Count Of A Character In A String
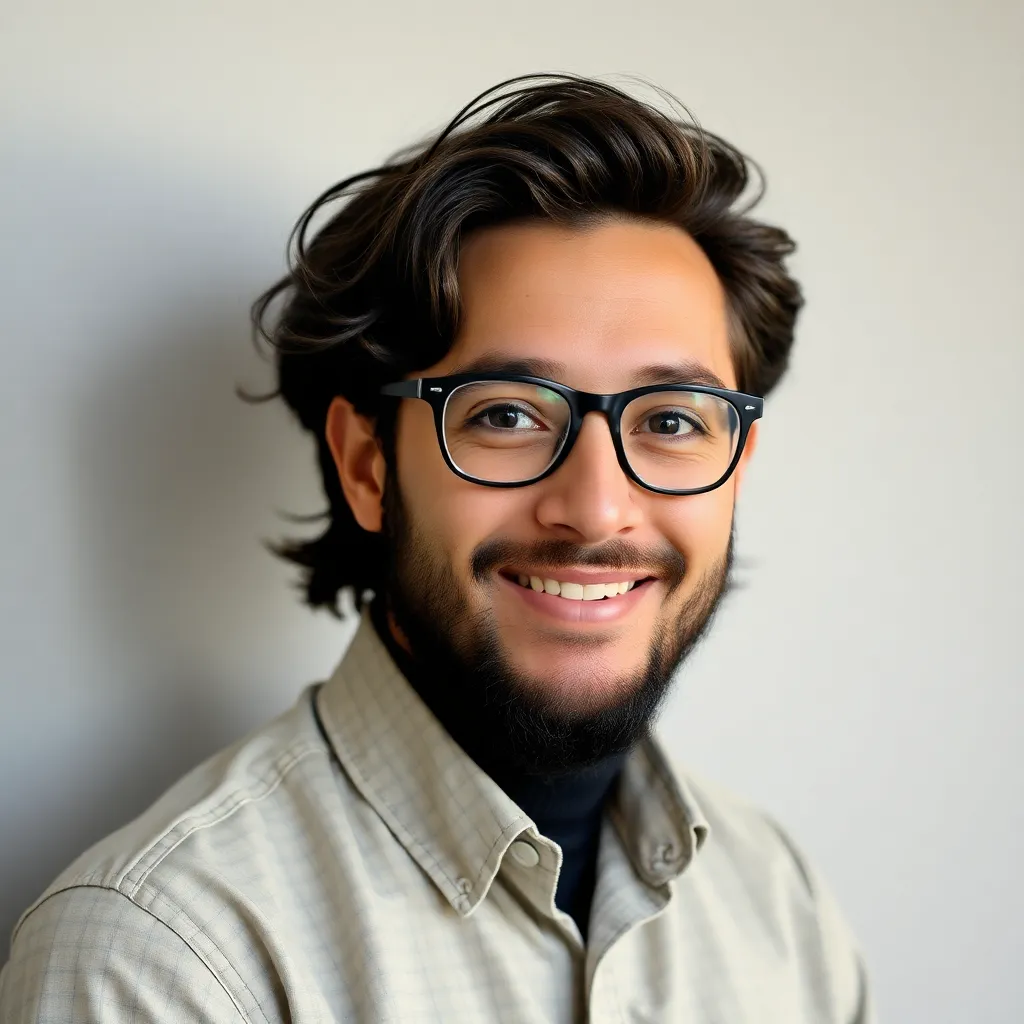
News Leon
Apr 21, 2025 · 6 min read

Table of Contents
Counting Characters in a String: A Comprehensive Guide
Counting the occurrences of specific characters within a string is a fundamental task in various programming applications, from data analysis and text processing to algorithm design and security. This comprehensive guide will delve into the intricacies of character counting, exploring different approaches, their efficiency, and practical applications. We'll cover methods suitable for diverse programming languages and scenarios, equipping you with the knowledge to tackle character counting challenges effectively.
Understanding the Problem: Why Count Characters?
Before diving into the solutions, let's understand why counting characters is so important. This seemingly simple task underpins many sophisticated operations:
-
Data Analysis: Analyzing text data often requires understanding the frequency of specific characters or character sets. This is crucial in natural language processing (NLP), sentiment analysis, and market research. For example, counting the frequency of punctuation marks can reveal aspects of writing style or emotional tone.
-
Text Processing: Many text manipulation tasks rely on character counts. Imagine needing to truncate a string to a specific length, or replacing a character with another based on its occurrence. Accurate character counting forms the basis of these operations.
-
Algorithm Design: Character counting algorithms form the building blocks of more complex algorithms. For instance, pattern matching algorithms often rely on counting the occurrences of specific patterns within a larger string.
-
Security: Character counting can play a role in security applications. Password strength checkers, for instance, might analyze the character composition of a password to assess its strength. The prevalence of certain characters might indicate vulnerabilities.
-
Data Validation: In input validation, character counting can ensure that data conforms to specific rules. For instance, a form might require a minimum number of characters in a password field.
Basic Approaches to Character Counting
Let's explore several ways to count characters in a string, ranging from simple iterative approaches to more sophisticated techniques using built-in functions and data structures.
1. Iterative Approach (Looping):
This is the most fundamental approach, using a loop to iterate through each character of the string. We can use a dictionary (or hash map) to store character counts:
def count_characters_iterative(text):
"""Counts character occurrences using an iterative approach."""
char_counts = {}
for char in text:
char_counts[char] = char_counts.get(char, 0) + 1
return char_counts
text = "Hello, world!"
counts = count_characters_iterative(text)
print(counts) # Output: {'H': 1, 'e': 1, 'l': 3, 'o': 2, ',': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1, '!': 1}
This Python code iterates through each character. If a character is already in char_counts
, its count is incremented; otherwise, it's added with a count of 1.
2. Using Counter
(Python):
Python's collections.Counter
class provides a highly efficient way to count character occurrences:
from collections import Counter
def count_characters_counter(text):
"""Counts character occurrences using Python's Counter."""
return Counter(text)
text = "Hello, world!"
counts = count_characters_counter(text)
print(counts) # Output: Counter({'l': 3, 'o': 2, 'H': 1, 'e': 1, ',': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1, '!': 1})
Counter
automatically handles the counting process, making the code significantly more concise and often faster than manual iteration.
3. Using Regular Expressions (Regex):
Regular expressions offer a powerful way to count character occurrences, especially when dealing with complex patterns or character sets.
import re
def count_characters_regex(text, pattern):
"""Counts occurrences of a pattern using regular expressions."""
matches = re.findall(pattern, text)
return len(matches)
text = "Hello, world!"
vowel_count = count_characters_regex(text, r"[aeiouAEIOU]") #Counts vowels
print(f"Number of vowels: {vowel_count}") #Output: Number of vowels: 3
consonant_count = count_characters_regex(text, r"[bcdfghjklmnpqrstvwxyzBCDFGHJKLMNPQRSTVWXYZ]") #Counts consonants
print(f"Number of consonants: {consonant_count}") #Output: Number of consonants: 7
This uses regular expressions to count vowels and consonants. The power of regex lies in its ability to handle complex patterns that go beyond simple character matching.
Advanced Techniques and Considerations
Let's explore more advanced scenarios and optimizations for character counting:
1. Case-Insensitive Counting:
Often, you might want to count characters regardless of their case (e.g., treating 'A' and 'a' as the same). This can be easily achieved by converting the string to lowercase (or uppercase) before counting:
def count_characters_case_insensitive(text):
"""Counts characters ignoring case."""
text = text.lower() #or text.upper()
return Counter(text)
text = "Hello, World!"
counts = count_characters_case_insensitive(text)
print(counts) # Output: Counter({'l': 3, 'o': 2, 'h': 1, 'e': 1, ',': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1, '!': 1})
2. Handling Unicode Characters:
Character counting becomes slightly more complex when dealing with Unicode characters, as some characters might require multiple bytes to represent. Most modern programming languages handle this automatically, but it's crucial to be aware of potential differences in character encoding.
3. Efficiency for Large Strings:
For extremely large strings, the efficiency of your counting method becomes critical. The iterative approach might become slow. Consider using optimized data structures or parallel processing techniques for substantial performance improvements, especially when dealing with massive datasets.
4. Counting Specific Character Sets:
Sometimes, you need to count occurrences of characters within a specific set (e.g., digits, punctuation, whitespace). Regular expressions provide a powerful and flexible way to achieve this.
import re
def count_specific_characters(text, char_set):
"""Counts characters belonging to a specified set."""
matches = re.findall(f"[{char_set}]", text)
return len(matches)
text = "Hello, world! 123"
digit_count = count_specific_characters(text, r"\d") # Counts digits
punctuation_count = count_specific_characters(text, r"\p{P}") # Counts punctuation (Unicode aware)
print(f"Digit count: {digit_count}") # Output: Digit count: 3
print(f"Punctuation count: {punctuation_count}") # Output: Punctuation count: 2
Practical Applications and Examples
Let's explore how character counting is used in real-world scenarios:
1. Natural Language Processing (NLP):
NLP heavily relies on character counting for tasks like:
- N-gram analysis: Counting the frequency of character sequences (n-grams) is essential for language modeling and text prediction.
- Stop word removal: Identifying and removing common words (stop words) often involves analyzing character frequencies.
- Tokenization: Breaking text into individual units (tokens) often requires considering character boundaries.
2. Data Cleaning and Preprocessing:
Character counting is crucial for data cleaning and preprocessing tasks, such as:
- Removing extra whitespace: Counting and removing extra spaces or tabs improves data consistency.
- Handling special characters: Identifying and handling special characters (like accented characters or control characters) is crucial for data normalization.
3. Security Applications:
In security, character counting assists in:
- Password strength analysis: Evaluating password complexity based on the variety and frequency of characters.
- Input validation: Enforcing minimum character requirements for fields in forms or databases.
- Detecting malicious code: Analyzing character frequencies to identify patterns indicative of malicious code injection.
4. Algorithm Design and Optimization:
Character counting is a fundamental building block in various algorithms, such as:
- Pattern matching: Efficiently finding patterns within large strings often involves character counting optimizations.
- Data compression: Techniques like Huffman coding rely on character frequency analysis for optimal compression.
- Data structures: Efficient data structures, like tries and suffix trees, utilize character counting for efficient searching and indexing.
Conclusion: Mastering Character Counting
Character counting, while seemingly simple, is a powerful technique with numerous applications across diverse domains. Understanding the various methods, their efficiency trade-offs, and the practical contexts in which they are used is crucial for any programmer or data scientist. This guide has provided a comprehensive overview, equipping you with the knowledge and tools to effectively tackle character counting challenges, paving the way for more advanced text processing and data analysis tasks. Remember to choose the method that best suits your specific needs, considering factors like string size, character set complexity, and performance requirements. The ability to efficiently and accurately count characters is a fundamental skill in the world of programming and data science.
Latest Posts
Latest Posts
-
Find The Measure Of Angle X And Angle Y
Apr 21, 2025
-
How To Find A Unit Vector Perpendicular To Two Vectors
Apr 21, 2025
-
Amino Acids Can Be Classified By The
Apr 21, 2025
-
Common Multiples Of 7 And 4
Apr 21, 2025
-
Find The Power Dissipated In The 6 Ohm Resistor
Apr 21, 2025
Related Post
Thank you for visiting our website which covers about Count Of A Character In A String . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.