Distance Between 2 Lines In 3d
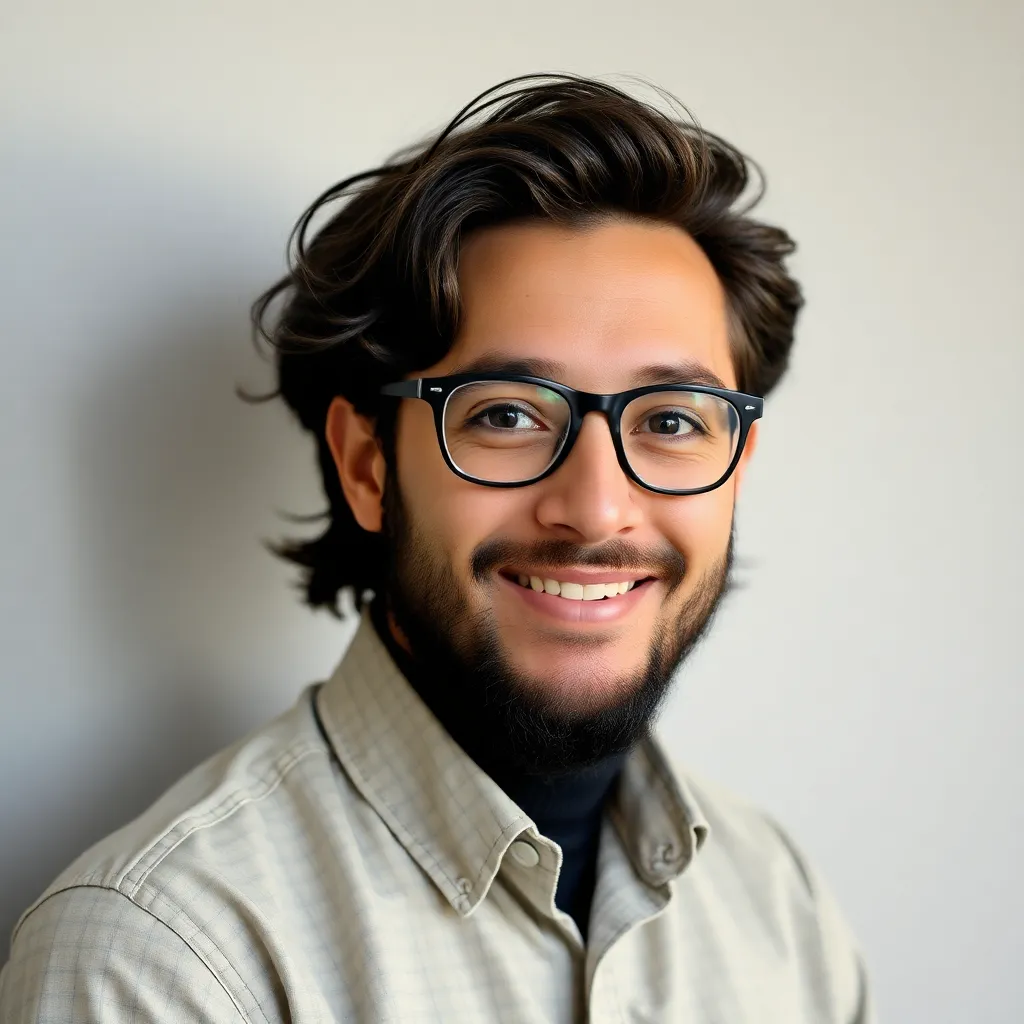
News Leon
Mar 16, 2025 · 6 min read
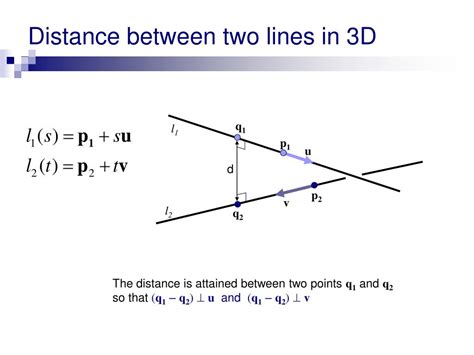
Table of Contents
Calculating the Distance Between Two Lines in 3D Space
Determining the distance between two lines in three-dimensional space is a fundamental problem in various fields, including computer graphics, physics simulations, and robotics. Unlike the relatively straightforward calculation of distance between two points or a point and a line, the distance between two lines requires a more nuanced approach. This article will delve into the methods for calculating this distance, explaining the underlying mathematics and providing practical examples.
Understanding the Problem
Before diving into the solutions, it's crucial to understand the different scenarios we might encounter. Two lines in 3D space can be:
- Intersecting: The lines share a common point. In this case, the distance between them is zero.
- Parallel: The lines never intersect, and the distance between them is constant throughout their lengths.
- Skew: The lines are neither intersecting nor parallel; they exist in different planes and maintain a minimum distance. This is the most complex scenario.
Method 1: Using Vector Projections for Parallel and Skew Lines
This method leverages vector projections to find the shortest distance between two lines. It's particularly useful for parallel and skew lines.
Defining the Lines
Let's define two lines, L1 and L2, in 3D space using vector notation:
- Line L1:
P1 + t * v1
where P1 is a point on L1, v1 is the direction vector of L1, and t is a scalar parameter. - Line L2:
P2 + s * v2
where P2 is a point on L2, v2 is the direction vector of L2, and s is a scalar parameter.
Calculating the Shortest Distance
-
Find the vector connecting points on the two lines: Let's denote this vector as
w = P2 - P1
. -
Find the vector that is perpendicular to both direction vectors: This vector represents the direction of the shortest distance between the lines. We find this using the cross product:
n = v1 x v2
. If the lines are parallel,n
will be the zero vector, indicating a distance calculation error (handled in the next section). -
Project the connecting vector onto the perpendicular vector: The magnitude of this projection is the shortest distance between the two lines. The projection is given by:
d = |(w • n) / ||n|| |
, where•
represents the dot product and||n||
represents the magnitude of vectorn
.
Example:
Let's say:
- P1 = (1, 2, 3)
- v1 = (2, 1, 0)
- P2 = (4, 1, 2)
- v2 = (1, -1, 1)
w = P2 - P1 = (3, -1, -1)
n = v1 x v2 = (1, -2, -3)
w • n = 3(1) + (-1)(-2) + (-1)(-3) = 8
||n|| = √(1² + (-2)² + (-3)²) = √14
d = |8 / √14| ≈ 2.14
Therefore, the shortest distance between these skew lines is approximately 2.14 units.
Handling Parallel Lines
If the lines are parallel (v1
is a scalar multiple of v2
), the cross product n
will be the zero vector. In this case, we project the vector w
onto a vector perpendicular to both lines. We can use the formula d = |w x v1| / ||v1||
(or equivalently, using v2
).
Method 2: Using a System of Linear Equations (for Intersecting Lines)
This method is more suitable for determining whether lines intersect and finding the intersection point (distance is zero).
Setting Up the Equations
Recall the parametric equations of the lines:
- L1: x = x1 + ta1, y = y1 + tb1, z = z1 + t*c1
- L2: x = x2 + sa2, y = y2 + sb2, z = z2 + s*c2
If the lines intersect, there must exist values of 't' and 's' that satisfy all three equations simultaneously. This leads to a system of three linear equations with two unknowns (t and s).
Solving the System
We can use methods such as substitution or matrix operations (Gaussian elimination) to solve this system. If a solution exists (consistent system), the lines intersect. If no solution exists (inconsistent system), the lines are skew or parallel. If an infinite number of solutions exist (dependent system), the lines are coincident (identical).
Method 3: Geometric Approach for Parallel Lines
For parallel lines, a simpler geometric approach exists:
- Find a vector connecting a point on one line to the other line: Select a point P1 on L1.
- Project this vector onto a vector perpendicular to both lines: The magnitude of the projection is the distance between the parallel lines.
This method bypasses the need for the cross product, making it computationally less expensive for parallel lines.
Code Implementation (Conceptual Python)
While providing complete code implementations for various programming languages is beyond the scope of this article (to keep the focus on mathematical concepts), the following Python snippets offer conceptual representations of the algorithms. Note that robust error handling and edge-case management would be needed in production-ready code.
import numpy as np
def distance_between_lines(P1, v1, P2, v2):
"""Calculates the distance between two lines in 3D space."""
w = np.array(P2) - np.array(P1)
v1 = np.array(v1)
v2 = np.array(v2)
n = np.cross(v1, v2)
if np.allclose(n, 0): # Check for parallel lines
# Handle parallel lines (using projection)
pass # Implementation for parallel lines goes here
else:
d = np.abs(np.dot(w, n) / np.linalg.norm(n))
return d
#Example usage (replace with your line data):
P1 = [1, 2, 3]
v1 = [2, 1, 0]
P2 = [4, 1, 2]
v2 = [1, -1, 1]
distance = distance_between_lines(P1, v1, P2, v2)
print(f"Distance between lines: {distance}")
Remember to adapt and enhance this code with proper error handling and vector library usage.
Applications
The ability to calculate the distance between two lines in 3D space has far-reaching applications:
-
Collision Detection: In computer games and simulations, this calculation helps determine if objects (represented as lines or line segments) are colliding.
-
Robotics: Planning robot trajectories often involves finding the shortest distance between a robot's arm and obstacles.
-
Computer-Aided Design (CAD): Determining distances between lines is crucial for ensuring precision and accuracy in design models.
-
Computer Graphics: Rendering realistic scenes often involves calculating distances between objects to determine visibility and shadows.
-
Physics Simulations: Many physics simulations, particularly those involving rigid bodies, require calculating distances between lines or line segments to model interactions.
Conclusion
Calculating the distance between two lines in 3D space is a multifaceted problem with different approaches depending on the lines' relative positions. Understanding the underlying vector algebra and choosing the appropriate method ensures accurate and efficient calculations in various applications. While this article provides a detailed explanation of the methods, implementing robust and production-ready code requires careful consideration of edge cases and error handling. Using established libraries for vector operations is strongly recommended for real-world applications.
Latest Posts
Latest Posts
-
Is A Webcam An Input Or Output Device
Mar 17, 2025
-
Word For A Person Who Uses Big Words
Mar 17, 2025
-
What Is 375 As A Percentage
Mar 17, 2025
-
Which Is The Correct Order Of The Scientific Method
Mar 17, 2025
-
How Long Is A Thousand Days
Mar 17, 2025
Related Post
Thank you for visiting our website which covers about Distance Between 2 Lines In 3d . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.