Difference Between Cls And Self In Python
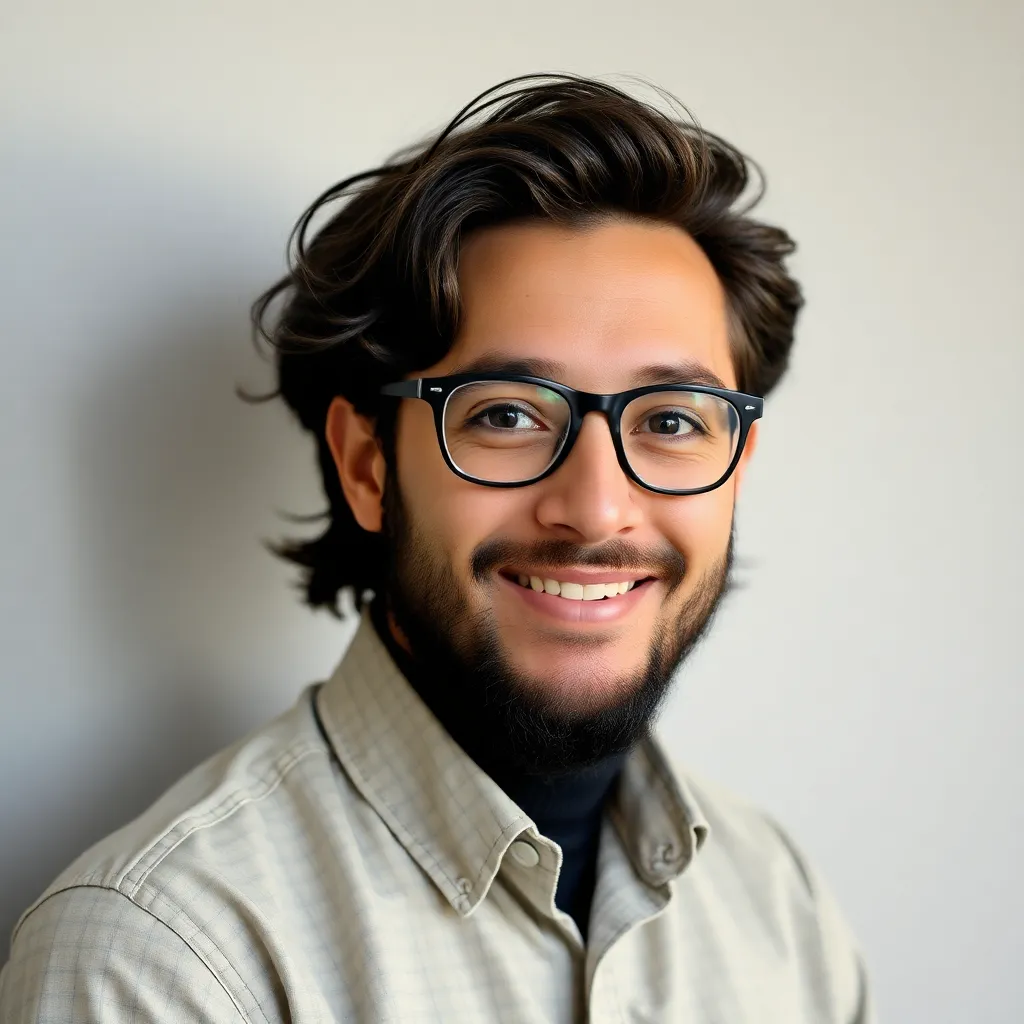
News Leon
Apr 05, 2025 · 6 min read
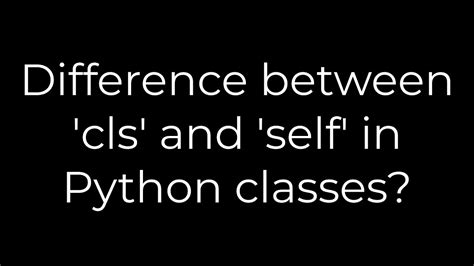
Table of Contents
Delving Deep into Python's cls
and self
: Unveiling the Mysteries of Class and Instance Methods
Python, a versatile and widely-used programming language, relies heavily on object-oriented programming (OOP) principles. A cornerstone of OOP is the concept of classes and methods, and within this framework, understanding the difference between cls
and self
is crucial for writing robust and efficient Python code. This comprehensive guide will dissect the nuances of these two parameters, highlighting their roles in class methods, static methods, and instance methods, and providing clear examples to solidify your understanding.
Understanding the Core Concepts: Classes, Objects, and Methods
Before diving into the specifics of cls
and self
, let's revisit fundamental OOP concepts. A class serves as a blueprint for creating objects. It defines attributes (data) and methods (functions) that objects of that class will possess. An object (also known as an instance) is a concrete realization of a class. Think of a class as a cookie cutter and an object as a cookie created using that cutter.
Methods are functions defined within a class. They operate on the data within the object (or the class itself, depending on the type of method). There are three primary types of methods in Python:
- Instance Methods: These are the most common type of method. They operate on an instance of a class and always require the
self
parameter. - Class Methods: These methods operate on the class itself, rather than a specific instance. They use the
cls
parameter. - Static Methods: These methods are not directly related to either the class or its instances. They are essentially regular functions that happen to reside within a class for organizational purposes.
The Role of self
in Instance Methods
The self
parameter in an instance method refers to the instance of the class upon which the method is called. It's a convention, not a keyword, and you could technically use any valid variable name, but self
is the widely accepted and preferred choice for readability and maintainability. self
gives the method access to the instance's attributes and other instance methods.
Let's illustrate with an example:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} says Woof!")
my_dog = Dog("Buddy", "Golden Retriever")
my_dog.bark() # Output: Buddy says Woof!
In this example, bark()
is an instance method. The self
parameter allows the bark()
method to access the name
attribute of the my_dog
instance. Without self
, the method wouldn't know which dog's name to use.
Key Characteristics of self
:
- Implicitly Passed: You don't explicitly pass
self
when calling an instance method; Python handles it automatically. - Reference to Instance:
self
refers to the specific object (instance) the method is called upon. - Access to Attributes and Methods:
self
allows access to the object's attributes and other methods within the class.
The Role of cls
in Class Methods
The cls
parameter in a class method refers to the class itself. Class methods are defined using the @classmethod
decorator. They can access and modify class-level attributes (attributes defined directly within the class, not within an instance). They can also create instances of the class.
Consider this example:
class Circle:
pi = 3.14159
def __init__(self, radius):
self.radius = radius
@classmethod
def from_diameter(cls, diameter):
radius = diameter / 2
return cls(radius)
my_circle = Circle.from_diameter(10)
print(my_circle.radius) # Output: 5.0
Here, from_diameter
is a class method. cls
refers to the Circle
class. The method uses cls
to create a new Circle
instance using the provided diameter. Note that cls
is used to instantiate the class, creating a new object.
Key Characteristics of cls
:
- Explicitly Passed: The
@classmethod
decorator ensurescls
is passed implicitly when the class method is called. - Reference to Class:
cls
points to the class itself, not an instance. - Access to Class Attributes:
cls
allows access to and modification of class-level attributes. - Class Creation:
cls
is commonly used to create instances of the class from alternative parameters.
Static Methods: The Independent Functions
Static methods are defined using the @staticmethod
decorator. They don't have access to either self
or cls
. They are essentially regular functions that are included within a class for organizational purposes; they are not bound to the class or its instances. They primarily enhance code structure and readability.
class MathUtils:
@staticmethod
def is_even(number):
return number % 2 == 0
print(MathUtils.is_even(4)) # Output: True
In this example, is_even
is a static method. It doesn't need to access any class or instance attributes, hence it doesn't use self
or cls
.
Key Characteristics of Static Methods:
- No
self
orcls
: They don't have access to instance or class attributes. - Organizational Purposes: They are mainly used for grouping logically related functions within a class.
- No Implicit Binding: They are not bound to the class or its instances.
self
vs. cls
: A Detailed Comparison
Feature | self (Instance Method) |
cls (Class Method) |
Static Method |
---|---|---|---|
Parameter Type | Instance | Class | Neither |
Access | Instance attributes | Class attributes | Neither |
Decorator | None | @classmethod |
@staticmethod |
Purpose | Operate on instance | Operate on class, factory methods | Utility functions within a class |
Call Syntax | object.method() |
Class.method() |
Class.method() |
Advanced Usage Scenarios and Best Practices
The choice between instance, class, and static methods depends heavily on the context and desired functionality. Understanding these nuances allows for well-structured and maintainable code.
When to use instance methods:
- When the method needs to access or modify instance-specific attributes.
- When the method's logic is directly tied to the state of a particular object.
When to use class methods:
- When the method needs to access or modify class-level attributes.
- When creating factory methods (alternative constructors for creating instances).
- When the method's logic is related to the class itself, not a specific instance.
When to use static methods:
- When you have utility functions closely related to the class's functionality but don't need access to instance or class data.
- To improve code organization and readability.
Error Handling and Debugging Tips
Incorrect usage of self
or cls
can lead to AttributeError
exceptions if you try to access attributes that don't exist or are not accessible within the scope of the method. Thorough testing and careful consideration of method types are essential for preventing these errors.
Conclusion: Mastering cls
and self
for Effective OOP
Understanding the differences between self
and cls
is pivotal for writing effective and maintainable object-oriented Python code. By grasping the roles of instance, class, and static methods, you gain the tools to design elegant and scalable applications. Remember to choose the appropriate method type based on its intended functionality and access requirements, ensuring your code reflects best practices and remains easily understandable and debuggable. The consistent and correct use of self
and cls
directly contributes to the robustness and clarity of your Python programs, making them easier to maintain, extend, and collaborate on.
Latest Posts
Latest Posts
-
Bangalore Is Capital Of Which State
Apr 06, 2025
-
Cytoplasm Divides Immediately After This Period
Apr 06, 2025
-
What Is The Percent Composition Of Co
Apr 06, 2025
-
In The Visible Spectrum Which Color Has The Longest Wavelength
Apr 06, 2025
-
Which Of The Following Statements About Stigma Is True
Apr 06, 2025
Related Post
Thank you for visiting our website which covers about Difference Between Cls And Self In Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.