list of dictionaries, each representing a person, this concisely extracts the ages of all people living in New York.
4. Working with Default Dictionaries
The collections.defaultdict
class offers a powerful way to handle missing keys without explicit error handling. It takes a factory function (e.g., int
, list
, dict
) as an argument. When you access a non-existent key, it automatically creates an entry with a default value from the factory.
Example:
from collections import defaultdict
word_counts = defaultdict(int) # default value is 0 for integers
sentence = "This is a test sentence."
for word in sentence.split():
word_counts[word] += 1
print(word_counts) # Counts each word in the sentence
This automatically handles words not yet seen in the word_counts
dictionary.
5. Filtering Dictionary Values
You can filter dictionary values using dictionary comprehensions to create a new dictionary containing only the values that meet certain criteria.
Example:
my_dict = {"a": 1, "b": 2, "c": 3, "d": 4}
filtered_dict = {k: v for k, v in my_dict.items() if v > 2}
print(filtered_dict) # Output: {'c': 3, 'd': 4}
Best Practices and Error Handling
- Always Use
get()
for Non-Critical Keys: Prioritizeget()
to avoidKeyError
exceptions, especially when dealing with user input or external data sources. - Robust Error Handling: Employ
try-except
blocks to gracefully handle potentialKeyError
exceptions. - Clear Variable Names: Use descriptive variable names to enhance code readability.
- Avoid Deeply Nested Dictionaries: Excessive nesting can make code hard to read and maintain. Consider alternative data structures if necessary (e.g., custom classes).
- Test Thoroughly: Write unit tests to verify that your dictionary value access functions correctly in various scenarios, including edge cases.
Conclusion
Accessing dictionary values in Python is a fundamental skill. By mastering these methods and best practices, you can write efficient, robust, and readable Python code. Remember to choose the appropriate method based on your specific needs, prioritizing error handling and code clarity to create high-quality, maintainable applications. The flexibility of dictionaries and the power of these methods ensure you can manage and manipulate data effectively in your Python projects.
Latest Posts
Related Post
Thank you for visiting our website which covers about How To Access Dict Values In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.