Check If Letter Is Uppercase Python
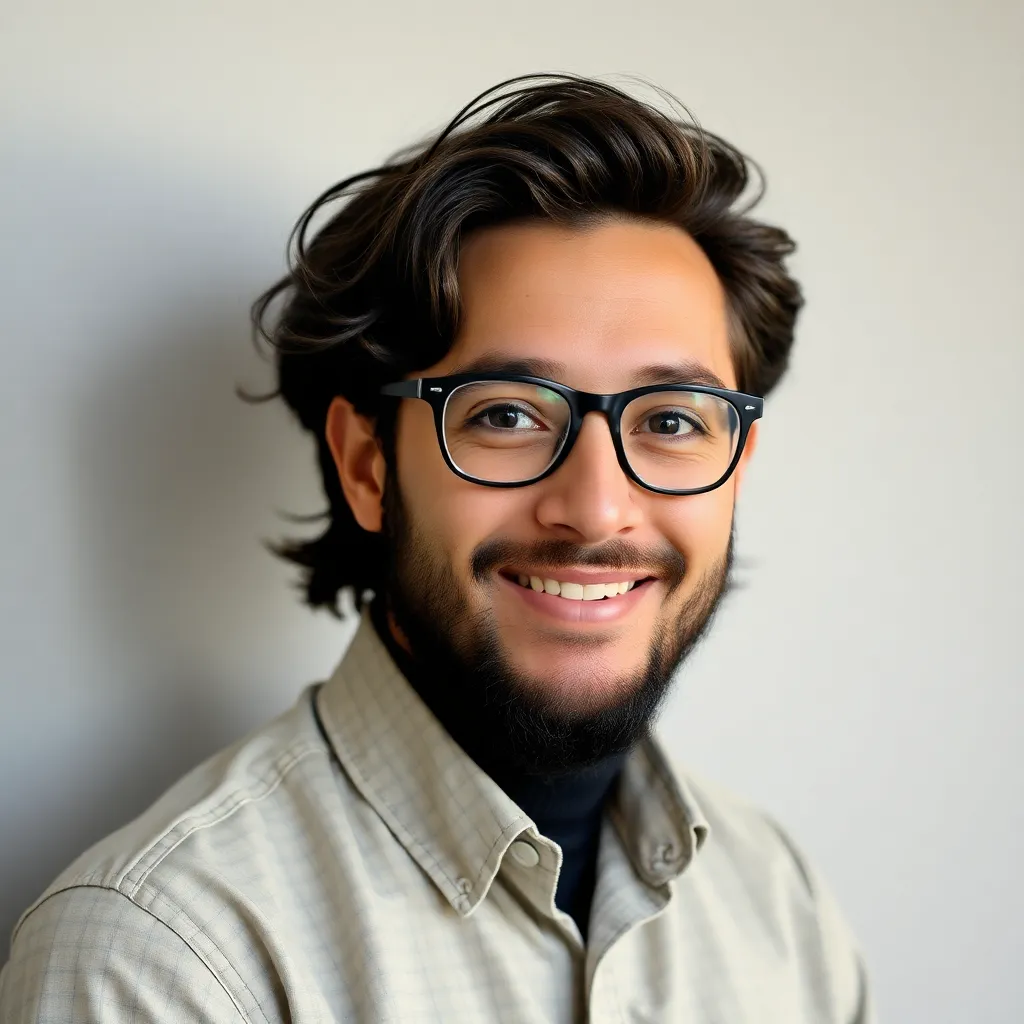
News Leon
Apr 17, 2025 · 6 min read

Table of Contents
Checking if a Letter is Uppercase in Python: A Comprehensive Guide
Determining whether a character is uppercase is a fundamental task in many Python programming scenarios, from data validation and text processing to more complex algorithms. This comprehensive guide explores various methods to achieve this, ranging from simple built-in functions to more nuanced approaches for handling diverse character sets and edge cases. We will delve into the efficiency and applicability of each method, equipping you with the knowledge to choose the optimal solution for your specific needs.
Understanding the Problem: Case Sensitivity in Python
Python, like many programming languages, is case-sensitive. This means that "A" is considered different from "a". This distinction is crucial when working with strings and characters, as operations like comparisons, searching, and sorting are affected by case. The need to check for uppercase characters arises frequently in tasks such as:
- Data Validation: Ensuring user input conforms to specified case requirements (e.g., a username must begin with an uppercase letter).
- Text Processing: Converting text to uppercase or lowercase, identifying capitalized words or proper nouns, or performing case-insensitive searches.
- Algorithm Design: Certain algorithms might rely on differentiating between uppercase and lowercase characters for specific logic or operations.
- Security: Verifying password complexity requirements, often including uppercase letters.
Method 1: Using the isupper()
Method
The most straightforward and Pythonic way to check if a letter is uppercase is using the built-in string method isupper()
. This method is highly efficient and directly addresses the problem.
character = 'A'
is_uppercase = character.isupper()
print(f"'{character}' is uppercase: {is_uppercase}") # Output: 'A' is uppercase: True
character = 'a'
is_uppercase = character.isupper()
print(f"'{character}' is uppercase: {is_uppercase}") # Output: 'a' is uppercase: False
character = '1'
is_uppercase = character.isupper()
print(f"'{character}' is uppercase: {is_uppercase}") # Output: '1' is uppercase: False
Advantages:
- Simplicity: Easy to understand and use.
- Efficiency: Highly optimized for performance.
- Readability: Makes code cleaner and more maintainable.
Disadvantages:
- Only for single characters: Doesn't work directly on strings with multiple characters. You'd need to iterate through the string.
- ASCII limitations: While generally effective, it might have limitations with extended Unicode characters.
Method 2: Using ASCII Values with ord()
This method leverages the ASCII (American Standard Code for Information Interchange) values of characters. Uppercase letters in ASCII have values between 65 ('A') and 90 ('Z'). The ord()
function returns the ASCII value of a character.
character = 'A'
ascii_value = ord(character)
is_uppercase = 65 <= ascii_value <= 90
print(f"'{character}' is uppercase: {is_uppercase}") # Output: 'A' is uppercase: True
character = 'a'
ascii_value = ord(character)
is_uppercase = 65 <= ascii_value <= 90
print(f"'{character}' is uppercase: {is_uppercase}") # Output: 'a' is uppercase: False
Advantages:
- Understanding ASCII: Provides a deeper understanding of character encoding.
- Flexibility (with modifications): Can be adapted to handle other character ranges.
Disadvantages:
- Less readable: Can be less intuitive than
isupper()
. - ASCII limitations: Primarily works with basic ASCII characters; may not accurately handle Unicode characters outside the basic ASCII range.
- Less efficient: Generally less efficient than the built-in
isupper()
method.
Method 3: Handling Unicode Characters with unicodedata
For robust handling of Unicode characters, the unicodedata
module provides more comprehensive case handling. The category()
function from this module can determine the character's category, allowing identification of uppercase letters even beyond the basic ASCII range.
import unicodedata
character = 'A'
category = unicodedata.category(character)
is_uppercase = category == 'Lu' # 'Lu' signifies uppercase letters
print(f"'{character}' is uppercase: {is_uppercase}") # Output: 'A' is uppercase: True
character = 'Ä' # German uppercase A umlaut
category = unicodedata.category(character)
is_uppercase = category == 'Lu'
print(f"'{character}' is uppercase: {is_uppercase}") # Output: 'Ä' is uppercase: True
character = 'α' # Greek lowercase alpha
category = unicodedata.category(character)
is_uppercase = category == 'Lu'
print(f"'{character}' is uppercase: {is_uppercase}") # Output: 'α' is uppercase: False
Advantages:
- Unicode support: Handles a wide range of Unicode characters accurately.
- Comprehensive: Provides a more detailed character classification.
Disadvantages:
- More complex: Requires importing a module and understanding character categories.
- Slightly less efficient: Generally slower than
isupper()
for basic ASCII characters.
Method 4: Regular Expressions (for more complex patterns)
For more sophisticated pattern matching involving uppercase letters within a larger string context, regular expressions (using the re
module) offer powerful capabilities.
import re
text = "Hello World! This is a Test."
uppercase_letters = re.findall(r'[A-Z]', text)
print(f"Uppercase letters in the string: {uppercase_letters}") # Output: Uppercase letters in the string: ['H', 'W', 'T']
#Check if a string starts with an uppercase letter.
starts_with_uppercase = bool(re.match(r'^[A-Z]', text))
print(f"String starts with uppercase: {starts_with_uppercase}") #Output: String starts with uppercase: True
Advantages:
- Pattern matching: Ideal for finding uppercase letters within complex string structures.
- Flexibility: Allows for highly customized pattern definitions.
Disadvantages:
- Overhead: Regular expressions can be less efficient than simpler methods for single-character checks.
- Complexity: Requires understanding of regular expression syntax.
Choosing the Right Method
The optimal method for checking if a letter is uppercase in Python depends on the specific context:
- For simple checks on individual ASCII characters,
isupper()
is the most efficient and readable choice. - If you need to understand the ASCII value or work with character ranges, the
ord()
method offers insight but is less efficient and less readable. - For robust handling of Unicode characters, the
unicodedata
module provides the necessary functionality. - When dealing with more complex patterns within strings, regular expressions provide the flexibility to match various uppercase letter occurrences.
Beyond Single Characters: Processing Strings
All the methods discussed above primarily focus on checking individual characters. When working with strings containing multiple characters, you'll typically need to iterate through the string and apply the chosen method to each character individually. Here's an example using isupper()
:
def count_uppercase(text):
count = 0
for char in text:
if char.isupper():
count += 1
return count
text = "Hello World!"
uppercase_count = count_uppercase(text)
print(f"Number of uppercase letters: {uppercase_count}") # Output: Number of uppercase letters: 2
This example demonstrates a simple way to count the number of uppercase letters in a string. You can adapt this approach to perform other operations, such as converting uppercase letters to lowercase, or identifying words that start with an uppercase letter.
Error Handling and Robustness
In real-world applications, you might encounter unexpected input. Always consider error handling to make your code more robust. For instance, if you're processing user input, you should handle potential exceptions (like TypeError
if the input isn't a string).
def is_uppercase_safe(input_char):
try:
return input_char.isupper()
except AttributeError:
return False #Handle non-string input gracefully.
except Exception as e:
print(f"An error occurred: {e}")
return False
print(is_uppercase_safe("A")) #True
print(is_uppercase_safe(123)) #False
Conclusion
Checking if a letter is uppercase in Python is a fundamental task with various solutions depending on your specific needs and the complexity of your data. The isupper()
method provides the simplest and most efficient approach for single characters, while the unicodedata
module handles Unicode characters effectively. Regular expressions offer flexibility for complex pattern matching, and understanding ASCII values offers a deeper insight into character representation. Remember to choose the method that best suits your situation and always consider error handling for robust code. This comprehensive guide provides you with a robust foundation for handling uppercase characters in your Python projects, from simple checks to advanced text processing tasks. By understanding the nuances of each method, you can write more efficient, readable, and maintainable Python code.
Latest Posts
Latest Posts
-
Which Solution Is The Most Acidic
Apr 19, 2025
-
Write The Prime Factorization Of 90
Apr 19, 2025
-
What Types Of Cells Would Have More Mitochondria
Apr 19, 2025
-
Domain Of 1 X 2 1
Apr 19, 2025
-
Are Combustion Reactions Exothermic Or Endothermic
Apr 19, 2025
Related Post
Thank you for visiting our website which covers about Check If Letter Is Uppercase Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.