A Loop Always Executes At Least Once
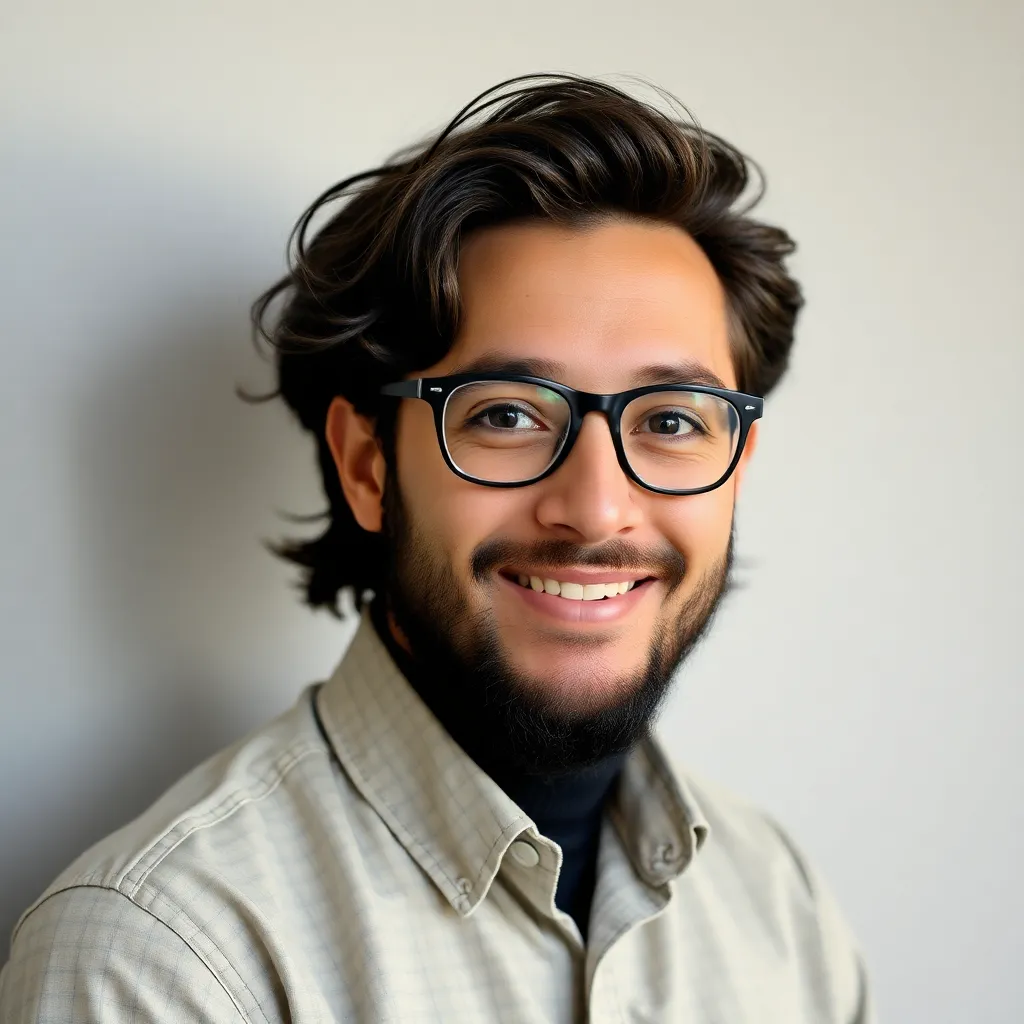
News Leon
Apr 14, 2025 · 5 min read
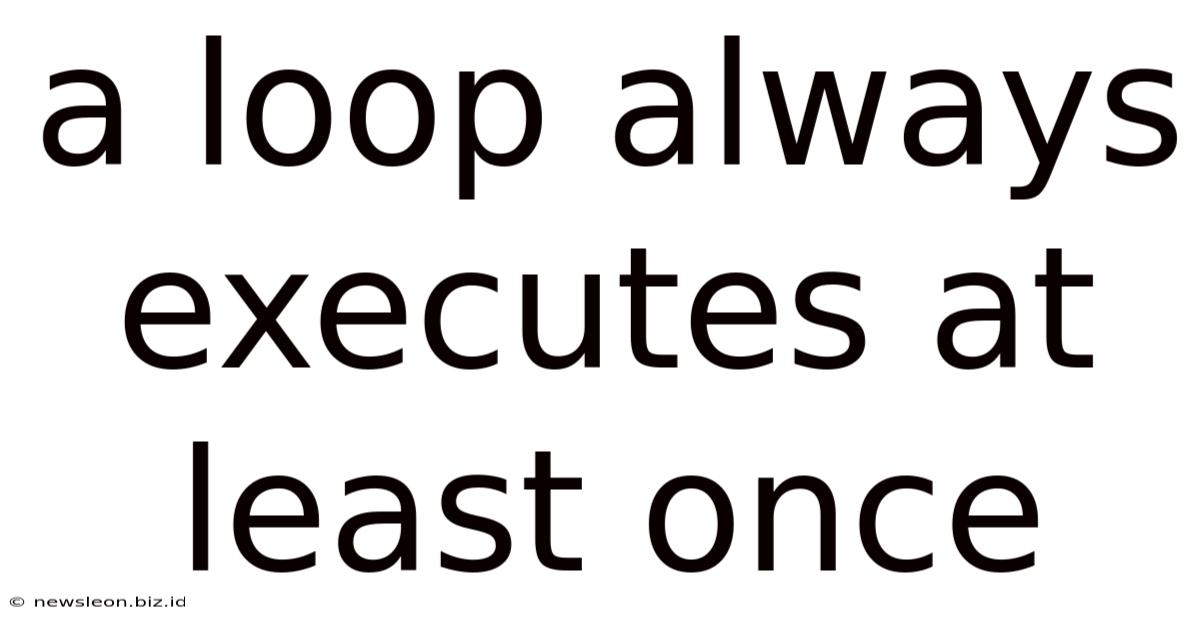
Table of Contents
A Loop Always Executes at Least Once: Exploring Do-While and Other Loop Structures
Loops are fundamental building blocks in programming, enabling the repetition of code blocks based on specified conditions. While many loops check their conditions before each iteration (like for
and while
loops), some, notably the do-while
loop, guarantee at least one execution. Understanding this distinction is crucial for writing efficient and error-free code. This article delves into the mechanics of loops that always execute at least once, focusing on the do-while
loop, its applications, and comparisons with other loop types. We'll also explore scenarios where this behavior is particularly advantageous and potential pitfalls to avoid.
The Do-While Loop: A Guarantee of Execution
The do-while
loop, unlike its while
counterpart, checks its condition after each iteration. This subtle difference fundamentally alters its behavior: the loop body will always execute at least once, regardless of the initial state of the condition. The syntax typically follows this structure:
do {
// Code to be executed repeatedly
} while (condition);
The code within the do
block is executed first. Only then is the condition
evaluated. If the condition is true, the loop continues; otherwise, it terminates. This ensures that the loop's body always runs through at least one cycle.
A Simple Example:
Let's illustrate with a C++ example that prompts the user for input until they enter a valid number:
#include
int main() {
int number;
do {
std::cout << "Enter a positive number: ";
std::cin >> number;
} while (number <= 0);
std::cout << "You entered: " << number << std::endl;
return 0;
}
In this case, the user is always prompted to enter a number at least once, even if they initially enter a non-positive value. The loop continues until a positive number is provided.
Comparing Do-While with While and For Loops
To fully appreciate the do-while
loop's unique characteristic, let's compare it with while
and for
loops:
While Loop:
The while
loop checks its condition before each iteration. If the condition is initially false, the loop body will never execute:
int i = 0;
while (i > 0) {
std::cout << i << std::endl;
i++;
} //This loop will never execute
This behavior contrasts sharply with the do-while
loop, which always executes at least once.
For Loop:
The for
loop is typically used for iterating a specific number of times or over a collection. Its condition is also checked before each iteration. While you can manipulate the loop counter to simulate a do-while
behavior, it's generally less readable and can be more prone to errors:
for (int i = 0; i < 1; i++) {
//Code will always execute once
i--; //Simulate do while
}
This approach is less intuitive and less maintainable compared to using a do-while
loop directly when the 'at least once' execution is the primary requirement.
When to Use a Do-While Loop
The do-while
loop's guaranteed execution makes it particularly suitable for specific scenarios:
-
Menu-Driven Programs: In interactive programs with menus, you want the menu to be displayed at least once, allowing the user to make a choice. A
do-while
loop perfectly fits this situation. The loop continues until the user chooses to exit. -
Input Validation: As shown in the earlier example,
do-while
loops are ideal for prompting the user for input until valid data is provided. The loop ensures that the user is always prompted at least once, giving them a chance to enter their input. -
Game Loops: In simple game loops, you often want to perform at least one game cycle, even if the game's condition for continuing (e.g., game over) is initially met.
-
Processing Files or Data Streams: When processing data from a file or a network stream, you may want to attempt to read at least one piece of data, even if the stream is empty. A
do-while
loop ensures that at least one read operation is performed. -
Interactive Simulations: When creating a simulation that requires at least one iteration regardless of initial conditions, a
do-while
loop provides a clean and concise approach.
Potential Pitfalls and Best Practices
While do-while
loops offer valuable functionality, it's important to be aware of potential pitfalls:
-
Infinite Loops: Ensure that the loop's condition will eventually become false. An incorrectly structured
do-while
loop, just like any other loop, can easily lead to an infinite loop if the condition never evaluates tofalse
. Always carefully review the conditions to prevent this. -
Readability: While
do-while
loops have their advantages, overuse can sometimes reduce code readability. If awhile
loop with an initial execution step achieves the same outcome more clearly, it's often preferable for better code maintainability. -
Debugging: Infinite loops resulting from
do-while
loops can be challenging to debug. Employ debugging tools and techniques, including print statements or debuggers, to track the loop's execution and identify the source of any infinite loop problems.
Beyond Do-While: Achieving "At Least Once" Execution in Other Ways
Although the do-while
loop is the most direct approach, you can achieve at least one execution using other loop structures with careful design:
Using a while
loop with a flag:
bool firstIteration = true;
while (firstIteration || condition) {
// Code to be executed
firstIteration = false;
}
This approach uses a boolean flag to control the first iteration, ensuring that the loop body executes at least once.
Using a for
loop with a modified condition:
for (int i = 0; i >=0 ; i++) {
if (i == 0) {
// Code to execute at least once
if(condition)
i = 1;
}
if (condition) {
// subsequent iterations
} else {
break; //Exit if condition is false
}
}
However, these alternatives often lead to more complex code compared to the elegance and simplicity of the do-while
loop.
Conclusion
The do-while
loop, with its guarantee of at least one execution, provides a powerful tool for specific programming tasks. Understanding its mechanics, comparing it to other loop types, and recognizing its appropriate applications are crucial for writing efficient and maintainable code. While offering significant advantages, careful consideration of potential pitfalls, such as infinite loops and readability, is essential for ensuring robust and well-structured programs. When the requirement is that a block of code needs to be executed at least once, the do-while
loop provides the most elegant and direct solution. Remember to always choose the loop structure that best suits the specific needs of your program, prioritizing clarity and maintainability alongside functionality.
Latest Posts
Related Post
Thank you for visiting our website which covers about A Loop Always Executes At Least Once . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.